AI Features
AI Features base on SmartChat Assistants
Overview
Starting from version 2.17.0 of JavaScript SDK, the AI functionality is enabled and based on our SmartChat Assistants. The QuickBlox JavaScript SDK provides a range of features to enhance the chat experience. With essential messaging functionalities such as answer assistant, users can engage in more interactive conversations.
Supported features
Name | Description |
---|---|
AI Assist | Provides answers based on chat history to selected message. |
AI Translate | Provides translation based on chat history to selected incoming message. |
Requirements
The minimum requirements for using AI features are:
- JS QuickBlox SDK v2.17.0
- Quickblox account with activated SmartChat Assistants
Visit our Key Concepts page to get an overall understanding of the most important QuickBlox concepts.
Visit our Smart Chat Assistant overview page to get an overall understanding of the most important SmartChat Assistants concepts.
Before you begin
- Register a QuickBlox account. This is a matter of a few minutes and you will be able to use this account to build your apps.
- Configure QuickBlox SDK for your app. Check out our Setup page for more details.
- Create a user session to be able to use QuickBlox functionality. See our Authentication page to learn how to do it.
- Create or update your SmartChat Assistant. See our Smart Chat Assistant documentation to learn how to do it.
Review your SmartChat Assistant
To get ID of SmartChat Assistant to use it as smartChatAssistantId
param follow the steps below:
- Navigate to the Dashboard => YOUR_APP => SmartChat Assistant page.
- Choose the ID of the SmartChat Assistant you want to update and click on it.
- Edit the SmartChat Assistant settings.
- Click the Save button to save changes.
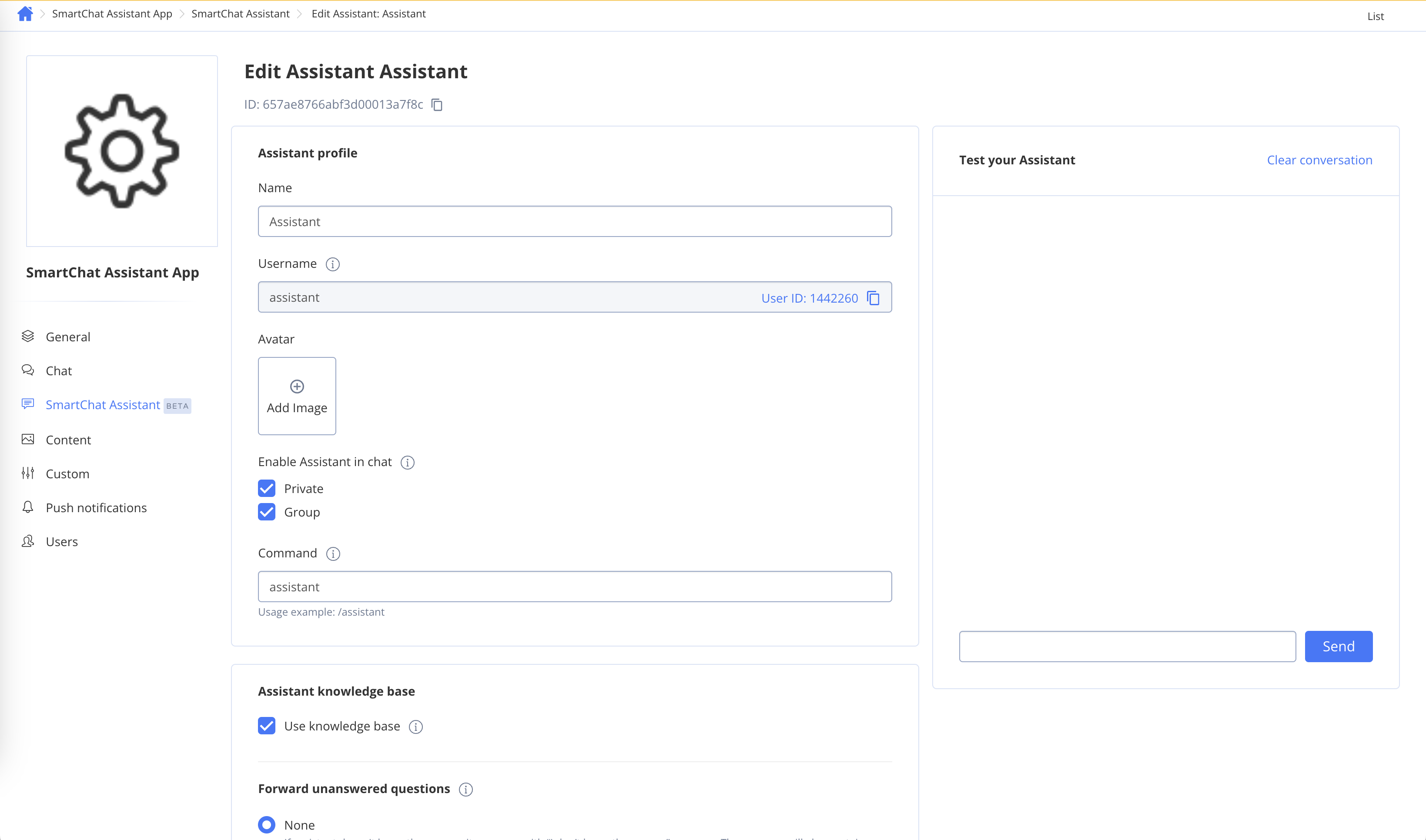
AI Assist Answer
QuickBlox provides answer assistant functionality that helps users effortlessly send various answers considering chat history.
How to use Assist Answer
var smartChatAssistantId = '6633a1300fea600001bd6e71';
var messageToAssist = 'Where is my order?';
var history = [
{role: "user", message: "Hello"},
{role: "assistant", message: "Hi"}
];
QB.ai.answerAssist(smartChatAssistantId,
messageToAssist,
history,
function (error, response) {
if (error) {
console.error('QB.ai.answerAssist: Error:', error);
} else {
console.log('QB.ai.answerAssist: Response:', response.answer);
}
});
Parameter name | Type | Description |
---|---|---|
smartChatAssistantId | String | This field should hold your actual Smart Chat Assistant ID that you'll receive from the Quickblox account. This ID is used to authenticate your requests to the AI service. |
messageToAssist | String | Message you want to get answer for. |
history | Array of Object | Conversation history. Used to add context. Each object of array should have the two fields: 'role' and 'message'. The field role should contains one of next values: 'user' or 'assistant'. The field message should be a string with chat message. |
callback | function | The callback function. |
AI Translate
QuickBlox offers translation functionality that helps users easily translate text messages in chat, taking into account the context of the chat history.
How to use AI Translate
var smartChatAssistantId = '6633a1300fea600001bd6e71';
var textToTranslate = 'Hola!';
var languageCode = 'en';
QB.ai.translate(smartChatAssistantId,
textToTranslate,
languageCode,
function (error, response) {
if (error) {
console.error('QB.ai.translate: Error:', error);
} else {
console.log('QB.ai.translate: Response:', response.answer);
}
});
Parameter name | Type | Description |
---|---|---|
smartChatAssistantId | String | This field should hold your actual Smart Chat Assistant ID that you'll receive from the Quickblox account. This ID is used to authenticate your requests to the AI service. |
textToTranslate | String | Text to translate. |
languageCode | Stringt | Translation language code. |
callback | function | The callback function. |
Updated 3 months ago