Screen modules
Overview
QuickBlox React Chat UIKit provides customizable screen modules and essential chat functions such as a list of dialogs, dialog creation, in-dialog chat, and dialog editing out of the box. The screen modules consist of components with component settings for each of them, as well as the ViewModel model that provides the functionality of the screens. Each screen module has customizable user interface components.
By following the steps outlined in the Get Start section, you will be able to display QuickBlox React Chat UIKit.
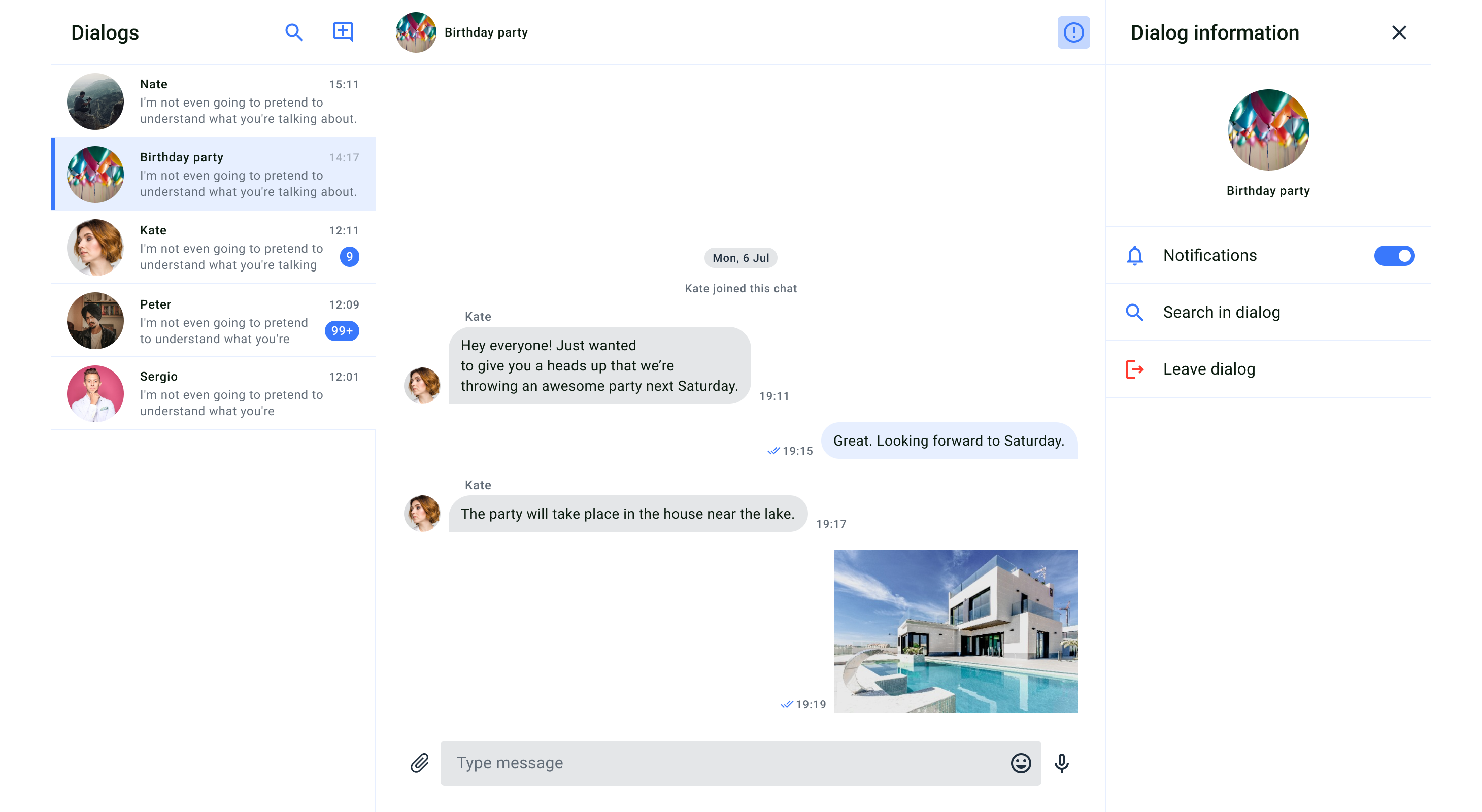
The QuickBlox React Chat UIKit screen is divided into three main screen modules:
- Dialogs List
- Message History for each dialog
- Dialog Information
Refer to the table below to see the screen modules we provide and the components that each screen module consists of.
Screen Module | ViewModel | Components |
---|---|---|
Dialogs | DialogsViewModel | HeaderDialogs PreviewDialog |
MessageView | DialogsViewModel, MessageViewModel | HeaderMessages, AudioAttachmentComponentProps HighLightLink ImageAttachmentComponent VideoAttachmentComponent VoiceRecordingProgress |
DialogInformation | DialogsViewModel, UserViewModel | MembersList, InviteMembers and UsersList |
Dialogs list screen
The dialog list displays a complete list of group and private dialogs that the current user is a member of.
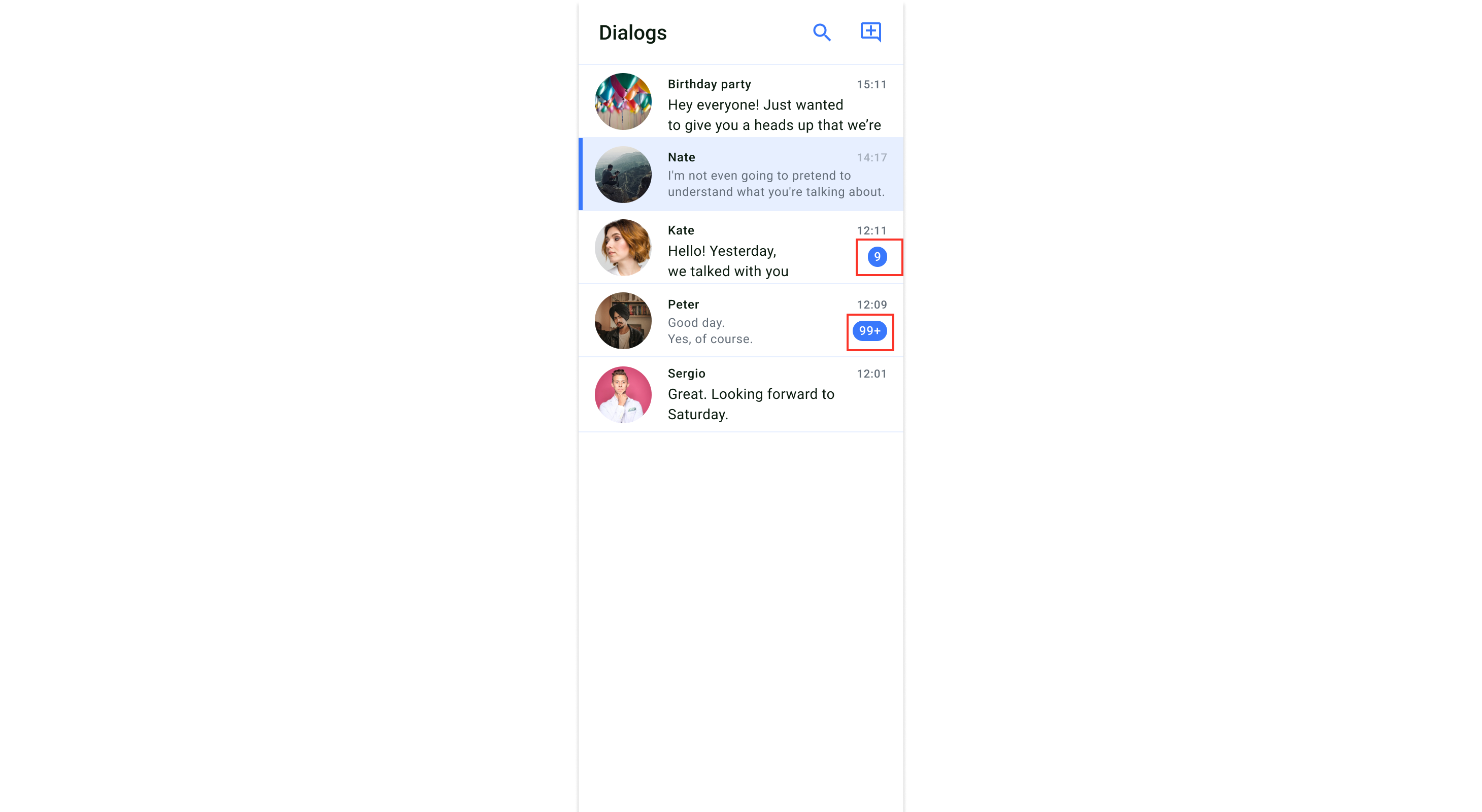
Screen structure
The screen with the list of dialogs is made up of two components: the header and the dialogs section.
Header
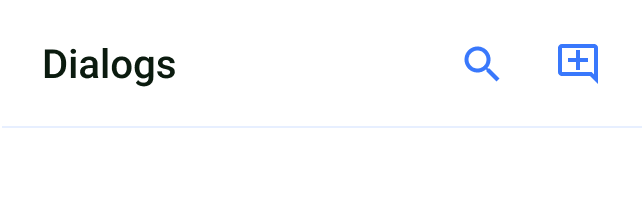
The header component includes the title for the dialog list and default buttons in the top right corner, which allow you to create dialogs and filter them.
The view of the header component is created and configured in the HeaderDialogsProps
.
Dialog list
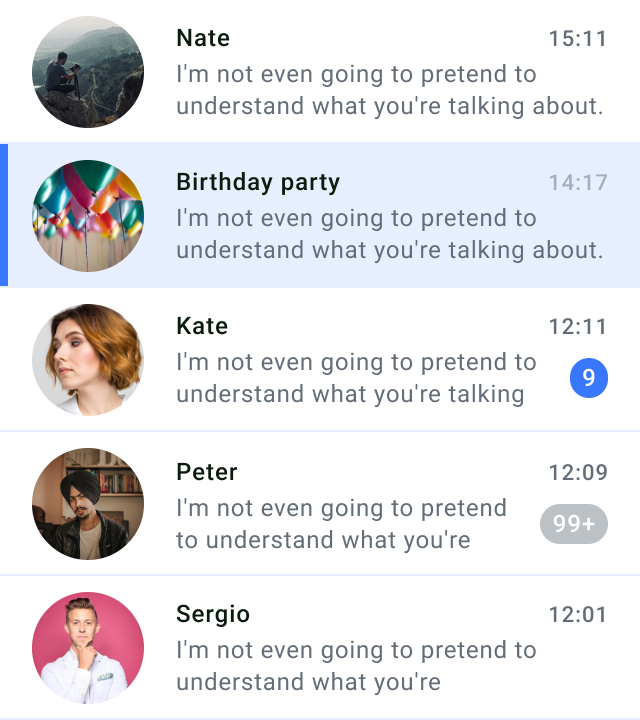
The dialogs component displays a list of all private and group dialogs that the current user is part of. The list of dialogs is generated in the Dialogs component based on data from the ViewModel. To create the view for each dialog list item, you need to use PreviewDialog
. When the current user clicks on one of the dialog windows in the list, they will be able to enter the chat screen.
Features
- Display a list of dialogs: The Dialog List component presents a visually appealing list of dialogs in the application.
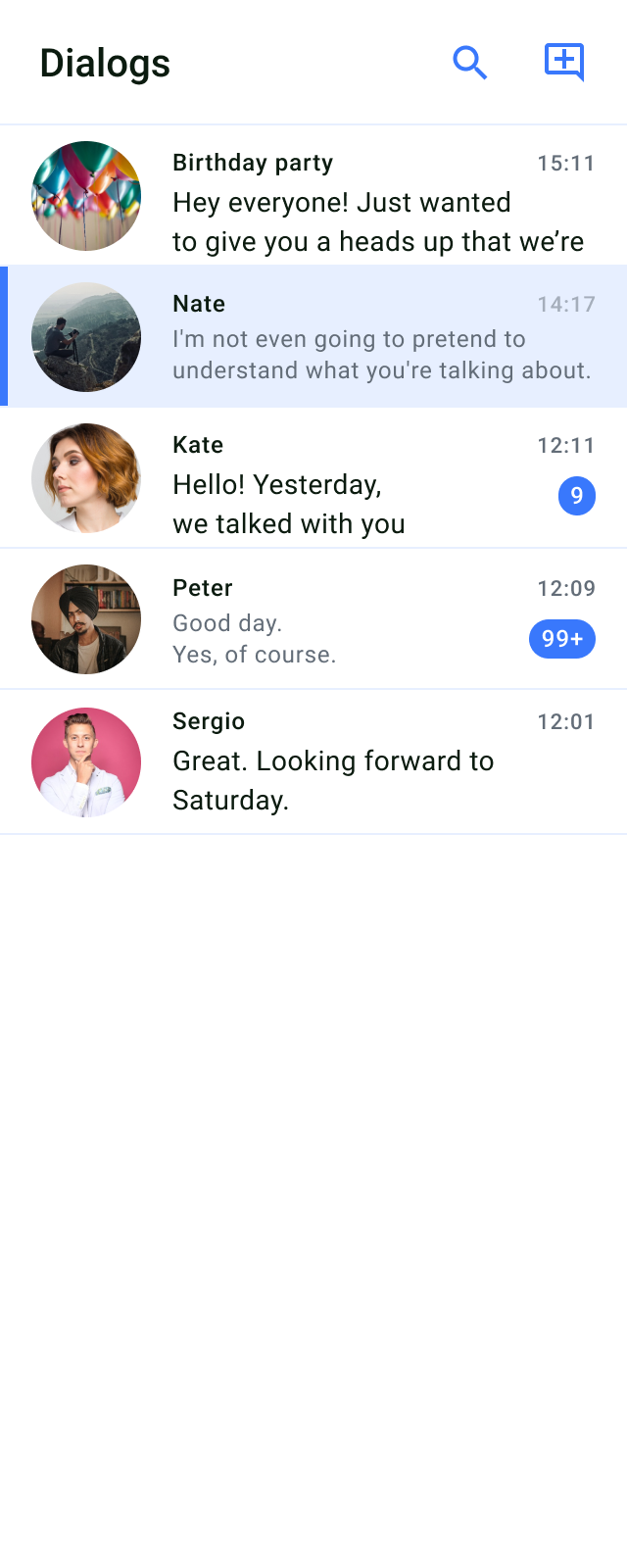
- Select and view dialog details: Users can select a dialog from the list to view more details about the selected dialog.
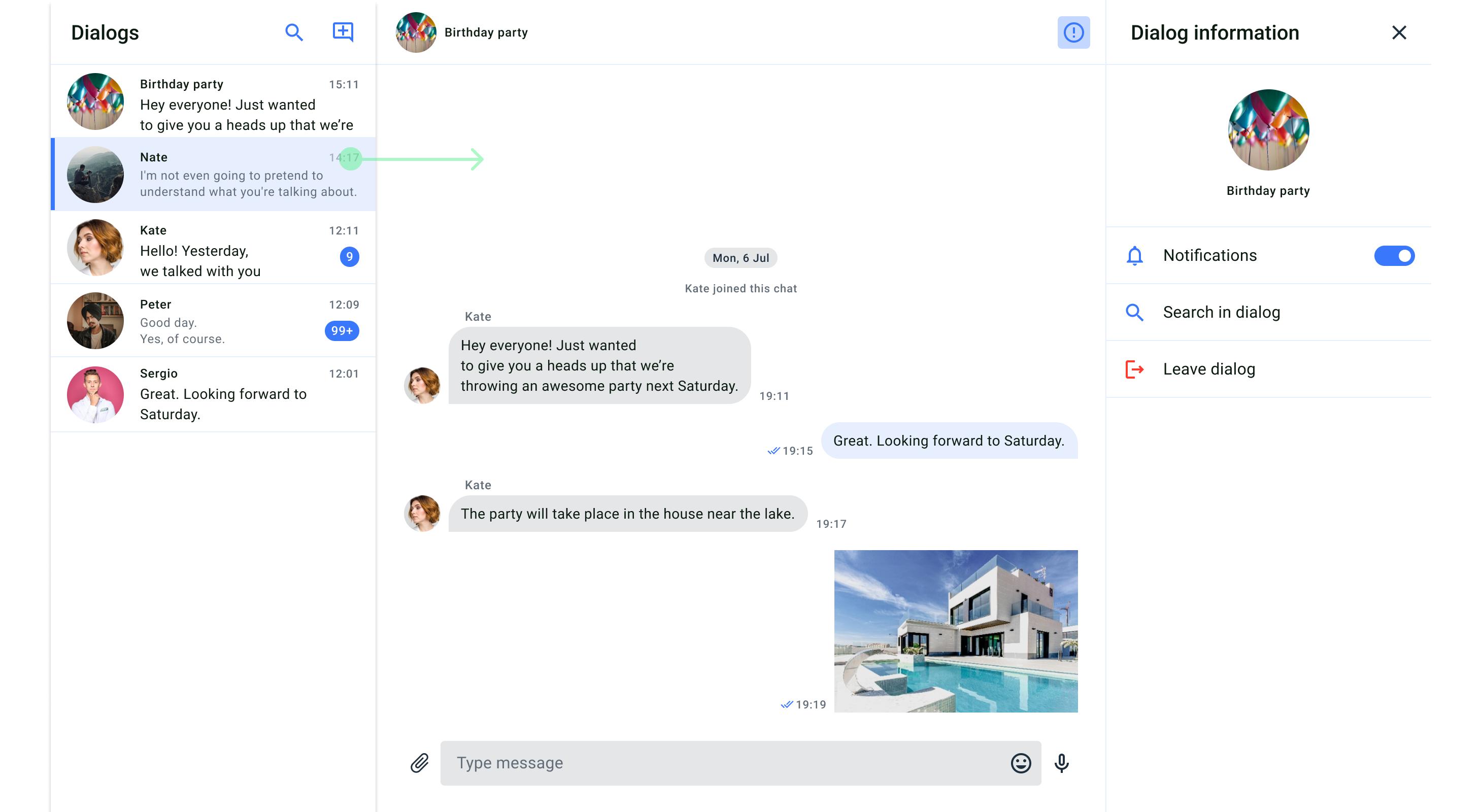
- Delete dialogs: The feature allows users to delete dialogs from the list, removing them from their conversation history.
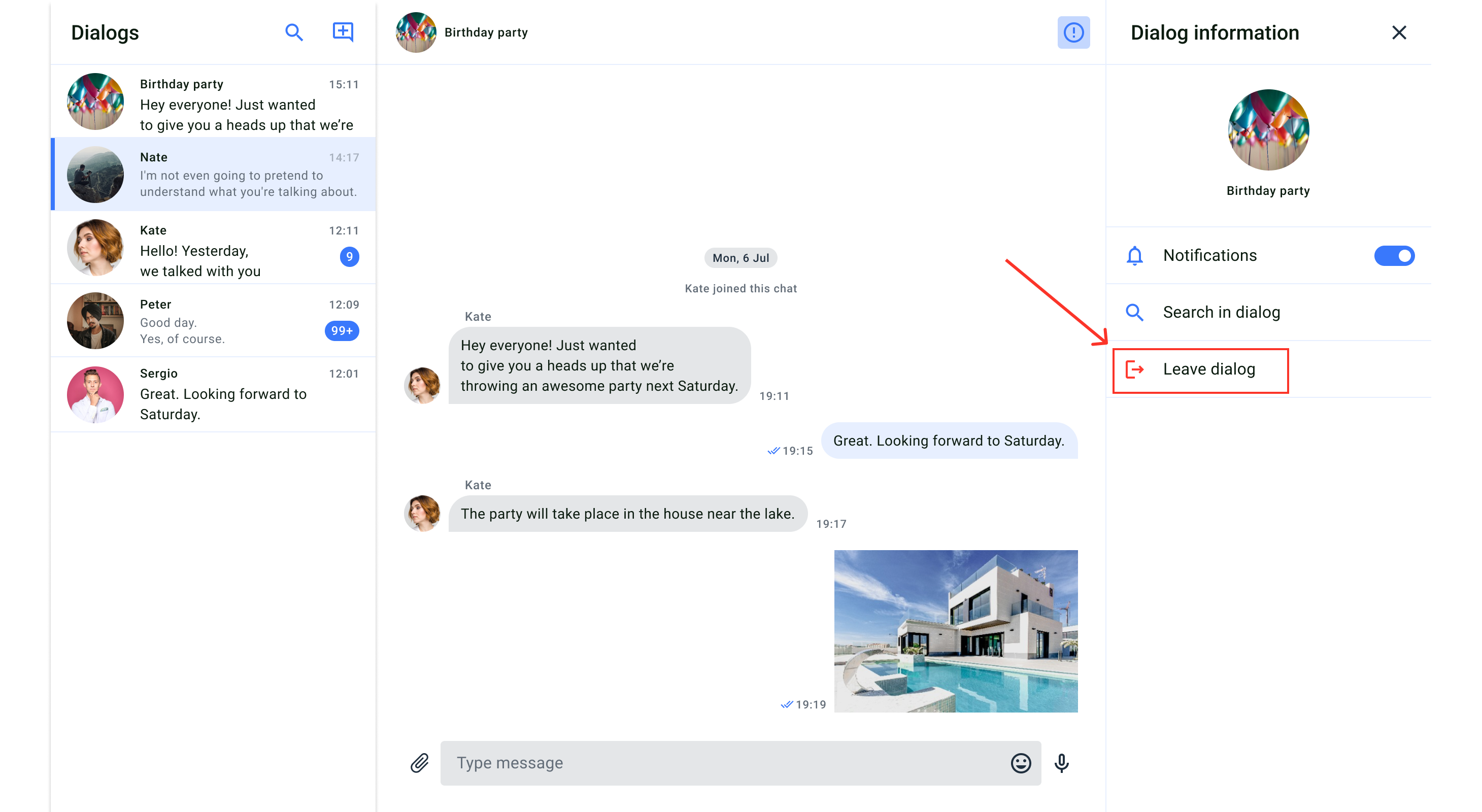
- Search functionality: Users can search for specific dialogs using the provided search bar.
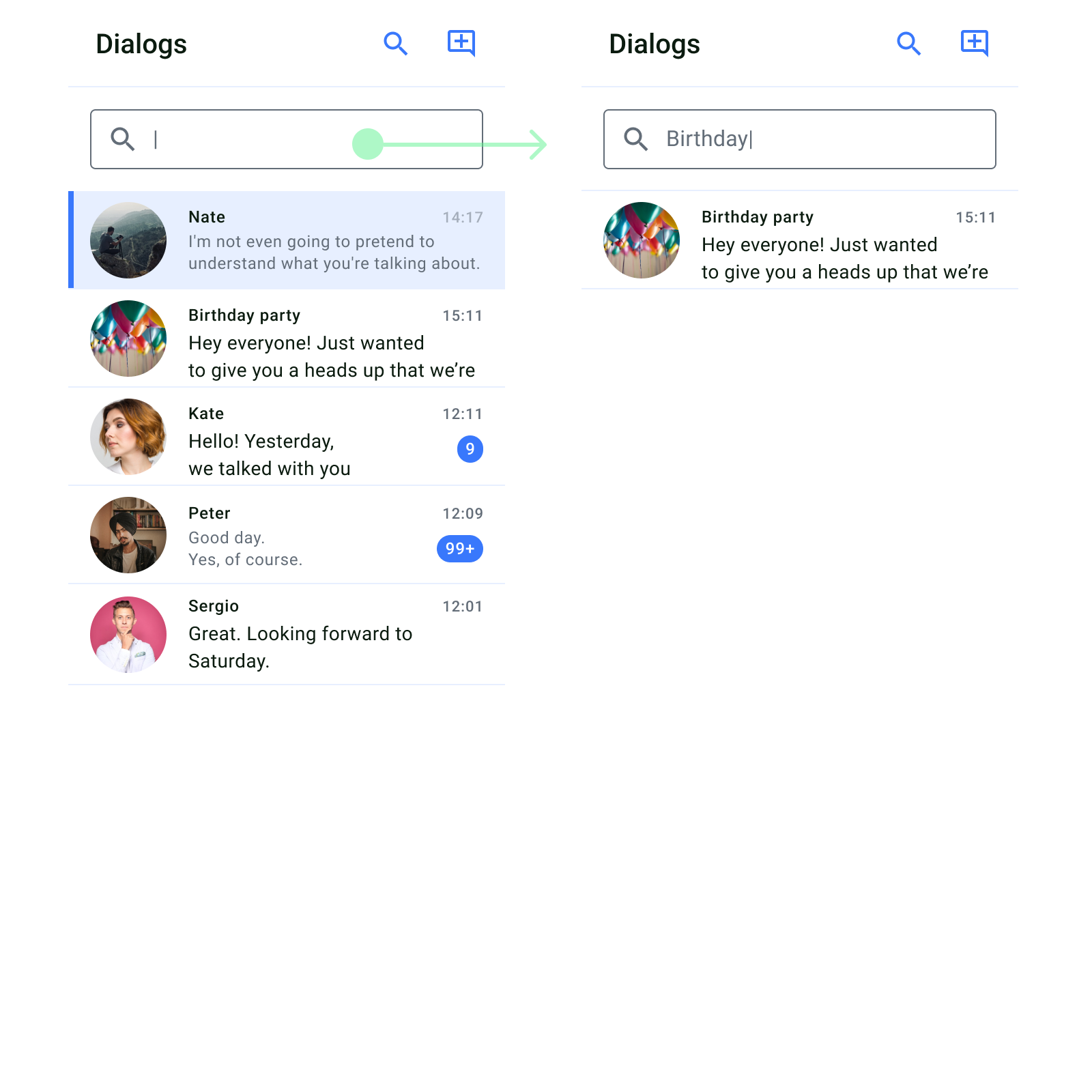
- Sync state indication: The sync state is provided to indicate whether the dialog list is currently syncing data with the server.
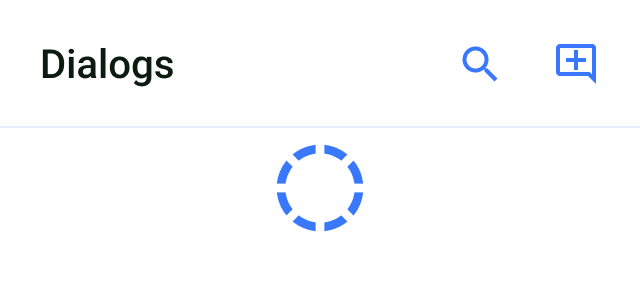
Usage
The Dialog List feature in QuickBlox UI Kit provides a user interface for displaying and managing a list of dialogs in an application. It allows users to view, select, and perform actions on individual dialogs, such as deleting or leaving a dialog. The "Dialog" component is designed to display a list of dialogs (chats) and consists of two parts: "HeaderDialog" (list header) and "PreviewDialog" (preview of a specific dialog). You can create your own custom component for the dialog header (as well as place your custom components above and below it). This allows you to add your logo to the UI Kit interface elements. However, the "PreviewDialog" component can only be customized through themes.
Note on PreviewDialog:
In the future versions of the UI Kit, you will be able to customize and use your own PreviewDialog component.
To use the Dialogs component in the project, you need to add it to the dialogsView section of the DesktopLayout layout component. We wrote about the DesktopLayout component in the Core Components section - here is the link. Below is a snippet of the DesktopLayout markup that uses the Dialogs component.
<DesktopLayout
theme={theme}
dialogsView={
<DialogsComponent
subHeaderContent={<CompanyDescription />}
upHeaderContent={<CompanyLogo />}
dialogsViewModel={dialogsViewModel}
onDialogSelectHandler={selectDialogActions}
additionalSettings={{
withoutHeader: false,
themeHeader: theme,
themePreview: theme,
useSubHeader: true,
useUpHeader: true,
}}
/>
}
dialogMessagesView={
<MessagesView
// ...
/>
}
dialogInfoView={
<DialogInformation
// ...
/>
}
/>
Customization
Components HeaderDialog and PreviewDialog are sensitive to the theme used.
HeaderDialog component - the header of the dialog list, uses Search icon, wich you can find on path src/Presentation/components/UI/svgs/Icons/Navigation/Search, and New Chat icon, wich you can find on path src/Presentation/components/UI/svgs/Icons/Actions/NewChat.
HeaderDialog component uses a set of theme elements defined by the following methods in the UiKitTheme interface: divider(), mainBackground(), mainText(), fontFamily(), mainElements().
The styles specific to this component are defined in the HeaderDialog.scss file.
PreviewDialog component - the preview of each available dialog, uses PublicChannel, GroupChat, User, and EditDots icons. All icons are located in the directory src/Presentation/components/UI/svgs/Icons.
PreviewDialog component uses a set of theme elements defined by the following methods in the theme UiKitTheme interface: mainElements(), mainBackground(), disabledElements(), secondaryText(), inputElements(), mainText(), divider(), chatInput(), fieldBorder().
The styles specific to this component are defined in the PreviewDialog.scss file.
The Dialog component has the following list of properties:
Property name | Type | Description |
---|---|---|
header | React.ReactNode | This field allows passing a React element as the content for the main header of the chat dialog list. |
upHeaderContent | React.ReactNode | This field allows passing a React element as the content for the upper header of the chat dialog list. |
subHeaderContent | React.ReactNode | This field allows passing a React element as the content for the additional subheader of the chat dialog list. |
dialogsViewModel | DialogsViewModel | This field corresponds to the dialog view model (DialogsViewModel) responsible for handling data and managing the state of the chat dialog list, following the MVVM pattern. |
onDialogSelectHandler | Object function | This field represents a function (Handler) to handle the event of selecting (highlighting) a dialog from the chat dialog list. It might take an object of type DialogEntity representing the selected dialog. |
additionalSettings | Object | This field represents additional settings (DialogsComponentSettings) for the chat dialog list component that we have already discussed. / type DialogsComponentSettings = { themeName?: ThemeNames; withoutHeader?: boolean; useSubHeader?: boolean; useUpHeader?: boolean; themeHeader?: UiKitTheme; themePreview?: UiKitTheme; }; / |
Note on DialogsComponentSettings:
themeName?: ThemeNames: This field optionally indicates the theme name (ThemeNames) that can be associated with the components inside the chat dialog list.
withoutHeader?: boolean: If this field is set to true, the chat dialog list component will not display the main header.
useSubHeader?: boolean: If this field is set to true, the chat dialog list component will use an additional subheader.
useUpHeader?: boolean: If this field is set to true, the chat dialog list component will use an upper header.
themeHeader?: UiKitTheme: This field optionally indicates the theme (UiKitTheme) for styling the main header of the chat dialog list.
themePreview?: UiKitTheme: This field optionally indicates the theme (UiKitTheme) for styling the preview of the chat dialog list.
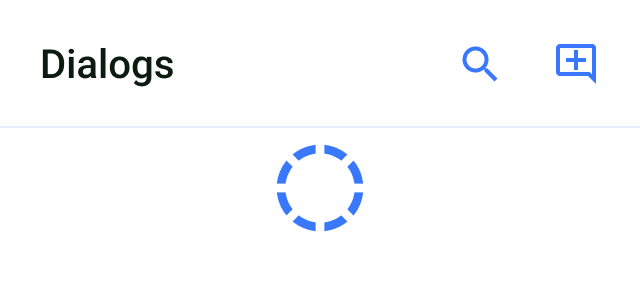
Usage
Create New Dialog Screen
The dialog list screen shows the complete list of group and private dialogs of which the current user is a member.
After following the steps described in the Get started section, you can display and manage the list of dialogs.
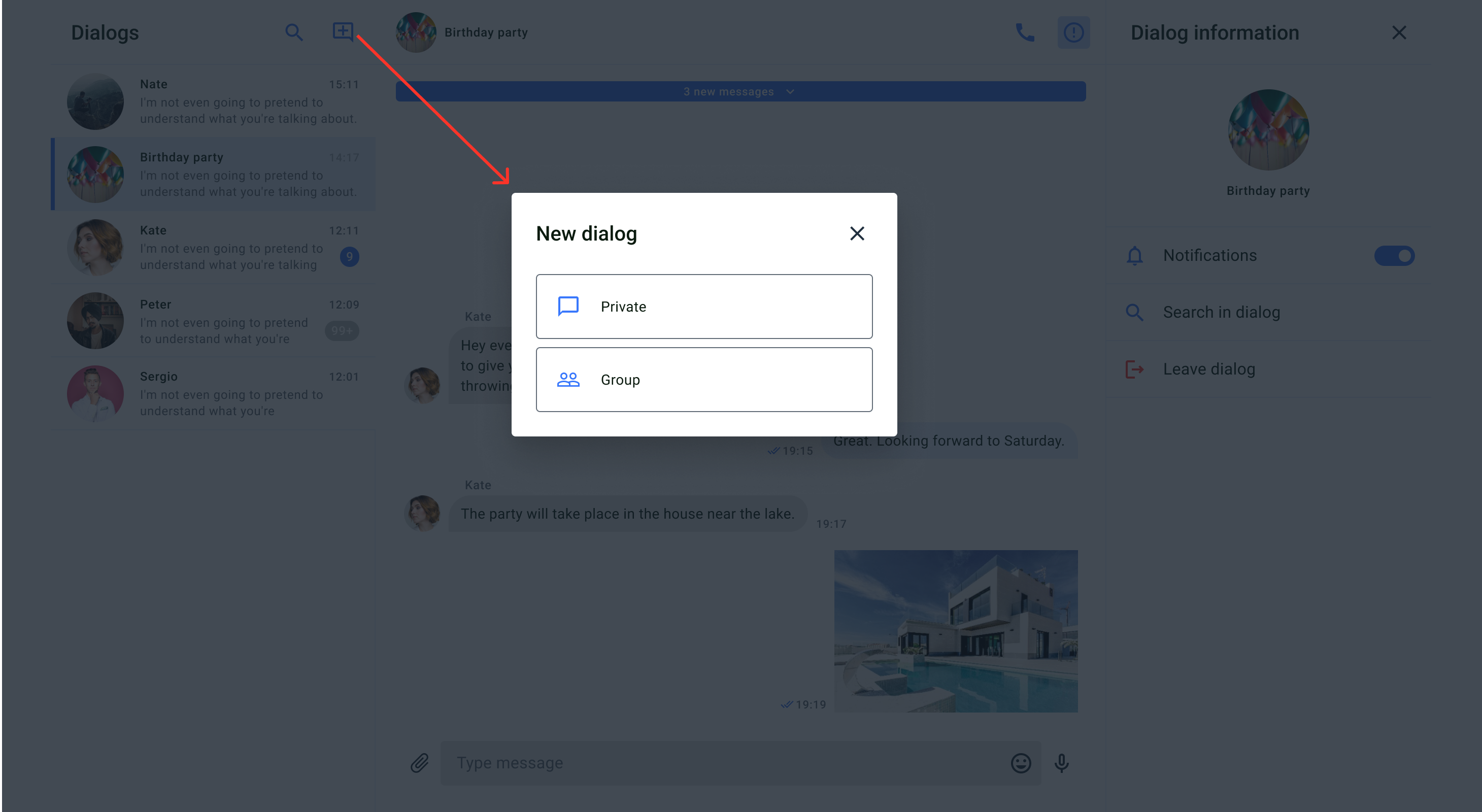
Coming Soon: Public Dialog Creation in Future UI Kit Updates
The ability to create public dialogs will be added in future updates of the UI Kit.
Dialog name screen
The dialog name screen is used in the group dialog creation flow. And allows you to set a name and photo for a group dialog.
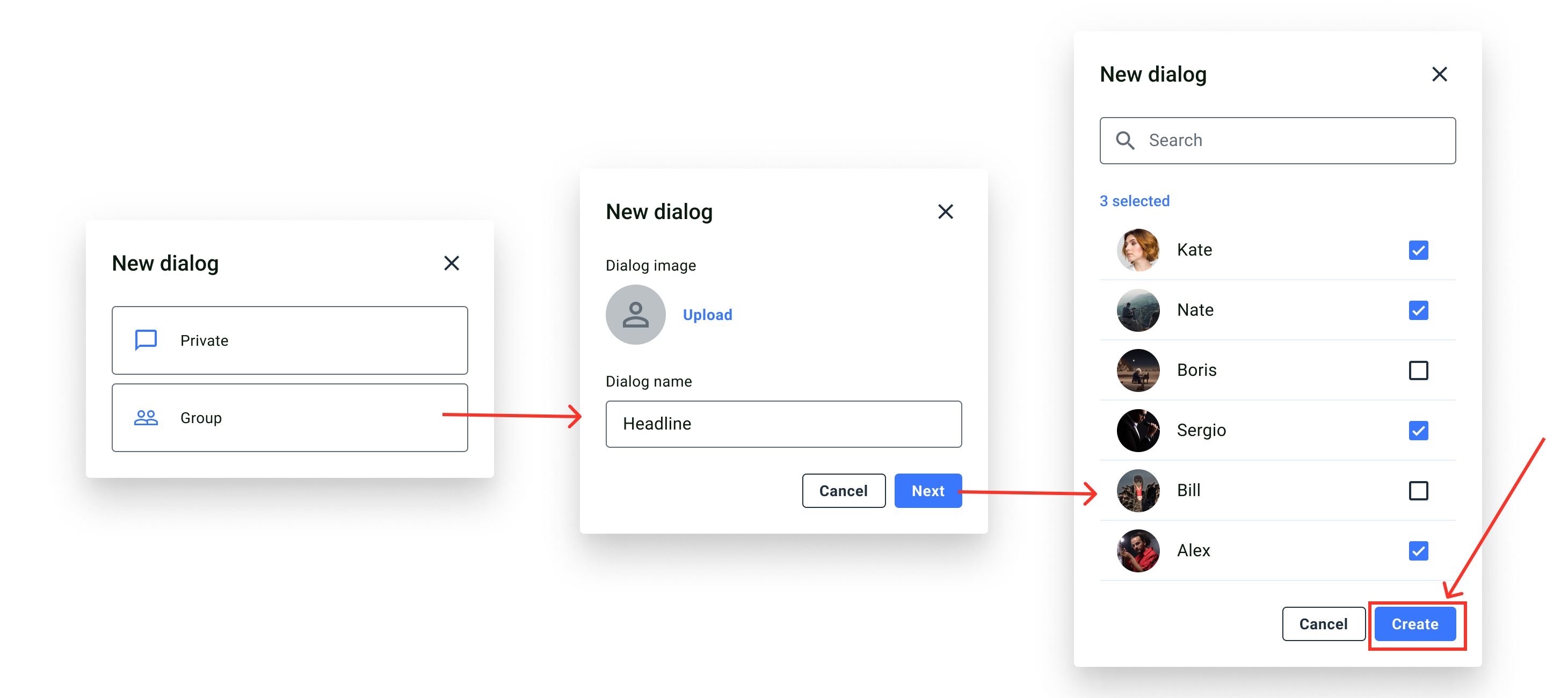
Customization
You can customize the "New dialog" pop-up window in the CreateDialog.scss file to modify various aspects of the screen. The UserList.scss file with classes allows you to customize the main list components.
User list screen
The user list screen displays available users that can be selected to create a group or private dialog.
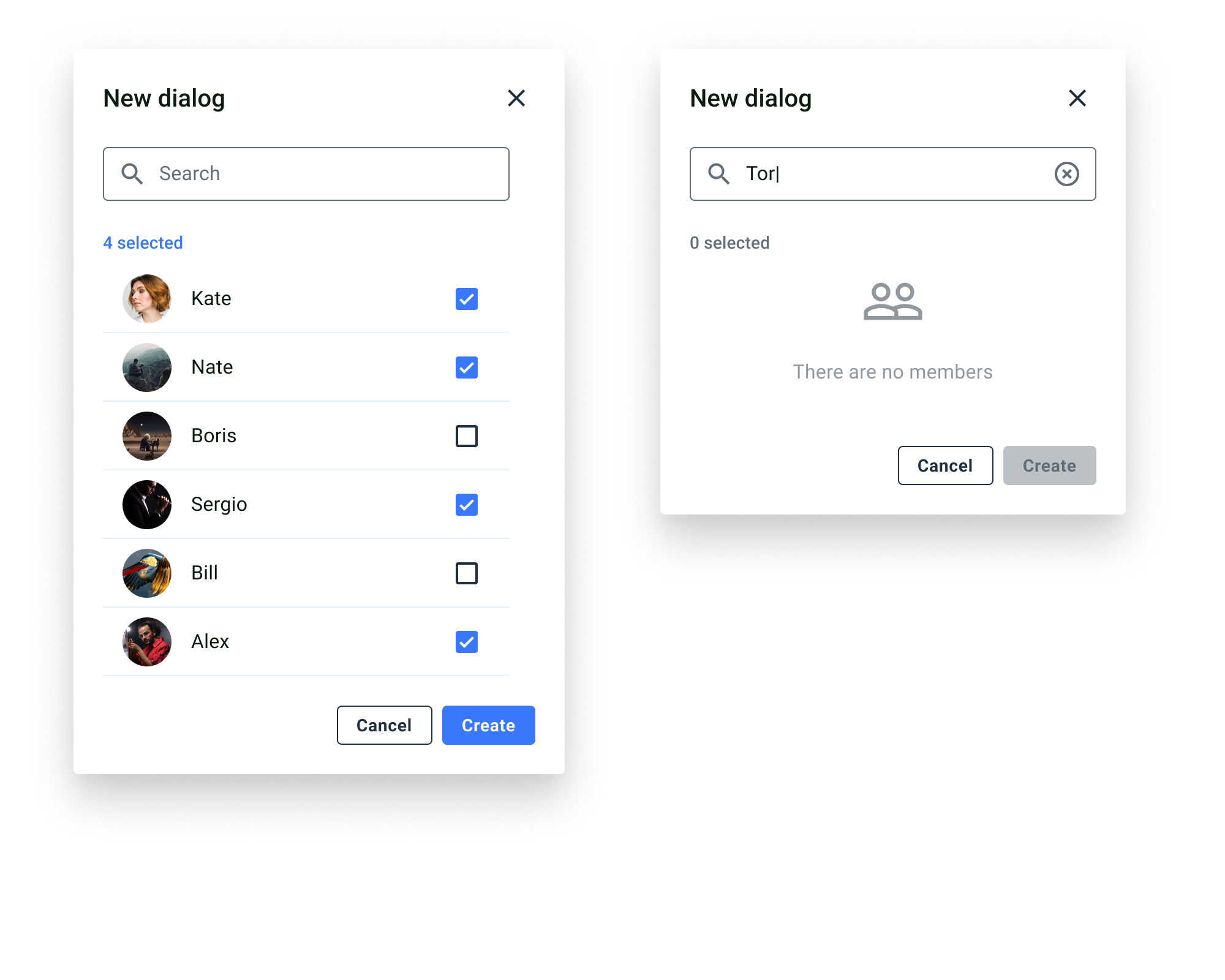
Group and private chats screen
A group chat screen allows close interactions between a limited number of users. To join the dialog, one of the members needs to add you to the dialog. A private chat screen allows close interaction between two users. To participate in this type of dialog, you can create a dialog with your opponent or your opponent can create a dialog with you.
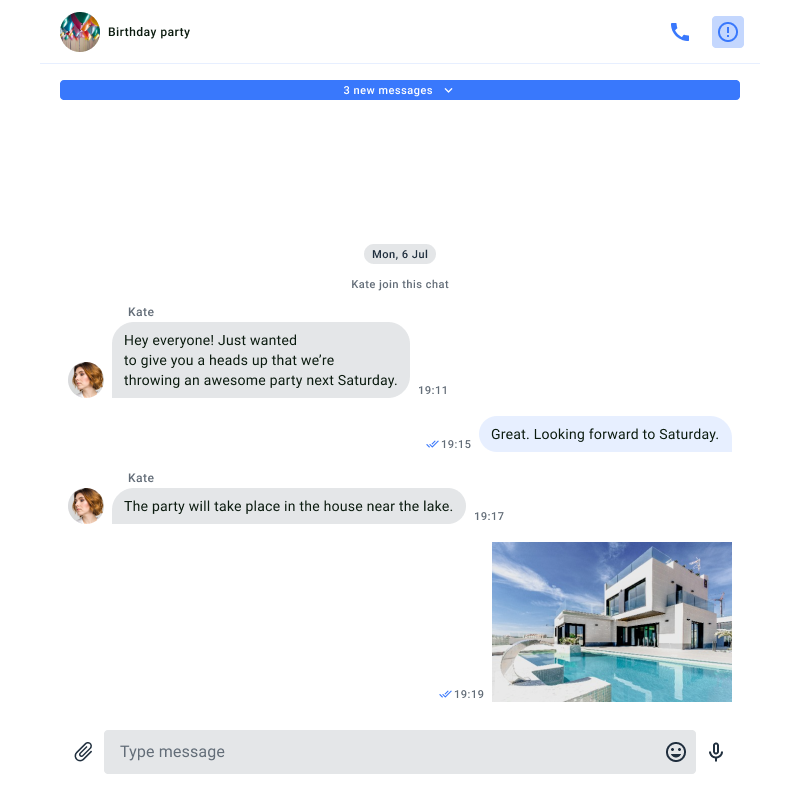
Screen structure
The chat screen consists of two components: the header and messages.
It utilizes two models: DialogsViewModel, which provides information about the current dialog, such as its name, list of participants, and message history, and MessagesViewModel, which describes the collection of messages for a specific dialog.
You can access the DialogsViewModel using the custom hook useDialogsViewModel(). Similarly, you can access the MessagesViewModel using the custom hook useMessagesViewModel().
The code for these hooks is located in the following files: src/Presentation/Views/Dialogs/useDialogsViewModel.ts and src/Presentation/components/UI/Dialogs/MessagesView/useMessagesViewModel.ts.
Header
The header component contains the name and the photo of the dialog. Also, the header contains a default button in the upper right corner that allows you to open the group chat info screen.
Messages
The message list component shows a list of all messages exchanged in the chat, in chronological order. The list displays both text and file messages, and messages sent by the current user are different from messages sent by other participants in the dialog.
Send Message
The send message component is where the user can either enter a text message or send the message as a file by importing a file, image, or video.
Usage
The MessageView component, designed to display the list of dialogs (chats), consists of three logical parts: the HeaderMessages component (header of the message list), components for displaying messages (VideoAttachmentComponent, AudioAttachmentComponent, ImageAttachmentComponent, HighLightLink), and the component for sending messages.
While you cannot create your own custom component for the header of the message list, you can place your own components above and below it. This allows you to add your logo or other custom elements to the UI Kit interface.
All parts of the MessageView component can be customized using themes.
In future versions of the UI Kit, you will also be able to set your own components for sending messages and displaying the messages themselves.
Customization
All parts of the MessageView component are sensitive to the theme used.
The HeaderMessages component, which serves as the header of the dialog list, uses icons:
- To display the type of dialog: GroupChat, UserAvatar, PublicChannel;
- To display dialog properties: InformationFill;
- To start a video call: Phone;
The icons are located in the directory: src/Presentation/components/UI/svgs/Icons
The HeaderMessages component uses a set of theme elements defined by the following methods in the UiKitTheme interface: divider(), secondaryText(), secondaryBackground().
The specific styles for this component are defined in the HeaderMessages.scss file.
Other parts of the MessageView component responsible for displaying messages (VideoAttachmentComponent, AudioAttachmentComponent, ImageAttachmentComponent, HighLightLink) use the icons SentStatusIcon, ViewedDelivered, ImagePlay, AudioFile, ImageEmpty.
The icons are located in the directory: src/Presentation/components/UI/svgs/Icons.
The MessageView component uses a set of theme elements defined by the following methods in the UiKitTheme interface: mainElements(), mainBackground(), disabledElements(), secondaryText(), inputElements(), mainText(), divider(), chatInput(), fieldBorder().
The specific styles for this component are defined in the MessageView.scss file.
Each of the nested components also has its own styles specified in separate files, which customize the parent component's styles. For example, the VideoAttachmentComponent component responsible for displaying the appearance of video files is styled in the VideoAttachmentComponent.scss file.
Similarly, the AudioAttachmentComponent component responsible for displaying and playing audio files is styled in the AudioAttachmentComponent.scss file.
The ImageAttachmentComponent component, responsible for displaying the appearance of images, is styled in the ImageAttachmentComponent.scss file.
The HighLightLink component, responsible for displaying the appearance of links in messages, is styled in the HighLightLink.scss file.
The MessageView component has the following list of properties:
Property name | Type | Description |
---|---|---|
upHeaderContent | React.ReactNode | This field allows passing a React element as the content for the upper header of the chat dialog list. |
subHeaderContent | React.ReactNode | This field allows passing a React element as the content for the additional subheader of the chat dialog list. |
dialogsViewModel | DialogsViewModel | This field represents the dialogs view model (DialogsViewModel) that manages the data and state of the chat messages list. In the context of the MVVM pattern, the view model handles the logic related to data and interaction with the user interface components. |
onDialogInformationHandler | Object function | This field represents the handler function (Handler) for the event of obtaining information about a dialog. It may not take any arguments and performs specific actions when requesting information about a dialog. |
theme | UiKitTheme | This field represents the theme (UiKitTheme) for styling the chat messages list component. In an MVVM pattern-based application, themes are often used to manage the style and appearance of the user interface. |
AIAnswerToMessage | AIWidget | In versions 0.2.0 and later, you can integrate AI functionality based on OpenAI features. This field allows you to specify your own AI Widget for generating quick responses to messages. For more details, please refer to the following AI Feature section of documentation. |
You can use AI features in UI Kit version 0.2.0 or later
Please, look at this section of our documentation.
To use the Dialogs component in the project, you need to add it to the dialogsView section of the DesktopLayout layout component. We wrote about the DesktopLayout component in the Core Components section - here is the link. Below is a snippet of the DesktopLayout markup that uses the Dialogs component.
<DesktopLayout
theme={theme}
dialogsView={
<DialogsComponent
// ...
/>
}
dialogMessagesView={
<MessagesView
subHeaderContent={<CompanyLogo />}
upHeaderContent={<CompanyLogo />}
dialogsViewModel={dialogsViewModel}
onDialogInformationHandler={informationOpenHandler}
theme={theme}
/>
}
dialogInfoView={
<DialogInformation
// ...
/>
}
/>
Dialog information screen
The group chat info screen allows configuring the settings of the group dialog. If you owner you can customize settings including dialog name and dialog image, you can also leave the dialog.
The MessageView component uses two models: DialogsViewModel and UsersListViewModel. DialogsViewModel is used to retrieve information about the current dialog, such as its title, list of participants, and message list. UsersListViewModel describes the collection of messages for a specific dialog. You can obtain the DialogsViewModel using the custom hook useDialogsViewModel() and the UsersListViewModel using the custom hook useUsersListViewModel().
The code for these hooks is located in the following files: src/Presentation/Views/Dialogs/useDialogsViewModel.ts and src/Presentation/components/UI/Dialogs/DialogInformation/UsersList/useUsersListViewModel.ts.
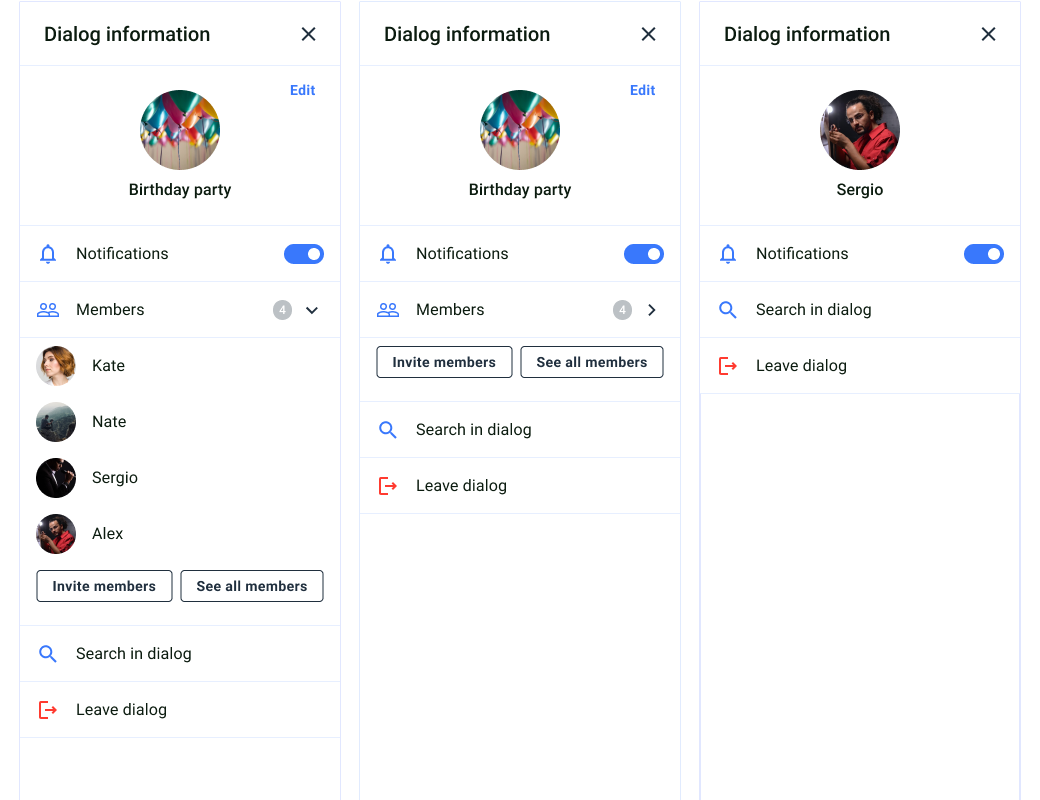
Screen structure
The group chat info screen consists of two components: header, dialog info and dialog members list. We want to draw your attention that the list of participants is only available for group dialogs and is not displayed for private dialogs.
Note:
The Notification component will be available in the upcoming UI Kit updates.
Header
The header component contains a screen title. If you dialog owner, the header includes a default button "Edit" in the upper right corner that allows you to open the flow for updating the name and image of the dialog.
Dialog info
The dialog info component shows the dialog's cover image and name by default. The view of the channel information is created and customized in the DialogInfoComponent.sccs file.
dialog members list
The "Members List" component - MembersList - displays the complete list of users who are participants in a group dialog. The display and appearance of member information can be customized in the MembersList.scss file. By default, the component shows profile images and names of all participants in the group dialog. We will examine the appearance and functionality of this component separately below.
Usage
The DialogInformation component, used to display information about dialogs (chats), consists of several sub-components: HeaderDialogInformation (header of the information window), the dialog information itself, which allows changing the dialog name and its icon, and the list of dialog participants. The MembersList component displays the participants of the dialog, while the InviteMembers component lists users who can be invited to the dialog. Both of these components are not available for private dialogs.
You cannot create your own custom component for the header of the dialog information, but you can place your own component above and below it. This allows you to add your logo to the UI Kit elements. However, the DialogInformation component can only be customized through themes.
Customization
All components inside DialogInformation are sensitive to the theme used.
The HeaderDialogInformation component, which serves as the header of the dialog information, utilizes the following icons: UserAvatar, GroupChat, PublicChannel, NotifyOn, and Search.
The icons are located in the directory:src/Presentation/components/UI/svgs/Icons.
The specific styles for the DialogInformation component are defined in the file DialogInformation.scss.
The components MembersList and InviteMembers, which are used to preview each available dialog, use the MainButton and SwitchButton components as buttons. These components are styled in the files MainButton.scss and SwitchButton.scss.
The MembersList and InviteMembers components use styles defined for them in the files MembersList.scss and InviteMembers.scss.
The DialogInformation component has the following list of properties:
Property name | Type | Description |
---|---|---|
theme | UiKitTheme | This field represents the theme (UiKitTheme) for styling the dialog information component. Themes allow controlling the style and appearance of the user interface in an application built using the MVVM pattern. |
upHeaderContent | React.ReactNode | This field allows passing a React element as the content for the upper header of the chat dialog list. |
subHeaderContent | React.ReactNode | This field allows passing a React element as the content for the additional subheader of the chat dialog list. |
dialogsViewModel | DialogsViewModel | This field represents the dialog view model (DialogsViewModel), which manages data and the state of the dialog list in the chat. In the context of the MVVM pattern, the view model handles logic related to data and interaction with user interface components. |
onCloseDialogInformationHandler | Object function | This field represents a handler function for the event of closing dialog information. This function performs specific actions when the window with dialog information is closed. |
dialog | DialogEntity | This field represents the dialog entity (DialogEntity), which contains information about the selected dialog, such as its ID, name, participants, and other properties that may be relevant for displaying dialog information. |
To use the DialogInformation component in the project, you need to add it to the dialogsView section of the DesktopLayout layout component. We wrote about the DesktopLayout component in the Core Components section - here is the link. Below is a snippet of the DesktopLayout markup that uses the Dialogs component.
<DesktopLayout
theme={theme}
dialogsView={
<DialogsComponent
// ...
/>
}
dialogMessagesView={
<MessagesView
// ...
/>
}
dialogInfoView={
<DialogInformation
subHeaderContent={<CompanyDescription />}
upHeaderContent={<CompanyLogo />}
dialog={selectedDialog.entity}
dialogViewModel={dialogsViewModel}
onCloseDialogInformationHandler={informationCloseHandler}
/>
}
/>
Members screen
A members screen is a complete list of users that are members of a group dialog. The dialog member list is created through MembersList components.
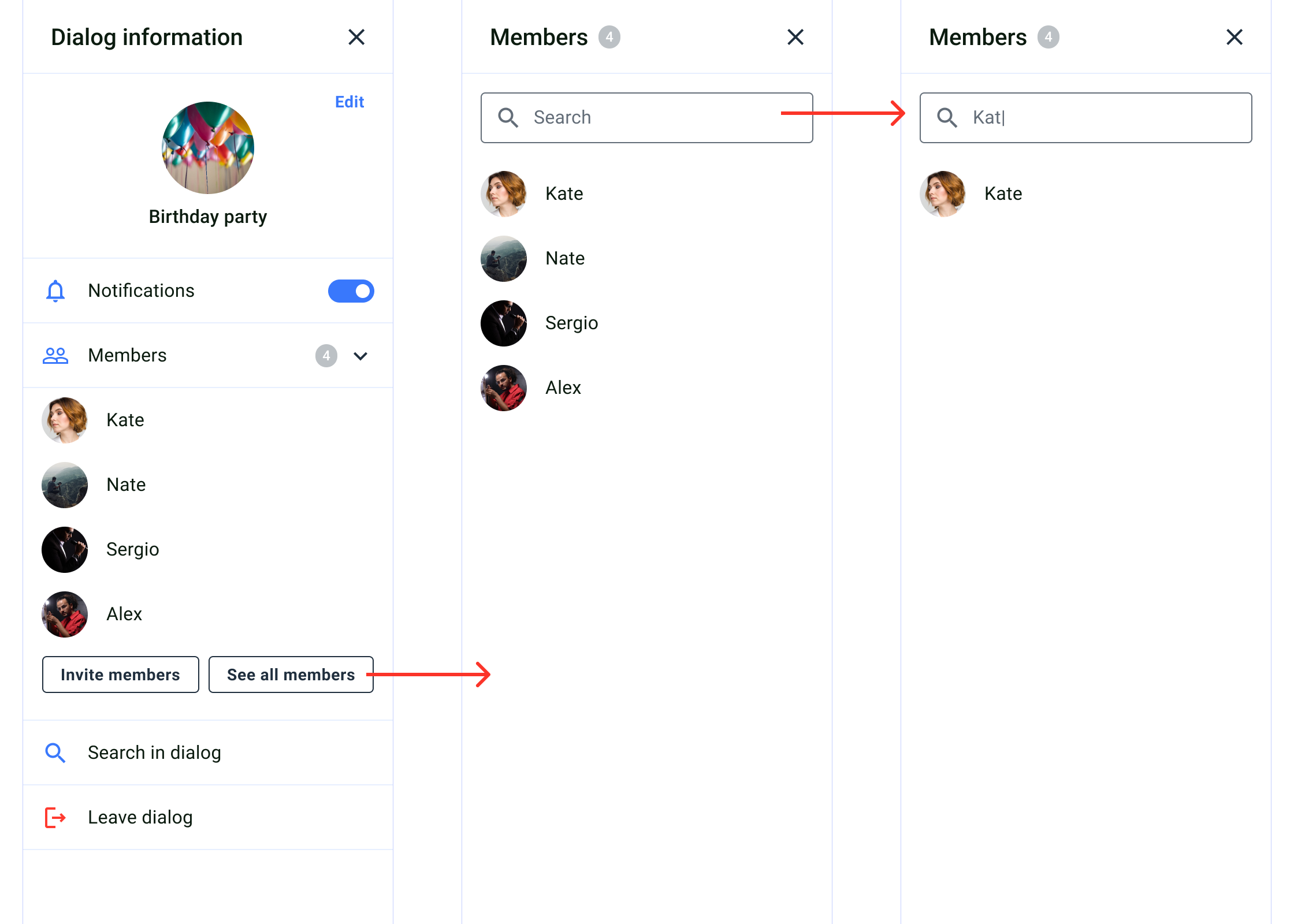
Add members screen
The add members screen allow invite new members to the group dialog. The dialog member list is created through MembersList or InviteMembers components. All users are listed through the pagination, and members who already belong to the dialog cannot be shown.
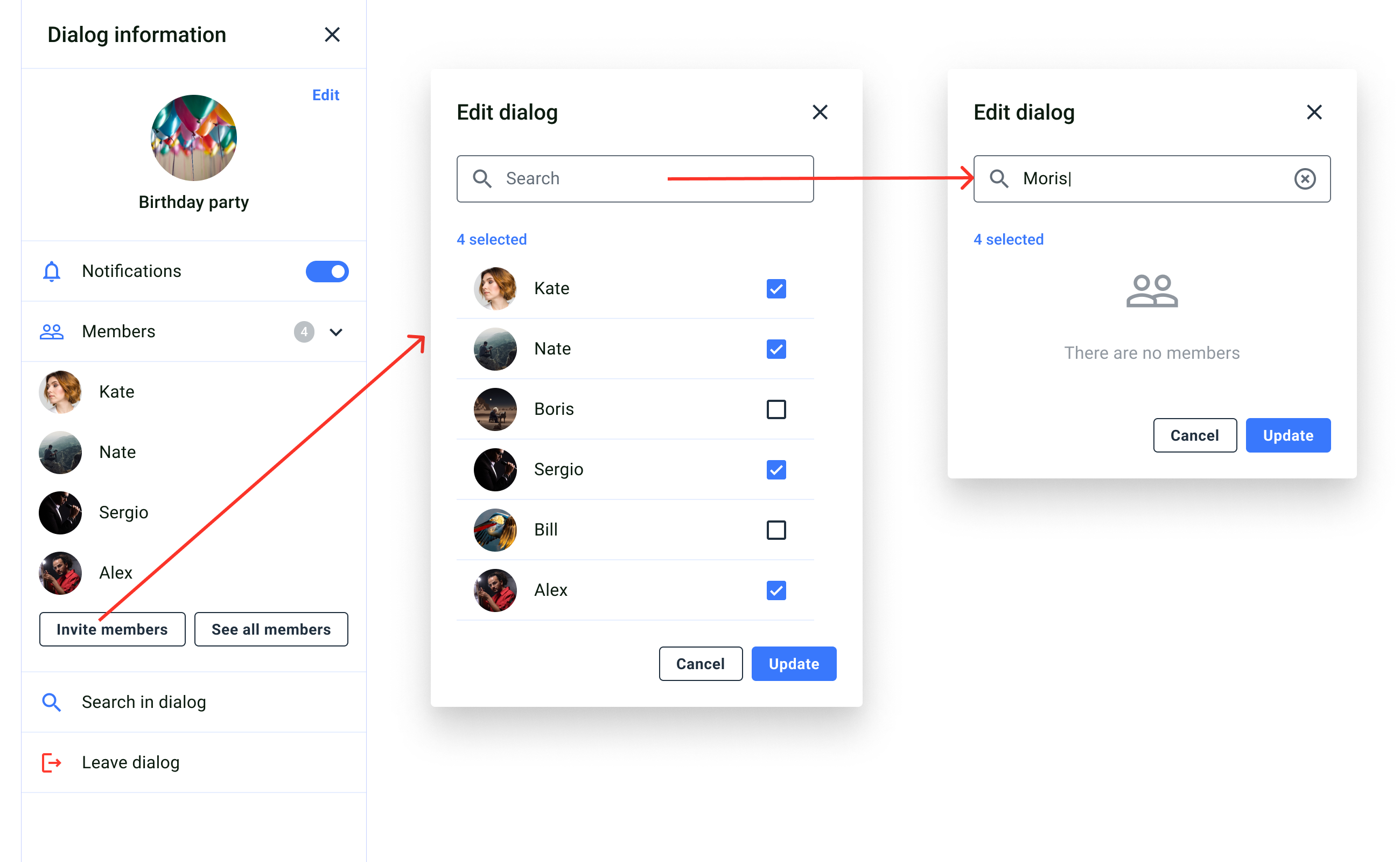
Customization
The MembersList and InviteMembers components use styles defined specifically for them in the files MembersList.scss and InviteMembers.scss, respectively.
Updated 12 months ago