Setup
Learn how to add and configure QuickBlox SDK for your app.
Follow the instructions below to ensure that QuickBlox SDK runs smoothly with your app.
Visit our Key Concepts page to get an overall understanding of the most important QuickBlox concepts.
Get application credentials
QuickBlox application includes everything that brings messaging right into your application - chat, video calling, users, push notifications, etc. To create a QuickBlox application, follow the steps below:
- Register a new account following this link. Type in your email and password to sign in. You can also sign in with your Google or Github accounts.
- Create the app by clicking the New app button.
- Configure the app. Type in the information about your organization into corresponding fields and click Add button.
- Go to Dashboard => YOUR_APP => Overview section and copy your Application ID, Authorization Key, Authorization Secret, and Account Key .
Requirements
The minimum requirements for QuickBlox React Native SDK are:
- iOS 12.0
- Android (minimum version 5.0, API 21)
- React Native (minimum version 0.60)
Install QuickBlox SDK into your app
To manage project dependencies:
- Node.js and npm must be installed
- CocoaPods must be installed for iOS.
To connect QuickBlox to your app just add it into your project's dependencies by running following code sample in terminal (in your react-native app):
npm install quickblox-react-native-sdk
iOS and Android have different dependencies systems. For that reason, you need to install dependencies in your iOS project. Navigate to ios/ folder in the root directory of the project and enter the following code snippet.
pod install
Add permissions
For Android
If your app requires adding some specific permissions to access camera, microphone, internet, and storage permissions, add these permissions to your app manifest.
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
Note that mentioning the camera and microphone permissions in the manifest isn't always enough. You need to request camera and microphone permissions additionally at runtime.
For iOS
You can use our SDK in the background mode as well. If you want to receive push notifications when the app goes to background mode, this requires you to add specific app permissions. Under the app build settings, open the Capabilities tab and turn on Remote notifications checkbox only.
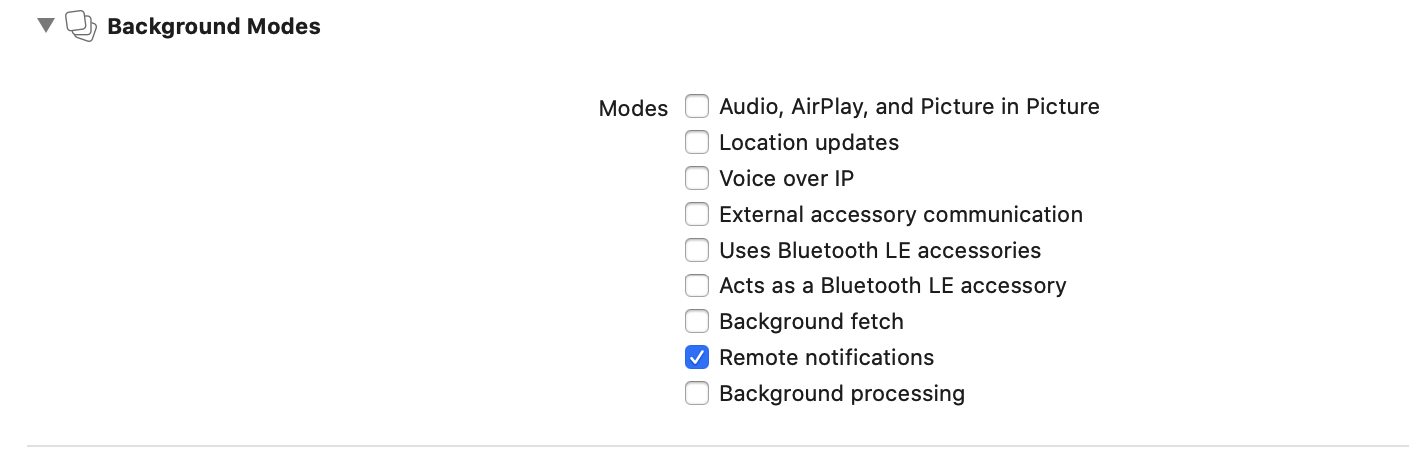
If you want to use video calling functionality in the background mode set the Audio, AirPlay, and Picture in Picture checkboxes.
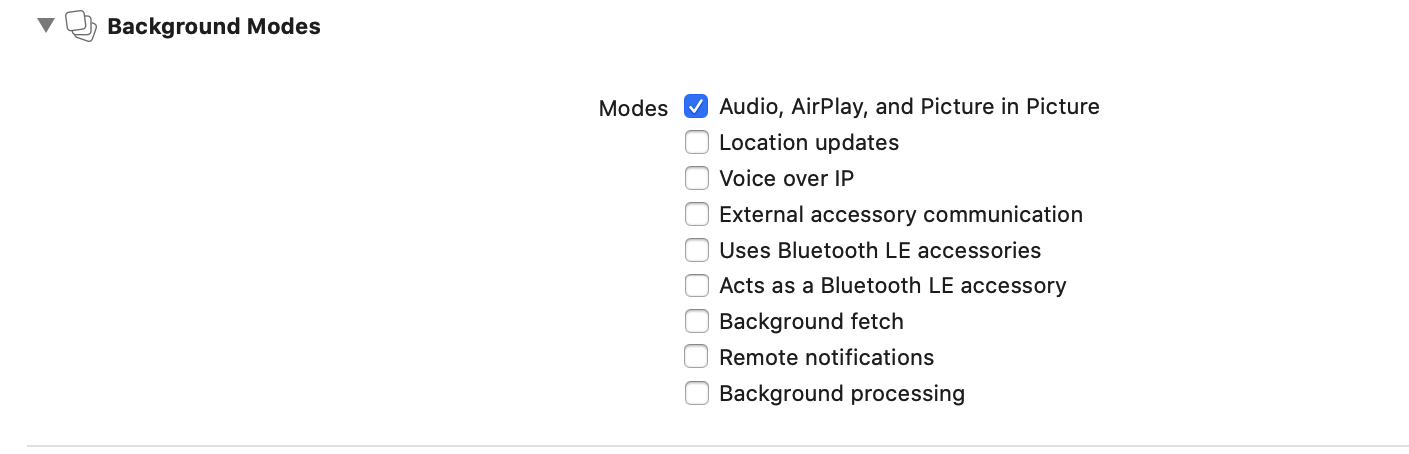
Initialize QuickBlox SDK
You must initialize SDK before calling any methods through the SDK, except for the
init()
method. If you attempt to call a method without initializing SDK previously - the error will be returned.
Initialize the framework with your application credentials. Specify Application ID, Authorization Key, Authorization Secret, and Account Key within appSettings
object and pass it to the init()
method.
const appSettings = {
appId: '',
authKey: '',
authSecret: '',
accountKey: ''
};
QB.settings
.init(appSettings)
.then(function () {
// SDK initialized successfully
})
.catch(function (e) {
// Some error occurred, look at the exception message for more details
});
Security
It's not recommended to keep your authKey and authSecret inside an application in production mode, instead of this, the best approach will be to store them on your backend.
The init()
method accepts one argument of the object type that has the following fields:
Field | Required | Description |
---|---|---|
appId | yes | Application ID. |
authKey | yes | Authorization key. |
authSecret | yes | Authorization secret. |
accountKey | yes | Required to get actual apiEndpoint and chatEndpoint for the right server. |
Initialize QuickBlox SDK without Authorization Key and Secret
You may don't want to store authKey and authSecret inside an application for security reasons. In such case, you can initialize QuickBlox SDK with applicationId and accountKey only, and store your authKey and authSecret on your backend. But, if so, the implementation of authentication with QuickBlox should be also moved to your backend.
const appSettings = {
appId: '',
accountKey: ''
};
QB.settings
.init(appSettings)
.then(function () {
// SDK initialized successfully
})
.catch(function (e) {
// Some error occurred, look at the exception message for more details
});
Then using your backend you can authorize a user in the QuickBlox system, send back the user session token, and set it to the QuickBlox SDK using startSessionWithToken()
method. You can find out more about this in the Set existing session section.
Point SDK to enterprise server
To point QuickBlox SDK to the QuickBlox enterprise server, you should set apiEndpoint
and chatEndpoint
fields of the appSettings
object and pass it to the init()
method.
const appSettings = {
appId: '',
authKey: '',
authSecret: '',
accountKey: '',
apiEndpoint: '', // optional
chatEndpoint: '' // optional
};
QB.settings
.init(appSettings)
.then(function () {
// SDK initialized successfully
})
.catch(function (e) {
// Some error occurred, look at the exception message for more details
});
The init()
method accepts one argument of the object type that has the following fields:
Field | Required | Description |
---|---|---|
appId | yes | Application ID. |
authKey | yes | Authorization key. |
authSecret | yes | Authorization secret. |
accountKey | yes | Required to get actual apiEndpoint and chatEndpoint for the right server. |
apiEndpoint | no | API endpoint. |
chatEndpoint | no | Chat endpoint. |
Contact our sales team to get API endpoint and chat endpoint.
Enable auto-reconnect to Chat
QuickBlox Chat runs over XMPP protocol. To receive messages in a real-time mode, the application should be connected to the Chat over XMPP protocol. To enable auto-reconnect to Chat, call enableAutoReconnect()
method and pass enable
as true
.
const autoReconnectParams = { enable: true };
QB.settings
.enableAutoReconnect(autoReconnectParams)
.then(function () {
// operation completed successfully
})
.catch(function (e) {
// Some error occurred, look at the exception message for more details
});
Thus, if XMPP connection is lost and autoreconnect functionality is enabled, the app connects to Chat automatically. You can disable autoreconnection to Chat by passing enable
parameter as false
to enableAutoReconnect()
method.
const autoReconnectParams = { enable: false };
QB.settings
.enableAutoReconnect(autoReconnectParams)
.then(function () {
// operation completed successfully
})
.catch(function (e) {
// Some error occurred, look at the exception message for more details
});
By default, this parameter is enabled. Set autoreconnection before calling the
login()
method so it could be applied in a current chat.
Enable logging
Logging functionality allows you to keep track of all events and activities while running your app. As a result, you can monitor the operation of the SDK and improve the debug efficiency. There are 3 logging use cases:
- Server API logging is used to monitor Server API calls.
- Chat logging is used to monitor chat issues.
- WebRTC logging is used to gather issues with video.
- Go over this section to learn how to enable logging for your iOS app.
- Go over this section to learn how to enable logging for your Android app.
Message carbons
Message carbons functionality allows for multi-device support. Thus, all user messages get copied to all their devices so they could keep up with the current state of the conversation. For example, a User A has phone running conversations and desktop running conversations. User B has desktop running conversations. When User B sends a message to User A, the message shows on both the desktop and phone of User A.
Enable message carbons
QB.settings
.enableCarbons()
.then(function () {
// operation completed successfully
})
.catch(function (e) {
// Some error occurred, look at the exception message for more details
});
Disable message carbons
QB.settings
.disableCarbons()
.then(function () {
// operation completed successfully
})
.catch(function (e) {
// Some error occurred, look at the exception message for more details
});
By default, this parameter is turned off. Keep it enabled if you want to receive messages on all devices of the particular user (when a user is logged in on several devices).
Stream management
Stream management has two important features Stanza Acknowledgements and Stream Resumption:
- Stanza Acknowledgements is the ability to know if a stanza or series of stanzas has been received by one's peer. In other words, a reply is requested on every sent message. If the reply is received, the message is considered as delivered.
- Stream Resumption is the ability to quickly resume a stream that has been terminated. So once a connection is re-established, Stream Resumption is executed. By matching the sequence numbers assigned to each Stanza Acknowledgement a server and client can verify which messages are missing and request to resend missing messages.
Call initStreamManagement()
method and pass autoReconnect
and messageTimeout
parameters to it to enable stream management.
const streamManagementParams = {
autoReconnect: false,
messageTimeout: 10
};
QB.settings
.initStreamManagement()
.then(function () {
// operation completed successfully
})
.catch(function (e) {
// Some error occurred, look at the exception message for more details
});
The initStreamManagement()
method accepts one argument of the object type that has the following fields:
Field | Required | Description |
---|---|---|
autoReconnect | yes | Set it as true to use Stream resumption for new connections. Default: false. |
messageTimeout | yes | Preferred resumption time (in seconds). If this parameter is greater than 0 , than it is applied, otherwise it is not applied. |
You should enable Stream Management before you do the
login()
because the Stream Management is initialized while Chat login is performed.The Stream Management defines an extension for active management of a stream between a client and server, including features for stanza acknowledgments.
Updated over 1 year ago