Screens
Overview
QuickBlox IOS UIKit provides customizable screens and basic chat features such as dialog list, dialog creation, chat in dialogs, and dialog editing out of the box. Screens are made up of components, a ScreenSettings
for that screen, and a ViewModel
that provides the screen's functionality.
Each screen has custom user interface components. Each screen has a specific ScreenSettings
that creates the view and each ScreenSettings
contains customizable UI components. A screen also has a corresponding ViewModel
that provides the necessary data from QuickBlox IOS SDK.
Refer to the table below to see which screens we provide and the components that make up each screen.
Screen | ScreenView | ScreenSettings | ViewModel | Components |
---|---|---|---|---|
Dialog list | DialogsView | DialogsScreenSettings | DialogsViewModel | DialogListHeader |
Private Dialog | PrivateDialogView | DialogScreenSettings | DialogViewModel | DialogHeader |
Private Dialog info | PrivateDialogInfoView | DialogInfoScreenSettings | DialogInfoViewModel | PrivateDialogInfoHeader |
Create Private Dialog | CreateDialogView | CreateDialogScreenSettings | CreateDialogViewModel | CreateDialogHeader |
Dialog Type | DialogTypeView | DialogTypeScreenSettings | DialogTypeHeaderView | |
Group Dialog | GroupDialogView | DialogScreenSettings | DialogViewModel | DialogHeader |
Group Dialog info | GroupDialogInfoView | DialogInfoScreenSettings | DialogInfoViewModel | DialogInfoHeader |
Members | RemoveMembersView | MembersScreenSettings | MembersDialogViewModel | MembersHeader |
Add members | AddMembersDialogView | AddMembersScreenSettings | AddMembersDialogViewModel | AddMembersHeader |
New Dialog | NewDialog | DialogNameScreenSettings | NewDialogViewModel | DialogNameHeader |
Create Group Dialog | CreateDialogView | CreateDialogScreenSettings | CreateDialogViewModel | CreateDialogHeader |
Dialog list screen
Overview
The Dialog List feature in QuickBlox iOS UI Kit provides a user interface for displaying and managing a list of dialogs in an iOS application. It allows users to view, select, and perform actions on individual dialogs, such as deleting or leaving a dialog.
Features
- Display a list of dialogs: The
DialogsListView
component presents a visually appealing list of dialogs in the application.
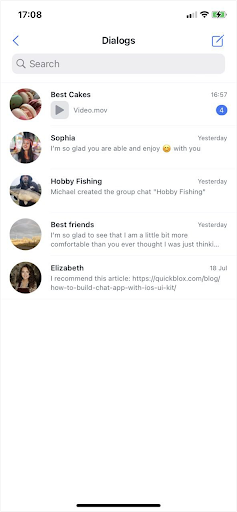
- Select and view dialog details: Users can select a dialog from the list to view more details about the selected dialog.
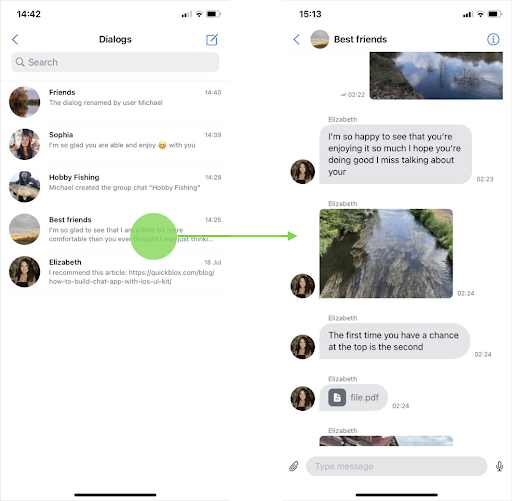
- Delete dialogs: The feature allows users to delete dialogs from the list, removing them from their conversation history.
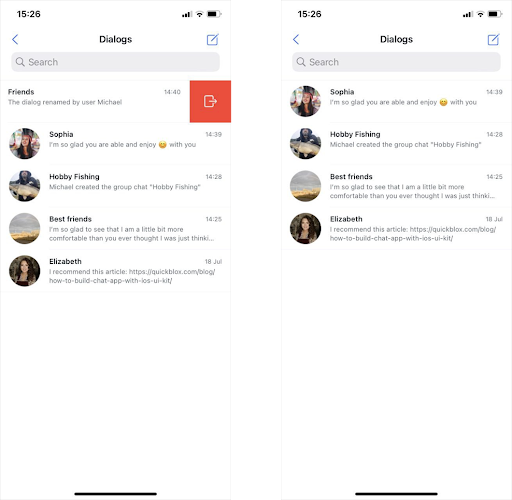
- Search functionality: Users can search for specific dialogs using the provided search bar.
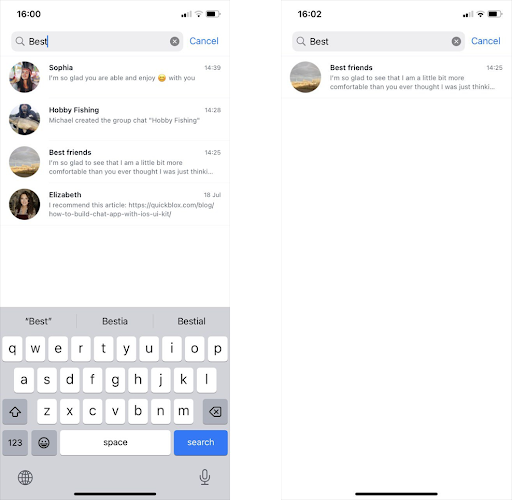
- Sync state indication: The sync state is provided to indicate whether the dialog list is currently syncing data with the server.
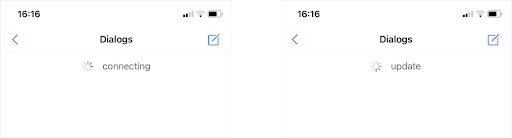
Usage
To use the DialogsListView
component in your SwiftUI view, follow these steps:
Create an instance of DialogsViewModel
with the appropriate DialogsRepository
instance.
Define the content views for the list view and the detail view for individual dialogs.
Customize the appearance and behavior of the DialogsListView
using the available configuration options.
Embed the DialogsListView
struct in your SwiftUI view hierarchy.
Here's an example of how to use the DialogsViewModel
feature:
The dialogs list shows the complete list of group and private dialogs of which the current user is a member.
After following the steps described in the Get start section, you can display and manage the list of dialogs.
Private Dialog screen
Overview
A private dialog is a chat that allows close interaction between two users. To participate in this type of dialog, you can create a dialog with your opponent or your opponent can create a dialog with you.
The QuickBlox iOS UI Kit offers comprehensive support for various message types to be sent within private dialogs. Users can send plain text messages, file messages, and media content like photos and videos seamlessly.
Once delivered, these messages are automatically organized and grouped within the dialog. The grouping is based on time in minutes and date, allowing for a clear and organized display of the messages in the dialog's chat history.
With this functionality, users can engage in effective communication, sharing a wide range of content types, and easily navigate through the chat history based on the chronological grouping of messages. This feature enhances the overall messaging experience and ensures a structured presentation of messages in private dialogs.
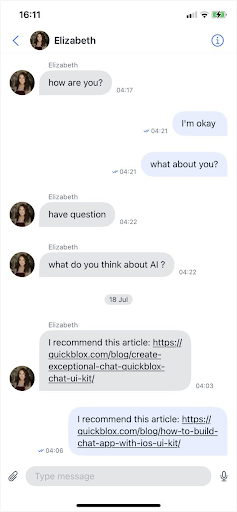
Features
- Sending messages allows users to send messages, images, videos and files in message input mode.
- Delivery Notice displays the delivery notification status of a message in a dialog.
- Read notification displays the status of receiving a read message in a dialog.
- Typing indicator displays whether another user is typing a message in the dialog.
Function List
sendMessage(\_ text: String)
Sending various types of messages.handleOnSelect(attachment: AttachmentAsset)
Preparing and Sending Messages with Attachments.sendTyping()
Sending signals while the User is composing a message. The user actively interacts with the message input interface specific to this chat session (for example, by entering text in the input area of the chat window).sendStopTyping(
) Sending signals when the User has been writing but has now stopped. The user wrote but did not interact with the message input interface for a short period of time (eg 30 seconds).handleOnAppear(\_ message: MessageItem)
Processing a message that appears from a dialog participant.startRecording()
Start recording a voice message.stopRecording()
Stop recording a voice message.deleteRecording()
Delete a voice message.playAudio(\_ audioData: Data, action: MessageAttachmentAction)
Play audio files, including voice messages.stopPlayng()
Stops playback of the audio file.unsubscribe()
Unsubscribe from receiving signals from publishers.
Usage
List Component
The "Private Dialog screen" function uses a ListComponent to provide information about the message exchange. The ListComponent contains various MessageRowView
types, each representing a different kind of message, such as simple text messages, file messages, and multimedia content such as photos and videos. This screen also contains a field for entering the text of the message, recording a voice message and accessing the Photo Gallery and Files.
View model
The "Private Dialog screen" function uses the DialogViewModel
class as the view model, which conforms to the DialogViewModelProtocol
. The view model handles the presentation logic and messaging and managing chat history data. It provides published properties for dialog-related information and chat interaction methods such as sending messages and interacting with features such as the Typing indicator.
Private Dialog info screen
Overview
The "Private Dialog info" function in the QuickBlox iOS UI Kit provides a user-friendly interface for viewing chat information. It allows users to access information related to a particular chat, such as the name and avatar of the participant. In addition, users can log out of the chat.
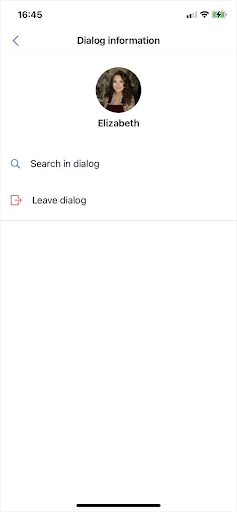
Features
- Display important information about the chat, including the participant's name and avatar.
- Allow the user to log out of the chat.
Function List
- deleteDialog: Delete chat.
Usage
The "Private Dialog info" function is integrated into the QuickBlox iOS UI Kit and users can easily access it from the chat interface. Users can go to the chat information screen to view chat details.
List Component
The "Private Dialog info" function uses a ListComponent to provide a structured view of chat information and actions. The ListComponent contains various segments, each representing a different chat action, such as searching the dialog, leaving the chat, and managing notifications.
View model
The Chat Info function uses the DialogInfoViewModel
class as a view model that conforms to the DialogInfoProtocol
. The view model handles the presentation logic and data management for the chat information. It provides published properties for dialog-related information and chat interaction methods, such as deleting a chat.
Create Private Dialog screen
Overview
The "Create Private Dialog" function is a key feature of the QuickBlox iOS UI Kit that allows users to create new private dialogs with the participants they want. It provides an easy and efficient way to create private conversations with specific participants.
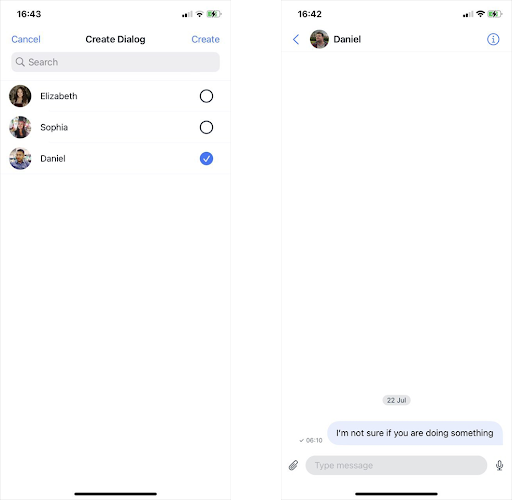
Features
- Search and Filter: Users can search for specific people to add to a private chat, and this feature will automatically filter and display the relevant results.
- Real-time Updates: This feature uses aggregation to update the displayed list of users in real time based on search criteria.
- Selecting the necessary chat participant and manipulating them.
- Create a private chat with the necessary chat participant.
Usage
To use the "Create Private Dialog" function in your QuickBlox iOS app, follow these steps:
- Create a
modeldDialog: Dialog
object representing the private chat you will set up for the desired users on this screen to complete the setup of the new dialog and create it. - Instantiate the
CreateDialogViewModel
by passing amodeldDialog: Dialog
object as a parameter. - Monitor search property changes to dynamically update the displayed list of users based on search criteria.
- To set up a user for a private chat, select the user from the displayed list by clicking on their cell by calling the
handleOnSelect(\_ item: UserItem)
method and you will notice that the selection is confirmed with a checkbox. - To remove a user from the member list, click the user by calling the
handleOnSelect(\_ item: UserItem)
method and the check box will return to its default state. - Select the required user and call the
createDialog()
method by clicking on the "Create" button. - After successfully creating a new private chat, it will automatically go to the Private Dialog screen with that dialog.
ListComponent
The user interface of the "Create Private Dialog" function consists of a ListComponent that displays a list of users that can be configured for a private chat. The ListComponent is dynamic and updates in real time depending on the search criteria. This allows users to scroll through the available users and select the right user to install in the conversation.
View Model
CreateDialogViewModel
is the view model responsible for managing the screen of the private creation dialog. It handles user interaction, search functionality, and updates the displayed list of users. The view model also manages asynchronous tasks with Task\<Void, Never>
and AnyCancellable
in Combine
. It provides efficient and secure handling of UI updates and background operations.
CreateDialogViewModel
is equipped with a set of methods, including sync() to synchronize dialog members, handleOnSelect(\_ item: UserItem)
to set user to a private dialog, and createDialog() to create a private chat.
Dialog Type screen
Overview
The "Dialog Type" function is a key feature of the QuickBlox iOS UI Kit that allows users to select the desired conversation type to create a new dialog. This can be a private conversation or a group conversation.
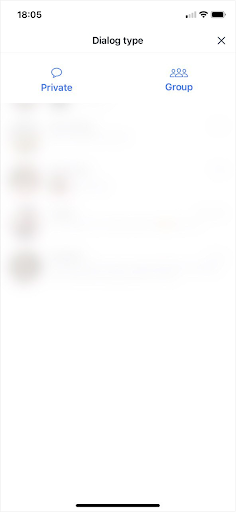
Features
- Select the desired dialog type to create a new dialog. It can be a personal conversation or a group conversation.
Usage
To use the "Dialog Type" function in your QuickBlox iOS app, follow these steps:
- Select the desired dialog type to create a new dialog. If a Private Dialog is selected, the Create Private Dialog screen will open, and if a Group Dialog is selected, the Group Dialog name screen will open.
Components
The "Dialog Type" function UI onsists of a DialogTypeBar and is a segmented control for selecting the desired dialog type to create a new dialog. This allows users to select the desired type of conversation to create a new conversation.
Group Dialog screen
Overview
A "Group Dialog" is a chat that allows close interaction between a specific group of users. To participate in this type of dialogue, you can create a group dialogue with a group of opponents.
QuickBlox iOS UI Kit offers comprehensive support for various types of messages to send in group conversations. Users can seamlessly send simple text messages, file messages, and multimedia content such as photos and videos.
Once delivered, these messages are automatically organized and grouped in a dialog box. The grouping is based on time in minutes and date, which allows messages to be displayed clearly and organized in the dialog box's chat history.
With this feature, users can engage in effective communication, share a wide range of content types, and easily navigate through chat history based on the chronological grouping of messages. This feature improves the overall messaging experience and provides a structured view of messages in group dialogs.
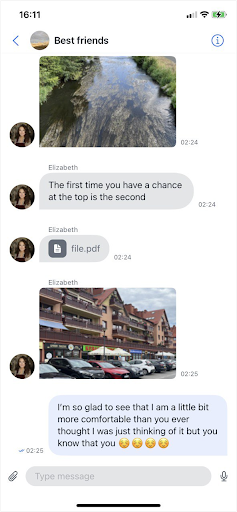
functions
- Sending messages allows users to send messages, images, videos and files in message input mode.
- Delivery Notice displays the delivery notification status of a message in a dialog.
- Read notification displays the status of receiving a read message in a dialog.
- Typing indicator displays whether another user is typing a message in the dialog.
Function List
- sendMessage(_ text: String) Sending various types of messages.
- handleOnSelect(attachment: AttachmentAsset) Preparing and Sending Messages with Attachments.
- sendTyping() Sending signals while the User is composing a message. The user actively interacts with the message input interface specific to this chat session (for example, by entering text in the input area of the chat window).
- handleOnAppear(_ message: MessageItem) Processing a message that appears from a dialog participant.
- sendStopTyping() Sending signals when the User has been writing but has now stopped. The user wrote but did not interact with the message input interface for a short period of time (eg 30 seconds).
- startRecording() Start recording a voice message.
- stopRecording() Stop recording a voice message.
- deleteRecording() Delete a voice message.
- playAudio(_ audioData: Data, action: MessageAttachmentAction) Play audio files, including voice messages.
- stopPlayng() Stops playback of the audio file.
- unsubscribe() Unsubscribe from receiving signals from publishers.
Usage
List Component
The "Group Dialog screen" function uses a ListComponent to provide information about the message exchange. The ListComponent contains various MessageRowView types, each representing a different kind of message, such as simple text messages, file messages, and multimedia content such as photos and videos. This screen also contains a field for entering the text of the message, recording a voice message and accessing the Photo Gallery and Files.
View model
The "Group Dialog screen" function uses the DialogViewModel class as the view model, which conforms to the DialogViewModelProtocol. The view model handles the presentation logic and messaging and managing chat history data. It provides published properties for dialog-related information and chat interaction methods such as sending messages and interacting with features such as the Typing indicator.
Group Dialog info screen
Overview
The "Chat Info" function in the QuickBlox iOS UI Kit provides a user-friendly interface to view and manage chat information. It allows users to access details related to a specific chat, such as the chat name, members, avatar, and other relevant data. In addition, users can perform various actions such as viewing members and leaving the chat. The chat creator can edit the chat name and change the chat avatar.
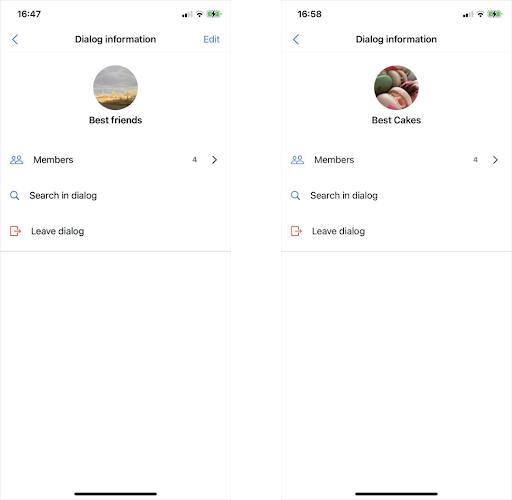
Features
- Display essential information about a chat, including chat name and avatar.
- Allow users to edit the chat name and update the chat avatar. Available to the chat creator.
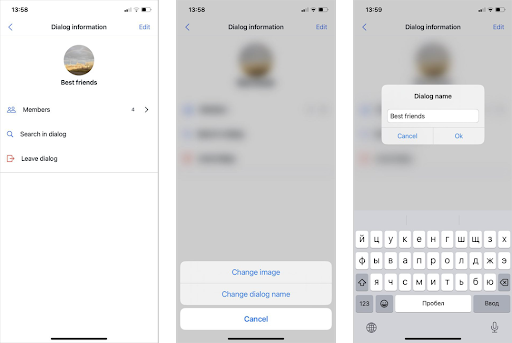
- Provide options to view and manage chat members.
- Support both private and group chat information display.
- Allow the user to log out of the chat.
Function List
- updateDialog: Update the chat's information, such as name and avatar.
- handleOnSelect(attachmentAsset:): Handle the selection of an attachment asset (e.g., image) for updating the chat avatar.
- removeExistingImage: Remove the existing chat avatar.
- removeDialogAvatar: Remove the chat avatar and update the chat information.
- handleOnSelect(newName:): Handle the selection of a new chat name and update the chat information.
- deleteDialog: Delete the chat.
Usage
The "Chat Info" feature is integrated into the QuickBlox iOS UI Kit and can be easily accessed by users from the chat interface. Users can navigate to the chat info screen to view and manage the chat details. The UI Kit provides different views for group chats based on the user's permission to edit the chat.
ListComponent
The "Chat Info" function utilizes a ListComponent to present the chat information and actions in a structured manner. The ListComponent contains various segments, each representing different chat actions, such as viewing members, searching in the dialog, leaving the chat, and managing notifications.
View Model
The "Chat Info" function uses a DialogInfoViewModel class as its View Model, which conforms to the DialogInfoProtocol. The View Model handles the presentation logic and data management for the chat information. It provides published properties for dialog-related information and methods to interact with the chat, such as updating the chat, handling user selections, and deleting the chat.
Members (Remove users) screen
Overview
The "Members (Remove users)" function is a key feature of the QuickBlox iOS UI Kit that allows users to remove participants from a group chat. It provides a user-friendly interface to manage the members of a group chat and control who can participate in the conversation.
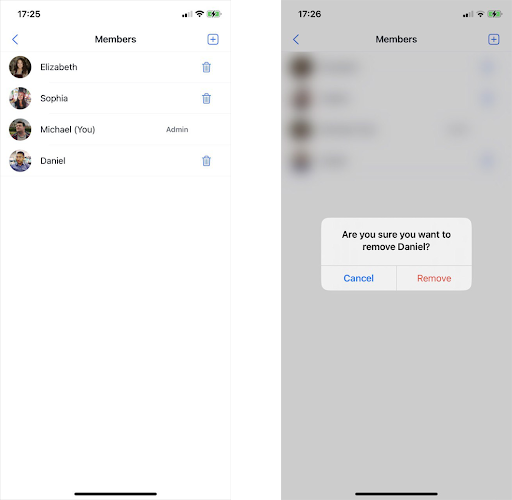
Features:
- Display Participants: The function displays a list of participants in the group chat, showing their names and other relevant details.
- User Removal: Users with administrative privileges can select and remove participants from the chat, ensuring efficient chat management.
- Alert Confirmation: When an admin attempts to remove a user, an alert prompt is presented, confirming their intent before the action is executed.
- Add Members: The function includes an option to add new members to the group chat through a navigation link to the "Add Members" screen.
Function List:
- removeUserFromDialog(): This function is responsible for removing the selected user from the group chat. It triggers an update to the dialog with the updated participant list, effectively removing the user from the chat.
Usage:
The "Members (Remove users)" function is integrated into the QuickBlox iOS UI Kit's group chat management system. Users with admin privileges can access this functionality from the group chat screen. Upon selecting a user to remove, an alert will appear, requesting confirmation for the removal action. Once confirmed, the selected user will be removed from the group chat.
ListComponent:
The RemoveUserListView is a SwiftUI component responsible for displaying the list of participants in the group chat and enabling the user to select users for removal. It includes options for handling user selection and pagination for large participant lists.
View Model:
The MembersDialogViewModel class serves as the view model for the "Members (Remove users)" function. It manages the state of the view, including the list of displayed users, the selected user to be removed, and the current search text. The view model handles interactions with the QuickBlox backend, ensuring data synchronization, fetching user information, and updating the dialog with the latest changes.
Add Members screen
Overview
The "Add Members" function is a key feature of the QuickBlox iOS UI Kit that allows users to add new members to a group chat. It provides an easy and efficient way to expand group conversations by inviting new participants to join the conversation.
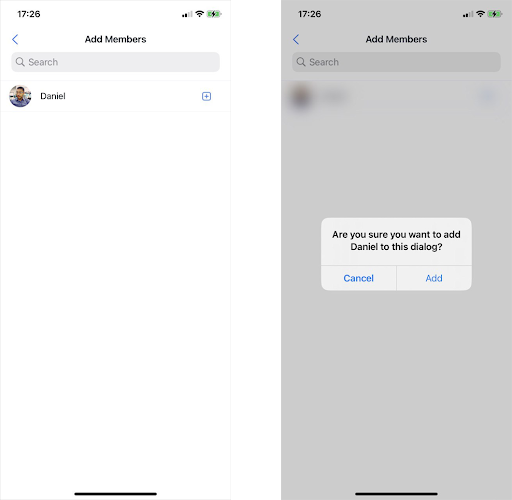
Features
- Search and Filter: Users can search for specific individuals to add to the group chat, and the function automatically filters and displays relevant results.
- Real-time Updates: The function leverages Combine, allowing for real-time updates of the displayed user list based on the search criteria.
Usage
To use the "Add Members" function in your QuickBlox iOS app, follow these steps:
- Instantiate a Dialog object representing the group chat you want to add users to.
- Create an instance of the AddMembersDialogViewModel, passing the Dialog object as a parameter.
- Observe changes to the search property to dynamically update the displayed user list based on the search criteria.
- To add a user to the group chat, select a user from the displayed list, and call the addSelectedUser() method.
ListComponent
The "Add Members" function UI consists of a ListComponent displaying the list of users that can be added to the group chat. The ListComponent is dynamic and updates in real-time based on the search criteria. It allows users to scroll through the available users and select the desired individuals to add.
View Model
The AddMembersDialogViewModel is the view model responsible for controlling the "Add Members" dialog. It handles user interactions, search functionality, and updates to the displayed user list. The view model also manages asynchronous tasks using Task<Void, Never> and Combine's AnyCancellable. It ensures efficient and safe handling of UI updates and background operations.
The AddMembersDialogViewModel is equipped with a set of methods, including sync() to synchronize dialog members, displayDialogMembers() to filter users based on the search query, and addSelectedUser() to add a selected user to the group chat.
New Dialog screen
Overview
The "New Dialog" feature is a key feature of the QuickBlox iOS UI Kit that allows users to create new group chats with the with the name already required and an optional avatar.
Features
- Dialog Avatar Selection: Users can select a desired image from the Photo Gallery and set it as the dialog avatar. This is an optional option to create a dialog.
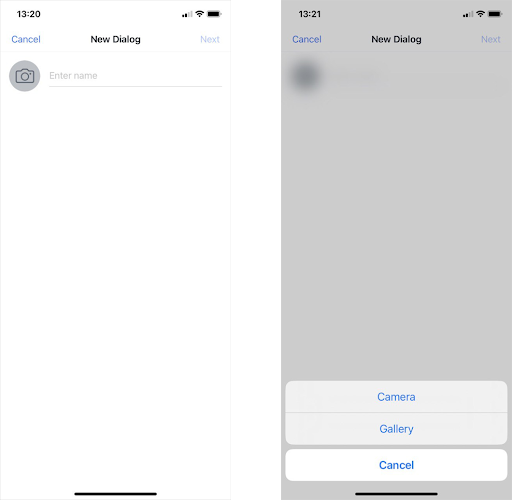
- Naming the Dialog: Users can give the desired name to the new dialog. This is a required option to create a dialog.
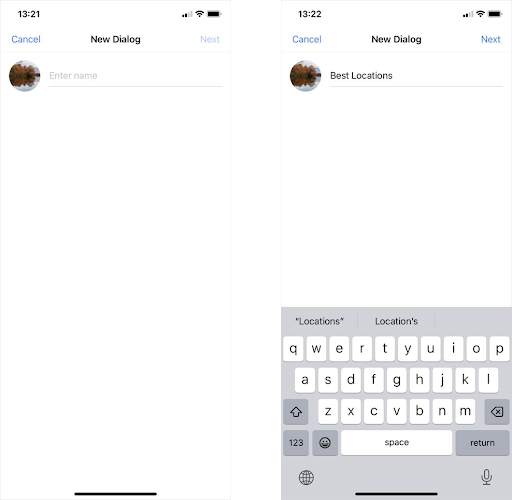
Usage
To use the "New Dialog" function in your QuickBlox iOS app, follow these steps:
- Select an avatar for the new dialog from the photo gallery and set it as the dialog's avatar. This is an optional parameter to create a dialog.
- Give the desired name to the new dialog box by typing it in the input field. The name must match these parameters: "Use alphanumeric characters and spaces in a range from 3 to 60. Cannot contain more than one space in a row.". This is a required option to create a dialog.
- Create a modelDialog: Dialog object representing the group chat to complete the setup of the new dialog.
Components
The user interface of the "New Dialog" function consists of a DialogPhoto to select an avatar for the new dialog from the photo gallery, and a DialogNameTextField with which you can give the desired name to the new dialog.
View Model
NewDialogViewModel is the view model responsible for managing the "New Dialog" screen. It handles the user interaction to select a photo for the dialog's avatar and enter the dialog's name. The view model also manages asynchronous tasks with Task<Void, Never> and AnyCancellable in Combine. It provides efficient and secure handling of UI updates and background operations.
The NewDialogViewModel is equipped with a set of methods, including handleOnSelect(attachmentAsset: AttachmentAsset) to set the dialog avatar photo, removeExistingImage() to remove the dialog avatar photo, and createDialogModel() to create a group chat model.
Create Group Dialog screen
Overview
The "Create Group Dialog" feature is a key feature of the QuickBlox iOS UI Kit that allows users to create new group chats with the members they want. It provides an easy and efficient way to create group conversations with specific participants.
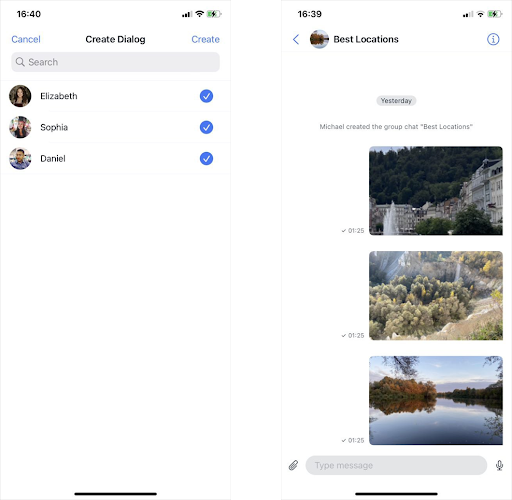
Features
- Search and Filter: Users can search for specific people to add to a group chat, and this feature will automatically filter and display the relevant results.
- Real-time Updates: This feature uses aggregation to update the displayed list of users in real time based on search criteria.
- Selecting the necessary chat participants and manipulating them.
- Create a group chat with the right members.
Usage
To use the "Create Group Dialog" function in your QuickBlox iOS app, follow these steps:
- Create a modeldDialog: Dialog object representing the group chat you will set up for the desired users on this screen to complete the setup of the new dialog and create it.
- Instantiate the CreateDialogViewModel by passing a modeldDialog: Dialog object as a parameter with the name already required and an optional avatar.
- Monitor search property changes to dynamically update the displayed list of users based on search criteria.
- To set up a user for a group chat, select the user from the displayed list by clicking on their cell by calling the handleOnSelect(_ item: UserItem) method and you will notice that the selection is confirmed with a checkbox.
- To remove a user from the member list, click the user by calling the handleOnSelect(_ item: UserItem) method and the check box will return to its default state.
- Select the required users and call the createDialog() method by clicking on the "Create" button.
- After successfully creating a new group chat, it will automatically go to the Group Dialog screen with that dialog.
ListComponent
The user interface of the "Create Group Dialog" function consists of a ListComponent that displays a list of users that can be configured for a group chat. The ListComponent is dynamic and updates in real time depending on the search criteria. This allows users to scroll through the available users and select the right people to install in the conversation.
View Model
CreateDialogViewModel is the view model responsible for managing the screen of the group creation dialog. It handles user interaction, search functionality, and updates the displayed list of users. The view model also manages asynchronous tasks with Task<Void, Never> and AnyCancellable in Combine. It provides efficient and secure handling of UI updates and background operations.
CreateDialogViewModel is equipped with a set of methods, including sync() to synchronize dialog members, handleOnSelect(_ item: UserItem) to set users to a group dialog, and createDialog() to create a group chat.
Updated over 1 year ago