AI Features
Overview
QuickBloxUIKit comes with a range of AI features that enhance the capabilities of your chat application. These AI features leverage cutting-edge technologies to assist users, translate messages, summarize content, and more.
Feature | Group Dialog | Private Dialog |
---|---|---|
Assist Answer | ✓ | ✓ |
Translate | ✓ | ✓ |
Rephrase | ✓ | ✓ |
Assist Answer
The AI Assist Answer feature in QuickBloxUIKit allows you to generate answers in a chat based on the chat history using the QBAIAnswerAssistant Swift package. This feature leverages the OpenAI API key or proxy server to generate responses more securely and efficiently.
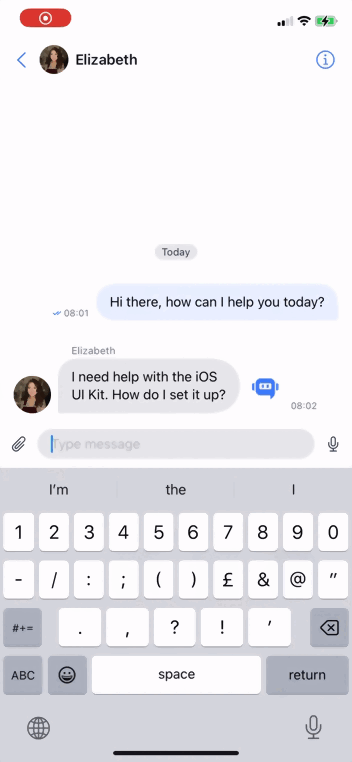
How to use
To use the AI Assist Answer feature in your QuickBloxUIKit project, follow these steps:
- Enable the AI Assist Answer feature:
QuickBloxUIKit.feature.ai.answerAssist.enable = true
If enabled, a button will appear next to each incoming message in the chat interface.
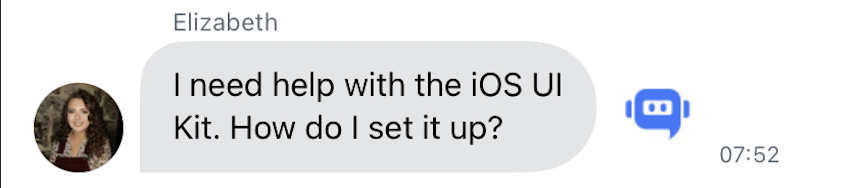
When the button is clicked, the Assist Answer feature will be launched, and a response will be generated based on the chat history.
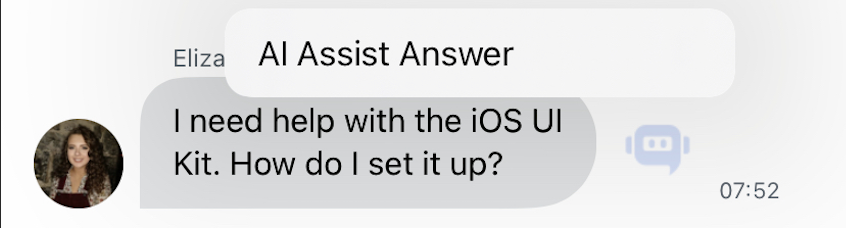
-
Set up the AI settings by providing either the OpenAI API key:
QuickBloxUIKit.feature.ai.answerAssist.apiKey = "YOUR_OPENAI_API_KEY"
Or set up with a proxy server:
QuickBloxUIKit.feature.ai.answerAssist.serverPath = "https://your-proxy-server-url"
We recommend using a proxy server like the QuickBlox AI Assistant Proxy Server offers significant benefits in terms of security and functionality:
- When making direct requests to the OpenAI API from the client-side, sensitive information like API keys may be exposed. By using a proxy server, the API keys are securely stored on the server-side, reducing the risk of unauthorized access or potential breaches.
- The proxy server can implement access control mechanisms, ensuring that only authenticated and authorized users with valid QuickBlox user tokens can access the OpenAI API. This adds an extra layer of security to the communication.
-
A developer using the AI Answer Assist library has the ability use to Default AIAnswerAssistSettings.
public class AIAnswerAssistSettings { /// Determines if assist answer functionality is enabled. public var enable: Bool = true /// The OpenAI API key for direct API requests (if not using a proxy server). public var apiKey: String = "" /// The URL path of the proxy server for more secure communication (if not using the API key directly). /// [QuickBlox AI Assistant Proxy Server](https://github.com/QuickBlox/qb-ai-assistant-proxy-server). public var serverPath: String = "" /// Represents the available API versions for OpenAI. public var apiVersion: QBAIAnswerAssistant.APIVersion = .v1 /// Optional organization information for OpenAI requests. public var organization: String? = nil /// Represents the available GPT models for OpenAI. public var model: QBAIAnswerAssistant.Model = .gpt3_5_turbo /// The temperature setting for generating responses (higher values make output more random). public var temperature: Float = 0.5 /// The maximum number of tokens to generate in the request. public var maxRequestTokens: Int = 3000 /// The maximum number of tokens to generate in the response. public var maxResponseTokens: Int? = nil }
-
A developer using the AI Answer Assist library has the ability to setup custom settings. This is an example of creating custom tones and installing them in QuickBlox iOS UI Kit from the custom application.
import QBAIAnswerAssistant // Setup custom settings for QBAIAnswerAssistant. QuickBloxUIKit.feature.ai.answerAssist.organization = "CustomDev" QuickBloxUIKit.feature.ai.answerAssist.model = .gpt4 QuickBloxUIKit.feature.ai.answerAssist.temperature = 0.8 QuickBloxUIKit.feature.ai.answerAssist.maxRequestTokens = 3500
More examples of setting custom settings for the appearance of user interface elements from a user application can be found in our UIKitSample.
-
A developer using the AI Answer Assist library has the ability to customize the Appearance of UI Answer Assist elements to adapt the user interface to their needs.
// Default UI Settings for Answer Assist public struct AIAnswerAssistUISettings { public var title: String public init(_ theme: ThemeProtocol) { self.title = theme.string.answerAssistTitle } }
This is an example of setting custom settings for the appearance of UI elements from a custom application.
QuickBloxUIKit.feature.ai.ui.answerAssist.title = "Quick Answer"
More examples of setting custom settings for the appearance of user interface elements from a user application can be found in our UIKitSample.
-
A developer using the AI Answer Assist library has the ability to customize the Appearance of "Robot" element to adapt the user interface to their needs.
// Default UI Settings for Robot public struct AIRobotSettings { public var icon: Image public var foreground: Color public var size: CGSize = CGSize(width: 24.0, height: 24.0) public var hidden: Bool = false public init(_ theme: ThemeProtocol) { self.icon = theme.image.robot self.foreground = theme.color.mainElements } }
This is an example of setting custom settings for the appearance of UI "Robot" element from a custom application.
QuickBloxUIKit.feature.ai.ui.robot.foreground = .green QuickBloxUIKit.feature.ai.ui.robot.icon = Image("CustomRobotIcon")
More examples of setting custom settings for the appearance of user interface elements from a user application can be found in our UIKitSample.
Translate
The AI Translate feature in QuickBloxUIKit empowers you to seamlessly integrate AI translation capabilities into your chat-based application using the QBAITranslate Swift package. This feature leverages the OpenAI API key or proxy server to generate responses more securely and efficiently.
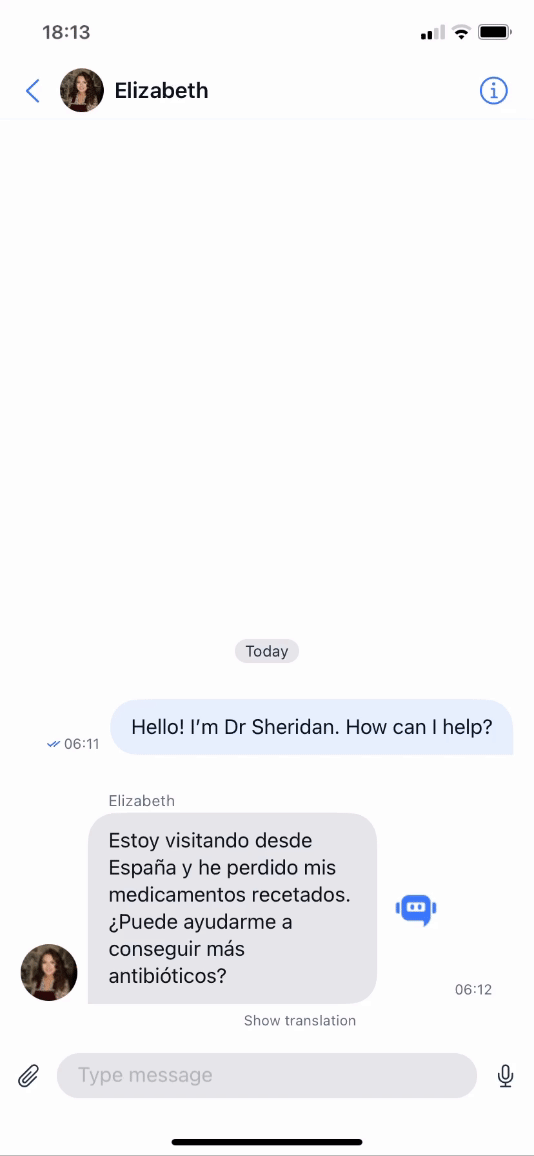
How to Use
To use the AI Translate feature in your QuickBloxUIKit project, follow these steps:
- Enable the AI Translate feature:
QuickBloxUIKit.feature.ai.translate.enable = true
If this option is enabled, the "Show translation" button will be displayed at the bottom of every incoming message in the chat interface.
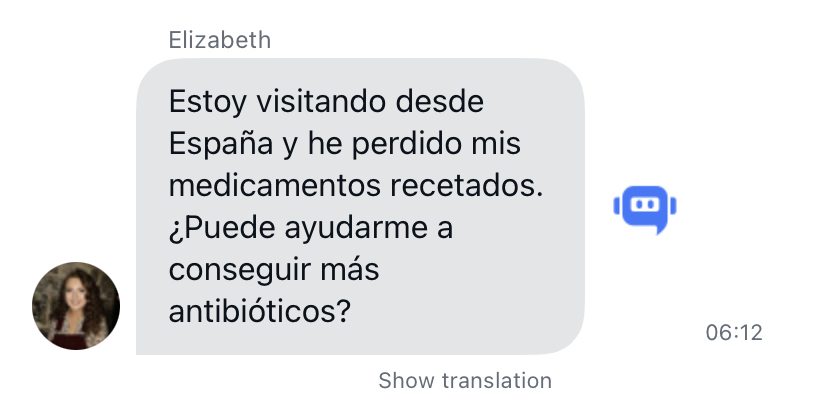
When you click on it, this message will be translated into the language set for translation, and the "Show original" button will be displayed, when clicked, the text of the message will immediately take the original version.
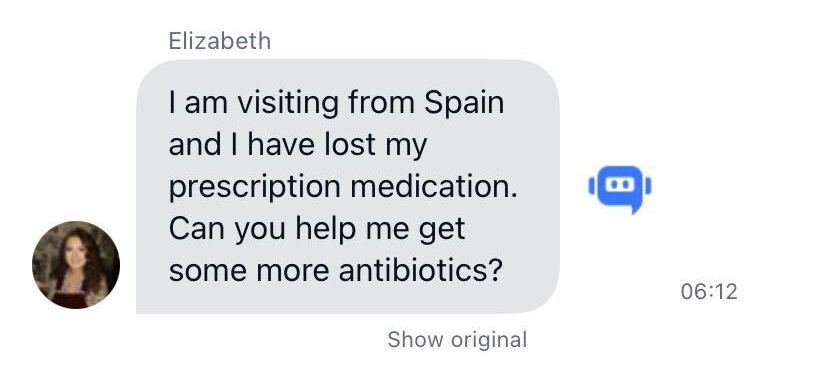
-
Set up the AI settings by providing either the OpenAI API key:
QuickBloxUIKit.feature.ai.translate.apiKey = "YOUR_OPENAI_API_KEY"
Or set up with a proxy server:
QuickBloxUIKit.feature.ai.translate.serverPath = "https://your-proxy-server-url"
We recommend using a proxy server like the QuickBlox AI Assistant Proxy Server offers significant benefits in terms of security and functionality:
- When making direct requests to the OpenAI API from the client-side, sensitive information like API keys may be exposed. By using a proxy server, the API keys are securely stored on the server-side, reducing the risk of unauthorized access or potential breaches.
- The proxy server can implement access control mechanisms, ensuring that only authenticated and authorized users with valid QuickBlox user tokens can access the OpenAI API. This adds an extra layer of security to the communication.
-
A developer using the AI Translate library has the ability use to Default QBAITranslate.Language and Default AITranslateSettings.
public class AITranslateSettings { /// The current `QBAITranslate.Language`. /// /// Default the same as system language or `.english` if `QBAITranslate.Language` is not support system language. public var language: QBAITranslate.Language /// Determines if assist answer functionality is enabled. public var enable: Bool = true /// The OpenAI API key for direct API requests (if not using a proxy server). public var apiKey: String = "" /// The URL path of the proxy server for more secure communication (if not using the API key directly). /// [QuickBlox AI Assistant Proxy Server](https://github.com/QuickBlox/qb-ai-assistant-proxy-server). public var serverPath: String = "" /// Represents the available API versions for OpenAI. public var apiVersion: QBAITranslate.APIVersion = .v1 /// Optional organization information for OpenAI requests. public var organization: String? = nil /// Represents the available GPT models for OpenAI. public var model: QBAITranslate.Model = .gpt3_5_turbo /// The temperature setting for generating responses (higher values make output more random). public var temperature: Float = 0.5 /// The maximum number of tokens to generate in the request. public var maxRequestTokens: Int = 3000 /// The maximum number of tokens to generate in the response. public var maxResponseTokens: Int? = nil }
-
A developer using the AI Translate library has the ability to setup custom translation language (by default used system language). Also a developer has the ability to setup custom settings. This is an example of creating custom tones and installing them in QuickBlox iOS UI Kit from the custom application.
import QBAITranslate // Set up the language for translation(by default used system language) QuickBloxUIKit.feature.ai.translate.language = .spanish // Setup custom settings for Translate. QuickBloxUIKit.feature.ai.translate.organization = "CustomDev" QuickBloxUIKit.feature.ai.translate.model = .gpt4 QuickBloxUIKit.feature.ai.translate.temperature = 0.8 QuickBloxUIKit.feature.ai.translate.maxRequestTokens = 3500
More examples of setting custom settings for the appearance of user interface elements from a user application can be found in our UIKitSample.
-
A developer using the AI Translate library has the ability to customize the Appearance of UI Translate elements to adapt the user interface to their needs.
// Default UI Settings for Translate public struct AITranslateUISettings { public var showOriginal: String public var showTranslation: String public var width: CGFloat public init(_ theme: ThemeProtocol) { self.showOriginal = theme.string.showOriginal self.showTranslation = theme.string.showTranslation self.width = max(self.showTranslation, self.showOriginal) .size(withAttributes: [.font: UIFont.preferredFont(forTextStyle: .caption2)]) .width + 24.0 } }
This is an example of setting custom settings for the appearance of UI elements from a custom application.
QuickBloxUIKit.feature.ai.ui.translate.showOriginal = "Original" QuickBloxUIKit.feature.ai.ui.translate.showTranslation = "Translation"
More examples of setting custom settings for the appearance of user interface elements from a user application can be found in our UIKitSample.
Incorporate AI Translate into your iOS chat application seamlessly using the QBAITranslate Swift package. Unlock the potential of AI-driven interactions and provide your users with real-time translation capabilities, enhancing user communication and experience.
For additional resources, explore the QuickBlox AI Assistant Proxy Server and the QBAITranslate Swift package repositories.
Resources:
Rephrase
The AI Rephrase feature in QuickBloxUIKit empowers you to seamlessly integrate AI Rephrase capabilities into your chat-based application using the QBAIRephrase Swift package. This feature leverages the OpenAI API key or proxy server to generate responses more securely and efficiently.
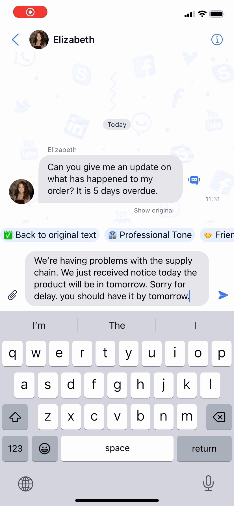
The AI Rephrase library allows you to rephrase a message using different tones so that the user can communicate effectively in different situations.
How to Use
To use the AI Rephrase feature in your QuickBloxUIKit project, follow these steps:
- Enable the AI Rephrase feature:
QuickBloxUIKit.feature.ai.rephrase.enable = true
If this option is enabled, the user interface provides a clear option or menu that allows the user to select the desired tone.
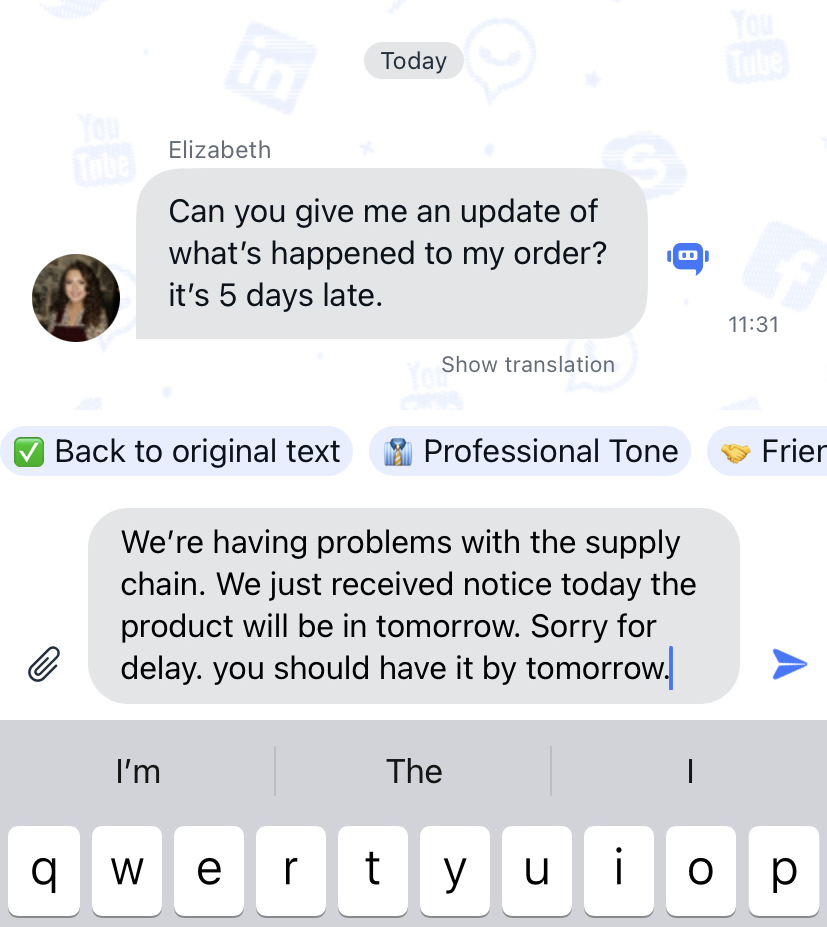
Once a tone is selected, the message is rephrased to reflect the characteristics of the selected tone.
A paraphrased message retains the main purpose of the original message.
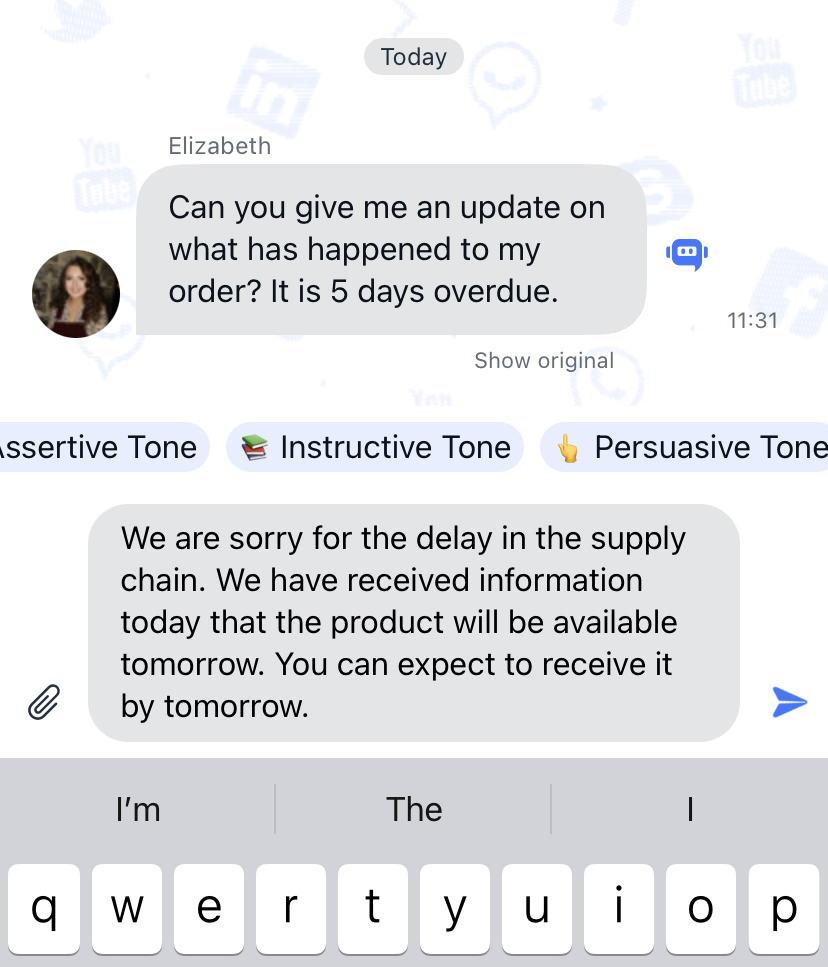
Users have the ability to seamlessly switch between different tones without having to rewrite the original message.
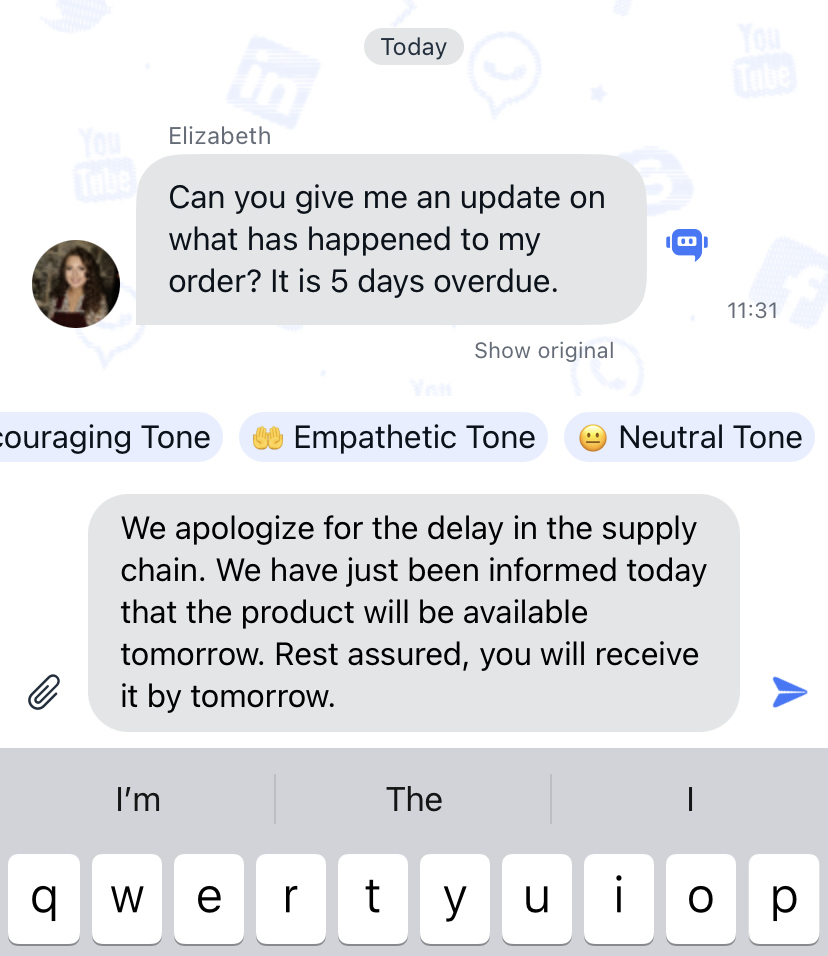
After rephrasing a message, the user is given an easily accessible option to return to the original.
Clicking on this "Back to original" option will instantly restore the message to its original state.
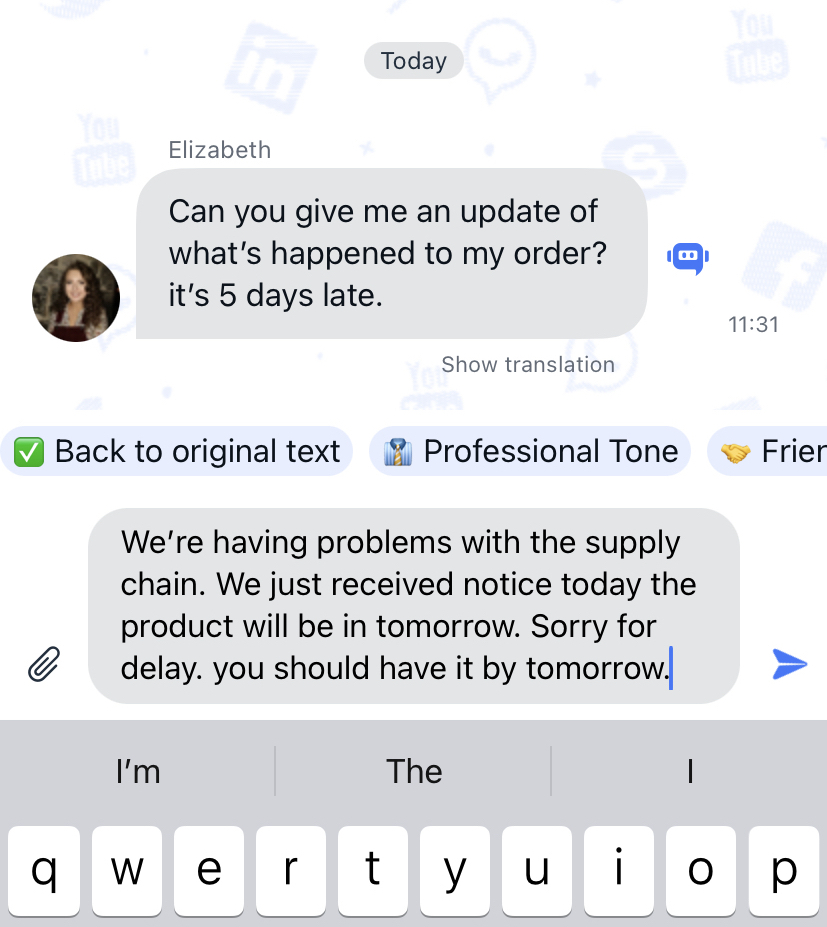
-
Set up the AI settings by providing either the OpenAI API key:
QuickBloxUIKit.feature.ai.rephrase.apiKey = "YOUR_OPENAI_API_KEY"
Or set up with a proxy server:
QuickBloxUIKit.feature.ai.rephrase.serverPath = "https://your-proxy-server-url"
We recommend using a proxy server like the QuickBlox AI Assistant Proxy Server offers significant benefits in terms of security and functionality:
- When making direct requests to the OpenAI API from the client-side, sensitive information like API keys may be exposed. By using a proxy server, the API keys are securely stored on the server-side, reducing the risk of unauthorized access or potential breaches.
- The proxy server can implement access control mechanisms, ensuring that only authenticated and authorized users with valid QuickBlox user tokens can access the OpenAI API. This adds an extra layer of security to the communication.
-
A developer using the AI Rephrase library has the ability use to Default Tones and Default AIRephraseSettings.
public class AIRephraseSettings { public var tones: [QBAIRephrase.AITone] = [ QBAIRephrase.AITone.professional, QBAIRephrase.AITone.friendly, QBAIRephrase.AITone.encouraging, QBAIRephrase.AITone.empathetic, QBAIRephrase.AITone.neutral, QBAIRephrase.AITone.assertive, QBAIRephrase.AITone.instructive, QBAIRephrase.AITone.persuasive, QBAIRephrase.AITone.sarcastic, QBAIRephrase.AITone.poetic ] /// Determines if assist answer functionality is enabled. public var enable: Bool = true /// The OpenAI API key for direct API requests (if not using a proxy server). public var apiKey: String = "" /// The URL path of the proxy server for more secure communication (if not using the API key directly). /// [QuickBlox AI Assistant Proxy Server](https://github.com/QuickBlox/qb-ai-assistant-proxy-server). public var serverPath: String = "" /// Represents the available API versions for OpenAI. public var apiVersion: QBAIRephrase.APIVersion = .v1 /// Optional organization information for OpenAI requests. public var organization: String? = nil /// Represents the available GPT models for OpenAI. public var model: QBAIRephrase.Model = .gpt3_5_turbo /// The temperature setting for generating responses (higher values make output more random). public var temperature: Float = 0.5 /// The maximum number of tokens to generate in the request. public var maxRequestTokens: Int = 3000 /// The maximum number of tokens to generate in the response. public var maxResponseTokens: Int? = nil }
-
A developer using the AI Rephrase library has the ability to delete tones, create his own tones, and add them to tailor the user interface to his needs. Also a developer has the ability to setup custom settings. This is an example of creating custom tones and installing them in QuickBlox iOS UI Kit from the custom application.
import QBAIRephrase // Custom Tones public extension QBAIRephrase.AITone { static let youth = QBAIRephrase.AITone ( name: "Youth", description: "This will allow you to edit messages so that they sound youthful and less formal, using youth slang vocabulary that includes juvenile expressions, unclear sentence structure and without maintaining a formal tone. This will avoid formal speech and ensure appropriate youth greetings and signatures.", icon: "🛹" ) static let doctor = QBAIRephrase.AITone ( name: "Doctor", description: "This will allow you to edit messages so that they sound doctoral, using medical and medical vocabulary, including professional expressions, unclear sentence structure. This will allow you to make speeches in a medical-doctoral tone and provide appropriate medical greetings and signatures.", icon: "🩺" ) } // Array of required tones for your application. public var customTones: [QBAIRephrase.AITone] = [ .youth, // Custom Tone .doctor, // Custom Tone .sarcastic, // Default Tone .friendly, // Default Tone .empathetic, // Default Tone .neutral, // Default Tone .poetic // Default Tone ] // Setup an array of required tones for your application. QuickBloxUIKit.feature.ai.rephrase.tones = customTones // Setup custom settings for Rephrase. QuickBloxUIKit.feature.ai.rephrase.organization = "CustomDev" QuickBloxUIKit.feature.ai.rephrase.model = .gpt4 QuickBloxUIKit.feature.ai.rephrase.temperature = 0.8 QuickBloxUIKit.feature.ai.rephrase.maxRequestTokens = 3500
More examples of setting custom settings for the appearance of user interface elements from a user application can be found in our UIKitSample.
-
A developer using the AI Rephrase library has the ability to customize the Appearance of UI Rephrase elements to adapt the user interface to their needs.
// Default UI Settings for Rephrase public struct AIRephraseUISettings { public var nameForeground: Color public var nameFont: Font public var iconFont: Font public var bubbleBackground: Color public var bubbleRadius: CGFloat = 12.5 public var contentSpacing: CGFloat = 4.0 public var height: CGFloat = 25.0 public var buttonHeight: CGFloat = 38.0 public var contentPadding: EdgeInsets = EdgeInsets(top: 6, leading: 4, bottom: 6, trailing: 4) public var bubblePadding: EdgeInsets = EdgeInsets(top: 2, leading: 8, bottom: 2, trailing: 8) public init(_ theme: ThemeProtocol) { self.nameForeground = theme.color.mainText self.nameFont = theme.font.callout self.iconFont = theme.font.caption self.bubbleBackground = theme.color.outgoingBackground } }
This is an example of setting custom settings for the appearance of UI elements from a custom application.
QuickBloxUIKit.feature.ai.ui.rephrase.bubbleBackground = .green QuickBloxUIKit.feature.ai.ui.rephrase.nameForeground = .red
More examples of setting custom settings for the appearance of user interface elements from a user application can be found in our UIKitSample.
Incorporate AI Rephrase into your iOS chat application seamlessly using the QBAIRephrase Swift package. Unlock the potential of AI-driven interactions and provide your users with real-time translation capabilities, enhancing user communication and experience.
For additional resources, explore the QuickBlox AI Assistant Proxy Server and the QBAIRephrase Swift package repositories.
Resources:
Default tones
- Professional tone: This will allow you to edit messages to sound more formal, using technical language, clear sentence structures, and maintaining a respectful tone. This would avoid colloquial language and ensure appropriate greetings and signatures.
- Friendly Tone: This will allow you to tailor your messages to reflect a casual, friendly tone. It will include casual language, use emoticons, exclamation points and other informal elements to make the message seem more friendly and approachable.
- Encouraging tone: This tone will be useful for motivation and encouragement. It will include positive words, affirmations and express support and faith in the recipient.
- Empathic Tone: This tone will be used to show understanding and empathy. This will require softer language, acknowledgment of feelings, and demonstrations of compassion and support.
- Neutral Tone: For when you want to maintain an even, impartial and objective tone. He will avoid harsh statements and emotional words, preferring clear and direct communication.
- Assertive Tone: This tone is useful for making a clear statement, asserting a position, or in negotiations. He uses direct speech, is confident and does not soften his words.
- Instructive Tone: This tone would be useful for tutorials, guides, or other teaching and learning materials. It is clear, concise and logically guides the reader through the steps or processes.
- Persuasive Tone: This tone can be used when trying to convince someone or to argue your point. He uses persuasive language, strong words and logical reasoning.
- Sarcastic/Ironic Tone: This tone can make the communication more humorous or convey an ironic attitude. It is more difficult to implement because it requires the AI to understand the nuances of the language and may not always be perceived by the reader as intended.
- Poetic Tone: This will add an artistic touch to messages by using figurative language, rhyme, and rhythm to create more expressive text.
Updated about 1 year ago