Dialogs
Learn how to create and manage dialogs.
Before you begin
- Register a QuickBlox account. This is a matter of a few minutes and you will be able to use this account to build your apps.
- Configure QuickBlox SDK for your app. Check out our Setup page for more details.
- Create a user session to be able to use QuickBlox functionality. See our Authentication page to learn how to do it.
- Connect to the Chat server. See our Connection page to learn how to do it.
Visit our Key Concepts page to get an overall understanding of the most important QuickBlox concepts.
Dialog types
All chats between users are organized in dialogs. There are 3 types of dialogs:
- private dialog - a dialog between 2 users.
- group dialog - a dialog between the specified list of users.
- public dialog - an open dialog. Any user from your app can be joined to it.
You need to create a new dialog and then use it to chat with other users. You also can obtain a list of your existing dialogs.
Create dialog
To create a private dialog, you need to set the dialog type
to 3
and ID of an opponent you want to create a chat with.
var params = {
type: 3,
occupants_ids: [56]
};
QB.chat.dialog.create(params, function(error, dialog) {});
To create a group dialog for a predefined number of occupants, you need to set the dialog type
to 2
and IDs of opponents, you want to create a chat with.
var params = {
type: 2,
occupants_ids: [56, 98, 34],
name: "Hawaii relax team"
// Photo can be a link to a file in Content module, Custom Objects module or just a web link.
// photo: ""
};
QB.chat.dialog.create(params, function(error, dialog) {});
It's possible to create a public dialog, so any user from your application can be joined to it. There is no list of occupants. This dialog is open for everybody. You just need to set the dialog type
to 1
and a name for a new dialog.
var params = {
type: 1,
name: "Blockchain trends"
// Photo can be a link to a file in Content module, Custom Objects module or just a web link.
// photo: ""
};
QB.chat.dialog.create(params, function(error, dialog) {});
Create dialog with custom parameters
A dialog can be extended with additional parameters. These parameters can be used to store additional data. Also, these parameters can be used in dialogs retrieval requests.
To start using additional parameters, create an additional schema of your parameters. This is a custom objects class. Just create an empty class with all fields that you need. These fields will be additional parameters in your dialog. See this section to learn how to create a schema using Custom Objects. Then, specify the parameters defined in the schema in a new dialog.
// you should already have created a 'CoolDialog'custom objects class
var params = {
type: 2,
name: "My friends",
data:{
"class_name":"CoolDialog",
"category":"friends"
}
};
QB.chat.dialog.create(params, function(error, dialog) {});
Set the following fields of the params
.
Field | Required | Description |
---|---|---|
type | yes | Dialog type. There tree dialog types: - type: 1 - public dialog.- type: 2 - group dialog.- type: 3 - private dialog. |
name | yes | Dialog name. |
data | yes | Specifies additional parameters in a new dialog. |
Join dialog
Before you start chatting in a group or public dialog, you need to join it by calling the join()
method. If you've successfully joined the dialog, you can send/receive messages in real-time. See this section to learn how to send/receive messages.
var dialog = "...";
var dialogJid = QB.chat.helpers.getRoomJidFromDialogId(dialog._id);
try {
QB.chat.muc.join(dialogJid, function(error, result) {});
} catch (e) {
if (e.name === 'ChatNotConnectedError') {
// not connected to chat
}
}
Argument | Required | Description |
---|---|---|
dialogJid | yes | Room JID. JID (Jabber ID) of XMPP room in the XMPP server. Empty for a privatе dialog. Generated automatically by the server after dialog creation. You can get JID from the dialog ID. The JID format is the following: <app_id>-<dialog_id>@muc.chat.quickblox.com |
function() | yes | Specifies a callback function that accepts an error and result. |
Let's see, how the join()
method is used with regard to the dialog type.
Capabilities | Public | Group | Private |
---|---|---|---|
Join | ✓ | ✓ | ✗ |
You can join a group dialog only if your user ID is present in the
occupants_ids
array, in the dialog model.Your user ID is added to the
occupants_ids
array if you create a dialog or you are added to the dialog by the other user. See this section to learn how to add occupants to the group dialog.
Leave dialog
You can leave the group or public dialog by calling the leave()
method. If the dialog is left, you can't send/receive messages. To be able to receive/send messages, you need to join it.
var dialogJid = QB.chat.helpers.getRoomJidFromDialogId(dialog._id);
try {
QB.chat.muc.leave(dialogJid, function(error) {
});
} catch (e) {
if (e.name === 'ChatNotConnectedError') {
// not connected to chat
}
}
Argument | Required | Description |
---|---|---|
dialogJid | yes | Room JID. JID (Jabber ID) of XMPP room in the XMPP server. Empty for a privatе dialog. Generated automatically by the server after dialog creation. You can get JID from the dialog ID. The JID format is the following: <app_id>-<dialog_id>@muc.chat.quickblox.com |
function() | yes | Specifies a callback function that accepts an error. |
Let's see, how the leave()
method is used with regard to the dialog type.
Capabilities | Public | Group | Private |
---|---|---|---|
Leave | ✓ | ✓ | ✗ |
When a group dialog is left, your user ID is still present in the
occupants_ids
array, in the dialog model. As a result, the dialog will still be present in the list of dialogs and you will still have access to the chat history.To remove yourself from the group dialog, use the
update()
method. See this section to learn how to remove occupants from the group dialog.
Retrieve online users
You can get a list of online users from the dialog. Call the listOnlineUsers()
method to get the list of online users who are joined to the dialog. As a result, an array of user IDs is returned.
var dialogId = "...";
var dialogJid = QB.chat.helpers.getRoomJidFromDialogId(dialogId);
try {
QB.chat.muc.listOnlineUsers(dialogJid, function(users) {
});
} catch (e) {
if (e.name === 'ChatNotConnectedError') {
// not connected to chat
}
}
Let's see, how the listOnlineUsers()
method is used with regard to the dialog type.
Capabilities | Public | Group | Private |
---|---|---|---|
Retrieve online users | ✗ | ✓ | ✗ |
You can retrieve online users from the group dialog only if you are joined to it.
Retrieve list of dialogs
It's common to request all your dialogs on every app login. The request below will return all private, group, and public dialogs created before the current date, sorted in descending order by the created_at
field, and limited to 10 dialogs on the page.
let params = {
created_at: {
lt: Date.now()/1000
},
sort_desc: 'created_at',
limit: 10
};
QB.chat.dialog.list(params, function(error, dialogs) {
});
Argument | Required | Description |
---|---|---|
params | no | Specifies param fields that should be set. |
function() | yes | A callback function that an accepts error and dialogs. |
If you want to retrieve only dialogs updated after some specific date time and order the search results, you can apply operators. This is useful if you cache dialogs somehow and do not want to obtain the whole list of your dialogs on every app start. Thus, you can apply search and sort operators to the list of dialogs on the page so that it is easier to view specific dialogs.
If you want to get a paginated list of dialogs or just a count of dialogs from the server, you can set the following fields of the filter
:
Field | Required | Description |
---|---|---|
skip | no | Skip N records in search results. Useful for pagination. Default (if not specified): 0. Should be an Integer. |
limit | no | Limit search results to N records. Useful for pagination. Default value: 100. |
Search operators
You can use search operators to get more specific search results. The request below will return a list of all private and group dialogs.
let params = {
type: {
in: [2,3]
}
};
QB.chat.dialog.list(params, function(error, dialogs) {
});
Here are the search operator that you can use to search for the exact data that you need.
Search operators | Applicable to types | Applicable to fields | Description |
---|---|---|---|
lt | number, string, date | last_message_date_sent, created_at, updated_at | Less Than operator. |
lte | number, string, date | last_message_date_sent, created_at, updated_at | Less Than or Equal to operator. |
gt | number, string, date | last_message_date_sent, created_at, updated_at | Greater Than operator. |
gte | number, string, date | last_message_date_sent, created_at, updated_at | Greater Than or Equal to operator. |
ne | number, string, date | _id, name, last_message_date_sent | Not Equal to operator. |
in | number, string, date | type, last_message_date_sent, name | IN array operator. |
nin | number, string, date | last_message_date_sent | IN array operator. |
all | number | occupants_ids | ALL are contained in array. |
ctn | string | name | All records that contain a particular substring. |
Sort operators
You can use sort operators to order the search results. The request below will return dialogs created since the beginning of this year and sorted in descending order.
let params = {
created_at: {
gte: (new Date(new Date().getFullYear(), 0, 1))/1000
},
sort_desc: 'sort_desc'
};
QB.chat.dialog.list(params, function(error, dialogs) {
});
Here are the sort operators that you can use to order search results.
Sort operator | Applicable to types | Applicable to fields | Description |
---|---|---|---|
sort_asc | All types | id, created_at, name, last_message_date_sent | Search results will be sorted in ascending order by the specified field. |
sort_desc | All types | id, created_at, name, last_message_date_sent | Search results will be sorted in descending order by the specified field. |
Aggregation operators
You can use an aggregation operator to count search results. The request below will return a count of all group dialogs from the server.
let params = {
type: 2,
count:1
};
QB.chat.dialog.list(params, function(err, result) {
if (err) {
console.log(err);
} else {
}
});
Here are the aggregation operators you can use to retrieve dialogs.
Aggregation operator | Description |
---|---|
count | Count search results. The response will contain only a count of records found. Set count to 1 to apply. |
Retrieve dialogs by custom parameters
You can retrieve dialogs by custom parameters. The request below will return all private, group, and public dialogs with a given category
and class_name
.
var filters = {
data: { // search by custom parameters
category:'friends',
class_name:'CoolDialog'
}
};
QB.chat.dialog.list(filters, function(error, dialogs) {});
Update dialog
You can update the information for a private, group, and public dialog.
var dialogId = "5356c64ab35c12bd3b108a41";
var toUpdateParams = {
name: "Tesla club"
};
QB.dialog.update(dialogId, toUpdateParams, function(error, dialog) {});
Let's see what capabilities a particular user role has with regard to the dialog type.
Capabilities | Public dialog | Group dialog | Private dialog |
---|---|---|---|
Update a dialog name | Owner | Owner | ✗ |
Update a photo | Owner | Owner | ✗ |
Update custom parameters | Owner, Occupant | Owner, Occupant | Owner, Occupant |
Add occupants
Set the push_all
field to add occupants to the dialog. As a result, the ID of the opponent will be added to the occupants_ids
array.
let
dialogId = "5356c64ab35c12bd3b108a41",
toUpdateParams = {
push_all: { occupants_ids: [97, 789] }
};
QB.chat.dialog.update(dialogId, toUpdateParams, function(error, dialog) {});
Arguments | Required | Description |
---|---|---|
dialogId | yes | Dialog ID. |
toUpdateParams | yes | Specifies the toUpdateParams fields that should be set. |
Let's see what capabilities a particular user role has with regard to the dialog type.
Capabilities | Public dialog | Group dialog | Private dialog |
---|---|---|---|
Add other users | ✗ | Owner, Occupant | ✗ |
Remove occupants
Set the pull_all
field to remove occupants from the dialog. As a result, the ID of the opponent will be removed from the occupants_ids
array.
let
dialogId = "5356c64ab35c12bd3b108a41",
toUpdateParams = {
pull_all: { occupants_ids: [97, 789] }
};
QB.chat.dialog.update(dialogId, toUpdateParams, function(error, dialog) {});
Argument | Required | Description |
---|---|---|
dialogId | yes | Dialog ID. |
toUpdateParams | yes | Specifies the toUpdateParams fields that should be set. |
Let's see what capabilities a particular user role has with regard to the dialog type.
Capabilities | Public dialog | Group dialog | Private dialog |
---|---|---|---|
Remove other users | ✗ | Owner | ✗ |
Remove yourself | ✗ | Owner, Occupant | ✗ |
Delete dialog
The request below will remove the dialog for a current user, but other users will be still able to chat there.
var dialogId = "5356c64ab35c12bd3b108a41";
QB.chat.dialog.delete([dialogId], function(error) {
});
Set the force
to 1
to completely remove the dialog for all users. You can also delete multiple dialogs in a single request.
var dialogId = "5356c64ab35c12bd3b108a41";
QB.chat.dialog.delete([dialogId], {force: 1}, function(error) {
});
Let's see what capabilities a particular user role has with regard to the dialog type.
Capabilities | Public | Group | Private |
---|---|---|---|
Delete dialog for all user using force parameter. | Owner | Owner | Owner |
Delete dialog for a current user | Owner | Owner, Occupant | Owner, Occupant |
Get number of unread messages
You can get a number of unread messages from a particular dialog using the list()
method.
var filters = { limit: 1 };
QB.chat.dialog.list(filters, function(error, dialogs) {
if (error) {
// handle error
} else {
var dialog = dialogs.items[0];
var unreadCount = dialog.unread_messages_count;
}
})
You can also retrieve total unread messages count using unreadCount()
method.
var params = {
chat_dialog_ids: ["5356c64ab35c12bd3b108a41"]
};
QB.chat.message.unreadCount(params, function(error, result) {
});
Set the following fields of the params
:
Field | Description |
---|---|
chat_dialog_ids | IDs of dialogs. - If chat_dialog_ids are not specified, the total number of unread messages for all dialogs of the user will be returned.- If chat_dialog_ids are specified, the number of unread messages for each specified dialog will be returned. Also, the total number of unread messages for all dialogs of the user will be returned. |
Resources
A sequence of steps a user takes to start a dialog by moving through the application lifecycle.
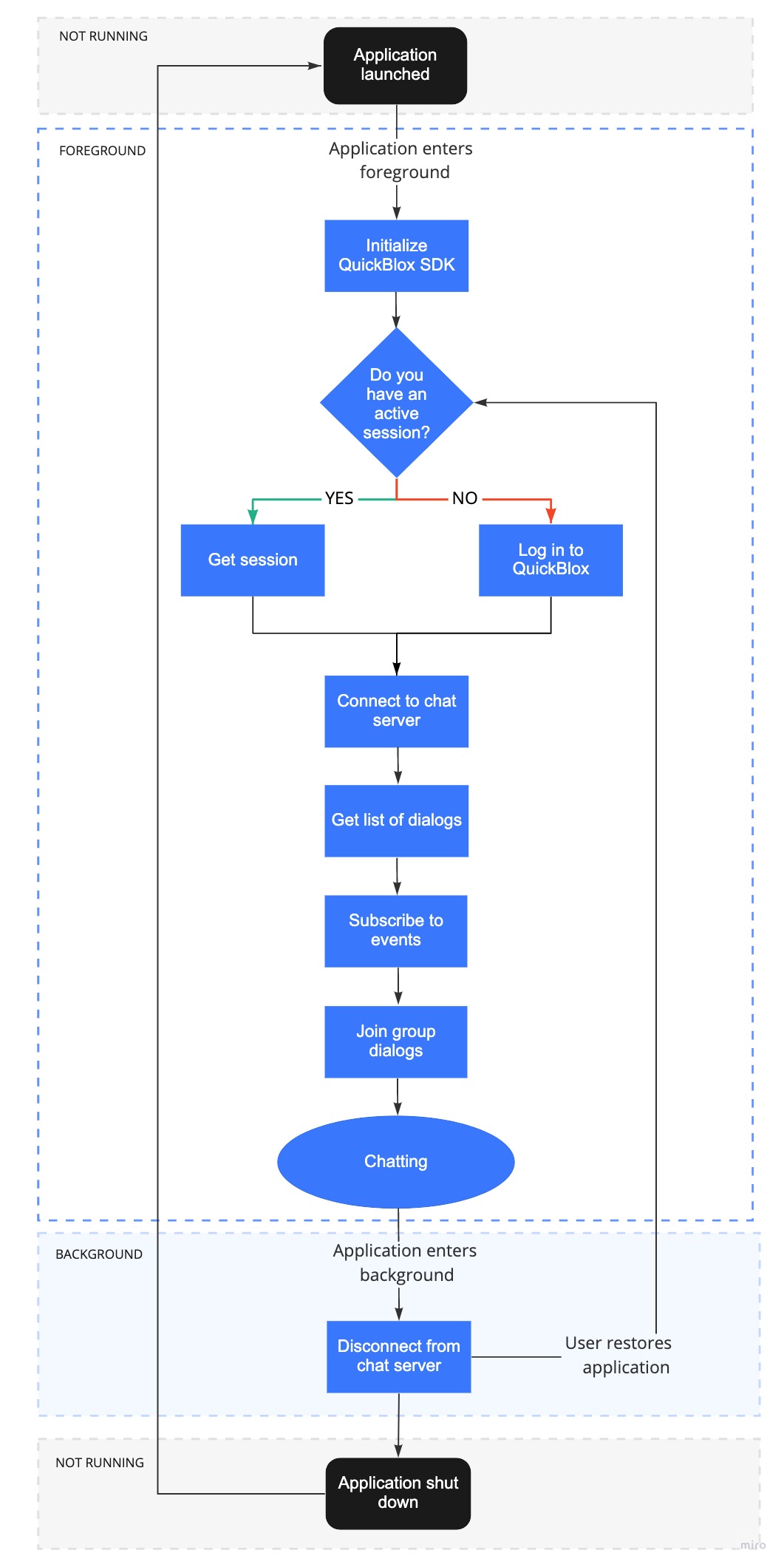
Updated over 2 years ago