Dialogs
Learn how to create and manage dialogs.
Before you begin
- Register a QuickBlox account. This is a matter of a few minutes and you will be able to use this account to build your apps.
- Configure QuickBlox SDK for your app. Check out our Setup page for more details.
- Create a user session to be able to use QuickBlox functionality. See our Authentication page to learn how to do it.
- Connect to the Chat server. See our Connection page to learn how to do it.
Visit our Key Concepts page to get an overall understanding of the most important QuickBlox concepts.
Dialog types
All chats between users are organized in dialogs. There are 3 types of dialogs:
- private dialog - a dialog between 2 users.
- group dialog - a dialog between the specified list of users.
- public dialog - an open dialog. Any user from your app can be joined to it.
You need to create a new dialog and then use it to chat with other users. You also can obtain a list of your existing dialogs.
Create dialog
To create a private dialog, you need to set the ID of the opponent you want to create a chat with.
List<int> occupantsIds = [89987878, 98987887];
String dialogName = "someone chat";
int dialogType = QBChatDialogTypes.CHAT;
try {
QBDialog? createdDialog = await QB.chat.createDialog(occupantsIds, dialogName, dialogType: dialogType);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
To create a group dialog for a predefined number of occupants, you need to set the IDs of opponents you want to create a chat with.
List<int> occupantIds = [234234324, 3243243];
String dialogName = "Group Chat";
String dialogPhoto = "some photo url";
int dialogType = QBChatDialogTypes.GROUP_CHAT;
try {
QBDialog? createdDialog = await QB.chat.createDialog(occupantIds, dialogName, dialogType: dialogType, dialogPhoto: dialogPhoto);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
It's possible to create a public dialog, so any user from your application can be joined to it. There is no list of occupants. This dialog is open for everybody.
List<int> occupantIds = [234234324, 3243243];
int dialogType = QBChatDialogTypes.PUBLIC_CHAT;
String dialogName = "Public Chat";
try {
QBDialog? createdDialog = await QB.chat.createDialog(occupantIds, dialogName, dialogType: dialogType);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
The createDialog()
method accepts the following arguments:
Argument | Required | Description |
---|---|---|
occupantIds | yes | A list of opponents IDs. - If the occupantsIds array is empty and type is not provided, the public dialog is created.- If the occupantsIds has a single user and type is not provided, the private dialog is created.- If the occupantsIds has more then one userId and type is not provided, a group dialog is created. |
dialogName | yes | The name of the dialog. Required only for group and public dialog types. Not needed for private dialog. |
dialogType | no | A type of the dialog. Possible values: QBChatDialogTypes.CHAT , QBChatDialogTypes.GROUP_CHAT or QBChatDialogTypes.PUBLIC_CHAT .By default, the public dialog is created. |
dialogPhoto | no | A url of the image. Can be a link to a file in Content module, Custom Objects module or just a web link. Must be a String. |
Join dialog
Before you start chatting in a group or public dialog, you need to join it by calling the joinDialog()
method. If you've successfully joined the dialog, you can send/receive messages in real-time. See this section to learn how to send/receive messages.
String dialogId = "fdb5f5a28388a64aba5b2f57570b13f827012bba";
try {
await QB.chat.joinDialog(dialogId);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
To check if you have already joined the dialog, you should call the method isJoinedDialog()
String dialogId = "fdb5f5a28388a64aba5b2f57570b13f827012bba";
try {
bool isJoinedDialog = await QB.chat.isJoinedDialog(dialogId);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
Let's see, how the joinDialog()
method is used with regard to the dialog type.
Capabilities | Public | Group | Private |
---|---|---|---|
Join | ✓ | ✓ | ✗ |
You can join a group dialog only if your user ID is present in the
occupantIDs
array, in the dialog model.Your user ID is added to the
occupantIDs
array if you create a dialog or you are added to the dialog by the other user. See this section to learn how to add occupants to the group dialog.
Leave dialog
You can leave the group and public dialog by calling the leaveDialog()
method. If the dialog is left, you can't send/receive messages. To be able to receive/send messages, you need to join it.
String dialogId = "fdb5f5a28388a64aba5b2f57570b13f827012bba";
try {
await QB.chat.leaveDialog(dialogId);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
Capabilities | Public | Group | Private |
---|---|---|---|
Leave | ✓ | ✓ | ✗ |
When a group dialog is left, your user ID is removed
occupantIds
array, in the dialog model. As a result, the dialog is removed from the list of dialogs and you won't have access to the chat history.To remove a dialog for all users, use the
deleteDialog()
method. See this section to learn how to delete the dialog completely for all users.
Retrieve list of dialogs
It's common to request all your dialogs on every app login. The request below will return private, group, and public dialogs containing test value
in their names, sorted in ascending order by the QBChatDialogFilterFields.LAST_MESSAGE_DATE_SENT
field, and limited to 100 dialogs on the page with 25 dialogs skipped at the beginning.
QBSort sort = QBSort();
sort.field = QBChatDialogSorts.LAST_MESSAGE_DATE_SENT;
sort.ascending = true;
QBFilter filter = QBFilter();
filter.field = QBChatDialogFilterFields.NAME;
filter.operator = QBChatDialogFilterOperators.CTN;
filter.value = "test value";
int limit = 100;
int skip = 25;
try {
List<QBDialog?> dialogs = await QB.chat.getDialogs(sort: sort, filter: filter, limit: limit, skip: skip);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
Argument | Required | Description |
---|---|---|
sort | no | Specifies sorting criteria for the field. |
filter | no | Specifies filtering criteria for the field. |
limit | no | Limit search results to N records. Useful for pagination. Default value: 100. |
skip | no | Skip N records in search results. Useful for pagination. Default (if not specified): 0. |
If you want to retrieve only dialogs updated after some specific date time and order the search results, you can apply operators. This is useful if you cache dialogs somehow and do not want to obtain the whole list of your dialogs on every app start. Thus, you can apply search and sort operators to list dialogs on the page so that it is easier to view specific dialogs.
Search operators
You can use search operators to get more specific search results. The request below will return all dialogs containing the flutter_chat
in their names.
try {
QBFilter filter = QBFilter();
filter.field = QBChatDialogFilterFields.NAME;
filter.operator = QBChatDialogFilterOperators.CTN;
filter.value = "flutter_chat";
List<QBDialog?> dialogs = await QB.chat.getDialogs(filter: filter);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
Here are the search operators that you can use to search for the exact data that you need.
Search operators | Applicable to types | Applicable to fields | Description |
---|---|---|---|
lt | number, string, date | last_message_date_sent, created_at, updated_at | Less Than operator. |
lte | number, string, date | last_message_date_sent, created_at, updated_at | Less Than or Equal to operator. |
gt | number, string, date | last_message_date_sent, created_at, updated_at | Greater Than operator. |
gte | number, string, date | last_message_date_sent, created_at, updated_at | Greater Than or Equal to operator. |
ne | number, string, date | _id, name, last_message_date_sent | Not Equal to operator. |
in | number, string, date | type, last_message_date_sent, name | IN array operator. |
nin | number, string, date | last_message_date_sent | IN array operator. |
all | number | occupants_ids | ALL are contained in array. |
ctn | string | name | All records that contain a particular substring. |
Sort operators
You can use sort operators to order the search results. The request below will return a list of dialogs sorted by the QBChatDialogSorts.LAST_MESSAGE_DATE_SENT
field in descending order.
try {
QBSort sort = QBSort();
sort.field = QBChatDialogSorts.LAST_MESSAGE_DATE_SENT;
sort.ascending = false;
List<QBDialog?> dialogs = await QB.chat.getDialogs(sort: sort);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
Here are the sort options that you can use to order the search results.
Sort options | Applicable to types | Applicable to fields | Description |
---|---|---|---|
ascending | All types | id, created_at, name, last_message_date_sent | Sort results in ascending order by setting the ascending as true . |
descending | All types | id, created_at, name, last_message_date_sent | Sort results in descending order by setting the ascending as false . |
Update dialog
You can update the information for a private, group, and public dialog.
String dialogId = "adfpo443ldfJLsdjdsf324lkjer";
String dialogName = "Some Chat";
String dialogPhoto = "some photo url";
try {
QBDialog? updatedDialog = await QB.chat.updateDialog(dialogId, dialogName: dialogName, dialogPhoto: dialogPhoto);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
Argument | Required | Description |
---|---|---|
dialogId | yes | The ID of the dialog. |
dialogName | no | A name of the dialog. |
dialogPhoto | no | A url of the image. Should be a String. |
Let's see what capabilities a particular user role has with regard to the dialog type.
Capabilities | Public dialog | Group dialog | Private dialog |
---|---|---|---|
Update a dialog name | Owner | Owner | ✗ |
Update a photo | Owner | Owner | ✗ |
Add occupants
Set the addUsers
argument to add occupants to the dialog. As a result, the ID of the opponent will be added to the occupantIds
array.
String dialogId = "fdb5f5a28388a64aba5b2f57570b13f827012bba";
List<int> addUsers = [234234111, 32432777];
try {
QBDialog? updatedDialog = await QB.chat.updateDialog(dialogId, addUsers: addUsers);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
Let's see what capabilities a particular user role has with regard to the dialog type.
Capabilities | Public dialog | Group dialog | Private dialog |
---|---|---|---|
Add other users | ✗ | Owner, Occupant | ✗ |
Remove occupants
Set the removeUsers
argument to remove occupants from the dialog. As a result, the ID of the opponent will be removed from the occupantIds
array.
String dialogId = "fdb5f5a28388a64aba5b2f57570b13f827012bba";
List<int> removeUsers = [234234888, 3243999];
try {
QBDialog? updatedDialog = await QB.chat.updateDialog(dialogId, removeUsers: removeUsers);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
Capabilities | Public dialog | Group dialog | Private dialog |
---|---|---|---|
Remove other users | ✗ | Owner | ✗ |
Remove yourself | ✗ | Owner, Occupant | ✗ |
Delete dialog
Delete a dialog for all users using the request below. When deleting a group dialog, all user IDs will be removed from the occupantIds
array in the dialog model. You can also delete multiple dialogs in a single request.
To delete a dialog for yourself, just leave the dialog. See this section for more information.
String dialogId = "fdb5f5a28388a64aba5b2f57570b13f827012bba";
try {
await QB.chat.deleteDialog(dialogId);
} on PlatformException catch (e) {
// some error occurred, look at the exception message for more details
}
Let's see what capabilities a particular user role has with regard to the dialog type.
Capabilities | Public | Group | Private |
---|---|---|---|
Delete a dialog for all users | Owner | Owner | Owner |
Resources
A sequence of steps a user takes to start a dialog by moving through the application lifecycle.
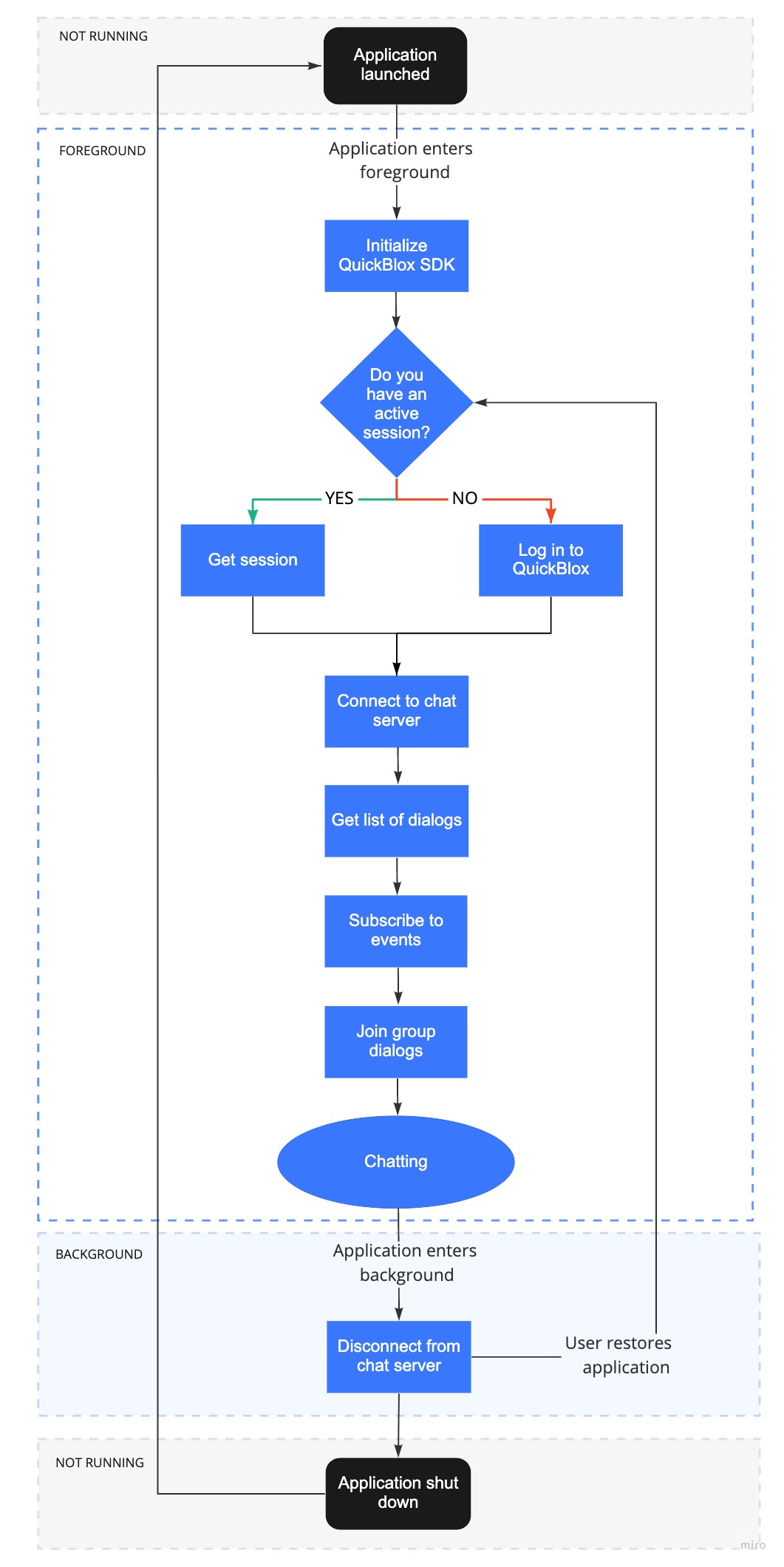
Updated 7 months ago