AI Features
AI Features base on OpenAI GЗt
Overview
Starting from version 0.2.0 of React UI Kit, the AI functionality is enabled by default and based on OpenAI's chat GPT. The QuickBlox AI for React UI Kit provides a range of features to enhance the chat experience. With essential messaging functionalities such as answer assistant, users can engage in more interactive conversations.
Supported features
Name | Description |
---|---|
AI Assist | Provides answers based on chat history to selected message. |
AI Translate | Provides translation based on chat history to selected incoming message. |
AI Rephrase | Provides rephrase in selected tone based on chat history to inputed message before sending. |
Requirements
The minimum requirements for using AI features are:
- JS QuickBlox SDK v2.15.5
- React v.18.0
- TypeScript v.4.9.3
- React UI Kit v0.2.0
AI Assist Answer
QuickBlox provides answer assistant functionality that helps users effortlessly send various answers considering chat history.
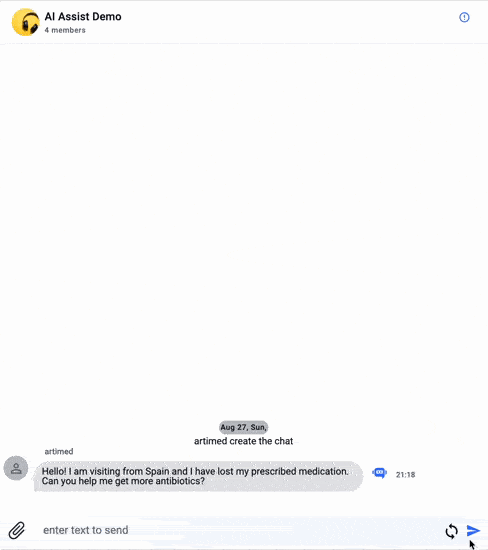
How to use in React UI Kit
Enabling QuickBlox AI answer assistant in React UI Kit involves just 2 straightforward actions:
- Set up the
AIAnswerAssistWidgetConfig
in theconfigAIApi
segment of theQBConfig.ts
file to provide your API Key. - Optionally, initialize the
AIAssist
property within theQuickBloxUIKitDesktopLayout
(orMessageView
) component for customizing the appearance or logic of the AI Assist component.
There are two ways to use the QuickBlox AI Assist answer:
- Direct: Access Open AI directly from your client code. The
AIAssist
component use a raw Open AI token. This is suitable for development or demos, but it's not the recommended approach for production due to security considerations. - Proxy: Access Open AI through a proxy server. The
AIAssist
component use QuickBlox user session token with a proxy server. This is the recommended method for production environments, ensuring a more secure and controlled setup.
Directly using configuration
To utilize the QuickBlox AI Assist answer React hook to communicate directly with the OpenAI API , you should configure the AIAnswerAssistWidgetConfig section in the configuration file, as shown in the code snippet provided below. The token should be stored in the apiKey
property in the configAIApi
section in the QBConfig.ts
file. This contains information about configurations for all AI features . AFter that theAIAssist
component will communicate directly with the OpenAI API.
export const QBConfig = {
credentials: {
//.. other section data
},
configAIApi: {
AIAnswerAssistWidgetConfig: {
apiKey: 'sk-k2...LfK', // Замените на ваш реальный ключ API
useDefault: true,
//... other section data
},
},
appConfig: {
//... other section data
},
};
Parameter name | Type | Description |
---|---|---|
apiKey | String | This field should hold your actual API key that you'll receive from the service provider (in this case, OpenAI). This key is used to authenticate your requests to the AI service. |
useDefault | Boolean | This setting seems to indicate whether you want to use default settings for the AI answer assistance widget. If set to true, it implies that the default settings will be applied. |
Proxy using configuration
To utilize the QuickBlox AI Assist answer React hook to communicate through a proxy server (not directly) with OpenAI Api, you should configure the AIAnswerAssistWidgetConfig section in the configuration file, as shown in the code snippet provided below. The fields api
, servername
, port
, and sessionToken
need to be configured within the proxyConfig section for communication with the proxy server.
export const QBConfig = {
credentials: {
//... other section data
},
configAIApi: {
AIAnswerAssistWidgetConfig: {
apiKey: '', // must be empty
useDefault: true,
proxyConfig: {
api: 'v1/chat/completions',
servername: 'https://myproxy.com',
port: '',
sessionToken: '',
},
},
},
appConfig: {
//... other section data
},
};
Parameter name | Type | Description |
---|---|---|
api | String | The specific API endpoint for AI completions.In default, it's set to v1/chat/completions . You should provide your url. |
serverName | String | The base URL for API requests. In default, it's set to 'https://api.openai.com/'. You should provide your url. |
sessionToken | String | Your should provide the QuickBlox user session token by default if you are using our proxy server. |
port | String | Default should be empty. |
We recommend using our proxy server, which you can access through this link. Please ensure that you are using the latest release.
AI Assist customization
You have the ability to control AI Assist Answer from code as follows: set it to enabled/disabled, customize the appearance of the component icon, and modify its operational logic. To enable or disable AIAssist use the following code:
<QuickBloxUIKitDesktopLayout
theme={new CustomTheme()}
AIAssist={{ enabled: true, default: true }}
/>
The QuickBloxUIKitDesktopLayout
is a layout component located within the provider section. We explored an example of its usage in our UI Kit documentation, specifically in the Send your First Message section.
To customize the appearance of the component icon, you should create your own React custom hook that must return an object of the AIMessageWidget interface and update the code within the renderWidget method.
For modifying its operational logic, you should create your own React custom hook that must return an object of the AIMessageWidget interface and update the code within the textToWidget method. The interface AIMessageWidget
has next structure:
Parameter name | Type | Description |
---|---|---|
renderWidget | Object function | The renderWidget provides the default appearance of the widget. This is a method that returns a JSX element (React component). It's responsible for rendering the widget, and depending on the presence of errors, it might display an error icon or a support icon. So you should change it to implement your own icon. |
textToWidget | Object function | The texToWidget provides the default logic of the widget. This is a function that takes a string (text) and context (in this case, an array of chat messages) but doesn't return anything (void). This function is used to pass text into the widget. So you should change it to implement your logic. |
textToContent | String | The textToContent provides the output of the default algorithm implementation. This is a string field representing content for the text widget. It's likely to be used to display the response obtained from AI in the text widget. |
Example code of custom hook:
interface MessageWidgetProps {
servername: string;
api: string;
port: string;
sessionToken: string;
}
export default function useDefaultAIAssistAnswerWidgetWithOpenAIApi({
servername,
api,
port,
sessionToken,
}: MessageWidgetProps): AIMessageWidget {
const [errorMessage, setErrorMessage] = useState<string>('');
const [textFromWidgetToContent, setTextFromWidgetToContent] = useState('');
const renderWidget = (): JSX.Element => {
if (errorMessage && errorMessage.length > 0) {
const errorsDescriptions:
| { title: string; action: () => void }[]
| undefined = [];
return (
<ErrorMessageIcon
errorMessageText={errorMessage}
errorsDescriptions={errorsDescriptions}
/>
);
}
// you can customize your own Widget Icon markup
return <AIWidgetIcon applyZoom color="green" />;
};
const textToWidget = (value: string, context: IChatMessage[]): void => {
if (value && value.length > 0) {
getData(value, context as ChatCompletionRequestMessage[]).then(
(data) => {
setTextFromWidgetToContent(data);
},
);
}
};
async function getData(
textToSend: string,
dialogMessages: ChatCompletionRequestMessage[],
): Promise<string> {
let answerSuggetion = '';
if (textToSend.length === 0) return answerSuggetion;
// your logic to use openai
return answerSuggetion;
}
return {
textToContent: textFromWidgetToContent,
renderWidget,
textToWidget,
};
}
To set up your own realization you should use the property AIAssist
inQuickBloxUIKitDesktopLayout
or in MessageView
components like this:
Let's modify the QuickBloxUIKitDesktopLayout component from "Send your first message" page :
import React from 'react';
import * as QB from "quickblox/quickblox";
import {
LoginData,
QuickBloxUIKitProvider,
qbDataContext,
RemoteDataSource,
useQBConnection, QuickBloxUIKitDesktopLayout,
} from 'quickblox-react-ui-kit';
function App() {
const currentUser: LoginData = {
userName: 'YOUR_REGISTRED_USER_NAME',
password: 'YOUR_REGISTRED_USER_PASSWORD',
};
const apiKey = 'sk-9aXs...ZFqhU'; // use your Api Key
const openAIConfiguration: Configuration = new Configuration({
apiKey,
});
const openAIApi: OpenAIApi = new OpenAIApi(openAIConfiguration);
const defaultAIAnswerToMessageWidget =
useDefaultAIAssistAnswerWidgetWithOpenAIApi({
...QBConfig.configAIApi.AITranslateWidgetConfig.proxyConfig,
openAIApi,
});
return (
<QuickBloxUIKitProvider
maxFileSize={100 * 1000000}
accountData={{ ...QBConfig.credentials }}
loginData={{
login: currentUser.login,
password: currentUser.password,
}} >
<div className="App">
<QuickBloxUIKitDesktopLayout
theme={new DefaultTheme()}
AIAssist={{
enabled: true,
default: false,
AIWidget: defaultAIAnswerToMessageWidget,
}}
/>
</div>
</QuickBloxUIKitProvider>
);
}
export default App;
in MessageView component se detail information on this page :
<DesktopLayout
theme={theme}
dialogsView={
<DialogsComponent
// ...
/>
}
dialogMessagesView={
<MessagesView
subHeaderContent={<CompanyLogo />}
upHeaderContent={<CompanyLogo />}
dialogsViewModel={dialogsViewModel}
onDialogInformationHandler={informationOpenHandler}
theme={theme}
AIAnswerToMessage={defaultAIAnswerToMessageWidget}
/>
}
dialogInfoView={
<DialogInformation
// ...
/>
}
/>
You can compare the configuration of the QuickBloxUIKitDesktopLayout component with the AI Widget to the default view of the QuickBloxUIKitDesktopLayout component from the "Send your first message" page .
How to use the feature as a standalone library
You can use logic of the React hook features separately from our UI kit is possible by connecting the library as described below. The library qb-ai-answer-assistant contains QBAIAnswerAssistant object wich provide all nececry features.
install standalone library
QBAIAnswerAssistant can be installed using Node Package Manager. To include it in your JS based project, follow these command:
npm install qb-ai-answer-assistant
add to own project
To use QBAIAnswerAssistant in your project, follow these steps:
- Import the QBAIAnswerAssistant module:
import { ChatMessage } from 'qb-ai-core';
import { AIAnswerAssistantSettings, QBAIAnswerAssistant } from 'qb-ai-answer-assistant';
- Create an array of
ChatMessage
objects representing the chat history:
let prompt = "YOUR_TEXT_TO_ANSWER_ASSIST";
let messages = [
{role: Role.other, content: "Hello, Bob!"},
{role: Role.me, content: "Hi, Jim!"},
{role: Role.me, content: prompt},
]
or
let prompt = "YOUR_TEXT_TO_ANSWER_ASSIST";
let messages = [
{role: "assistant", content: "Hello, Bob!"},
{role: "user", content: "Hi, Jim!"},
{role: "user", content: prompt},
]
- Call the
rephrase
method to generate translate using an API key:
const settings: AIAnswerAssistantSettings =
QBAIAnswerAssistant.createDefaultAIAnswerAssistantSettings();
settings.apiKey = 'YOUR_OPEN_AI_API_KEY';
settings.organization = 'YOUR_ORGANIZATION';// might be empty
settings.model = AIModel.gpt__3_5__turbo;
return QBAIAnswerAssistant.createAnswer(
promt,
messages,
settings,
);
Alternatively, you can use a QuickBlox user token and a proxy URL for more secure communication:
const settings: AIAnswerAssistantSettings =
QBAIAnswerAssistant.createDefaultAIAnswerAssistantSettings();
settings.organization = 'YOUR_ORGANIZATION';// might be empty
settings.model = AIModel.gpt__3_5__turbo;
settings.token = 'YOUR_QUICKBLOX_USER_SESSION_TOKEN';
settings.serverPath = `https://your-proxy-server-url`;
return QBAIAnswerAssistant.createAnswer(
promt,
messages,
settings,
);
AI Translate
QuickBlox offers translation functionality that helps users easily translate text messages in chat, taking into account the context of the chat history.
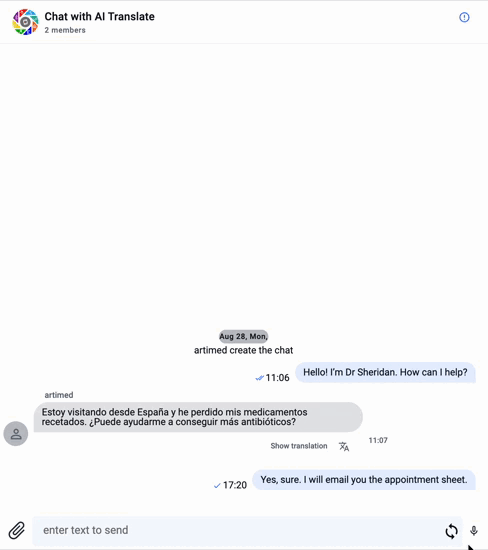
How to use
Enabling QuickBlox AI Translate in React UI Kit involves just 2 straightforward actions:
- Set up the
AITranslateWidgetConfig
in theconfigAIApi
segment of theQBConfig.ts
file to provide your API Key. - Optionally, initialize the
AITranslate
property within theQuickBloxUIKitDesktopLayout
(orMessageView
) component for customizing the appearance or logic of the AI Translate component.
There are two ways to use the QuickBlox AI Translate:
- Direct: Access Open AI directly from your client code. The
AITranslate
component use a raw Open AI token. This is suitable for development or demos, but it's not the recommended approach for production due to security considerations. - Proxy: Access Open AI through a proxy server. The
AITranslate
component use QuickBlox user session token with a proxy server. This is the recommended method for production environments, ensuring a more secure and controlled setup.
Directly using configuration
To utilize the QuickBlox AI Translate React hook to communicate directly with the OpenAI API , you should configure the AITranslateWidgetConfig section in the configuration file, as shown in the code snippet provided below. The token should be stored in the apiKey
property in the configAIApi
section in the QBConfig.ts
file. This contains information about configurations for all AI features . AFter that theAITranslate
component will communicate directly with the OpenAI API.
export const QBConfig = {
credentials: {
//.. other section data
},
configAIApi: {
AITranslateWidgetConfig: {
apiKey: 'sk-k2...LfK', // Change to your real API key
useDefault: true,
defaultLanguage: 'English',
languages: [
'English',
'Spanish',
'French',
'Portuguese',
'German',
'Ukrainian',
],
//... other section data
},
},
appConfig: {
//... other section data
},
};
Parameter name | Type | Description |
---|---|---|
apiKey | String | This field should hold your actual API key that you'll receive from the service provider (in this case, OpenAI). This key is used to authenticate your requests to the AI service. |
useDefault | Boolean | This setting seems to indicate whether you want to use default settings for the AI answer assistance widget. If set to true, it implies that the default settings will be applied. |
defaultLanguage | String | This parameter specifies the default language you will use for translation. If this parameter is not set, the language from the system settings will be used (the language set in the system). If it's not possible to determine the system language, then English will be used by default. |
languages | Array String | This option allows you to set an array of alternative languages that can be used for translation. This list of languages will be displayed in the context menu before performing the text translation. |
Proxy using configuration
To utilize the QuickBlox AI Translate React hook to communicate through a proxy server (not directly) with OpenAI Api, you should configure the AITranslateWidgetConfig section in the configuration file, as shown in the code snippet provided below. The fields api
, servername
, port
, and sessionToken
need to be configured within the proxyConfig section for communication with the proxy server.
export const QBConfig = {
credentials: {
//... other section data
},
configAIApi: {
AITranslateWidgetConfig: {
apiKey: '', // must be empty
useDefault: true,
defaultLanguage: 'English',
languages: [
'English',
'Spanish',
'French',
'Portuguese',
'German',
'Ukrainian',
]
proxyConfig: {
api: 'v1/chat/completions',
servername: 'https://myproxy.com',
port: '',
sessionToken: '',
},
},
},
appConfig: {
//... other section data
},
};
Parameter name | Type | Description |
---|---|---|
api | String | The specific API endpoint for AI completions.In default, it's set to v1/chat/completions . You should provide your url. |
serverName | String | The base URL for API requests. In default, it's set to 'https://api.openai.com/'. You should provide your url. |
sessionToken | String | Your should provide the QuickBlox user session token by default if you are using our proxy server. |
port | String | Default should be empty. |
We recommend using our proxy server, which you can access through this link. Please ensure that you are using the latest release.
AI Translate customization
You have the ability to control AI Translate from code as follows: set it to enabled/disabled, customize the appearance of the component icon, and modify its operational logic. To enable or disable AI Translate use the following code:
<QuickBloxUIKitDesktopLayout
theme={new CustomTheme()}
AITranslate={{ enabled: true, default: true }}
/>
The QuickBloxUIKitDesktopLayout
is a layout component located within the provider section. We explored an example of its usage in our UI Kit documentation, specifically in the Send your First Message section.
To customize the appearance of the component icon, you should create your own React custom hook that must return an object of the AIMessageWidget interface and update the code within the renderWidget method.
For modifying its operational logic, you should create your own React custom hook that must return an object of the AIMessageWidget interface and update the code within the textToWidget method. The interface AIMessageWidget
has next structure:
Parameter name | Type | Description |
---|---|---|
renderWidget | Object function | The renderWidget provides the default appearance of the widget. This is a method that returns a JSX element (React component). It's responsible for rendering the widget, and depending on the presence of errors, it might display an error icon or a support icon. So you should change it to implement your own icon. |
textToWidget | Object function | The texToWidget provides the default logic of the widget. This is a function that takes a string (text) and context (in this case, an array of chat messages) but doesn't return anything (void). This function is used to pass text into the widget. So you should change it to implement your logic. |
textToContent | String | The textToContent provides the output of the default algorithm implementation. This is a string field representing content for the text widget. It's likely to be used to display the response obtained from AI in the text widget. |
Example code of custom hook:
interface MessageWidgetProps {
servername: string;
api: string;
port: string;
sessionToken: string;
}
export default function useDefaultAITranslateWidget({
servername,
api,
port,
sessionToken,
}: MessageWidgetProps): AIMessageWidget {
const [errorMessage, setErrorMessage] = useState<string>('');
const [textFromWidgetToContent, setTextFromWidgetToContent] = useState('');
const renderWidget = (): JSX.Element => {
if (errorMessage && errorMessage.length > 0) {
const errorsDescriptions:
| { title: string; action: () => void }[]
| undefined = [];
return (
<ErrorMessageIcon
errorMessageText={errorMessage}
errorsDescriptions={errorsDescriptions}
/>
);
}
// you can customize your own Widget Icon markup
return <AIWidgetIcon applyZoom color="green" />;
};
const textToWidget = async (
textToSend: string,
context: IChatMessage[],
additionalSettings?: { [key: string]: any },
): Promise<string> => {
if (textToSend && textToSend.length > 0) {
let prompt = "Your prompt text with textToSend data";
const { yourAdditionalParam } = additionalSettings || {};
// your logic
return await getData(prompt, context).then((data) => {
setTextFromWidgetToContent(data);
return data;
});
}
return '';
};
async function getData(
prompt: string,
dialogMessages: IChatMessage[],
): Promise<string> {
let outputMessage = '';
// your logic to use openai
return outputMessage;
}
return {
textToContent: textFromWidgetToContent,
renderWidget,
textToWidget,
};
}
To set up your own realization you should use the property AITranslate
inQuickBloxUIKitDesktopLayout
or in MessageView
components like this:
Let's modify the QuickBloxUIKitDesktopLayout component from "Send your first message" page :
import React from 'react';
import * as QB from "quickblox/quickblox";
import {
LoginData,
QuickBloxUIKitProvider,
qbDataContext,
RemoteDataSource,
useQBConnection, QuickBloxUIKitDesktopLayout,
UseDefaultAITranslateWidget
} from 'quickblox-react-ui-kit';
function App() {
const currentUser: LoginData = {
userName: 'YOUR_REGISTRED_USER_NAME',
password: 'YOUR_REGISTRED_USER_PASSWORD',
};
const apiKey = 'sk-9aXs...ZFqhU'; // use your Api Key
const openAIConfiguration: Configuration = new Configuration({
apiKey,
});
const openAIApi: OpenAIApi = new OpenAIApi(openAIConfiguration);
defaultAITranslateWidget = UseDefaultAITranslateWidget({
...QBConfig.configAIApi.AITranslateWidgetConfig.proxyConfig,
sessionToken: token,
});
return (
<QuickBloxUIKitProvider
maxFileSize={100 * 1000000}
accountData={{ ...QBConfig.credentials }}
loginData={{
login: currentUser.login,
password: currentUser.password,
}} >
<div className="App">
<QuickBloxUIKitDesktopLayout
theme={new DefaultTheme()}
AITranslate={{
enabled: true,
default: false,
AIWidget: defaultAITranslateWidget,
}}
/>
</div>
</QuickBloxUIKitProvider>
);
}
export default App;
in MessageView component se detail information on this page :
<DesktopLayout
theme={theme}
dialogsView={
<DialogsComponent
// ...
/>
}
dialogMessagesView={
<MessagesView
subHeaderContent={<CompanyLogo />}
upHeaderContent={<CompanyLogo />}
dialogsViewModel={dialogsViewModel}
onDialogInformationHandler={informationOpenHandler}
theme={theme}
AITranslate={defaultAITranslateWidget}
/>
}
dialogInfoView={
<DialogInformation
// ...
/>
}
/>
You can compare the configuration of the QuickBloxUIKitDesktopLayout component with the AI Widget to the default view of the QuickBloxUIKitDesktopLayout component from the "Send your first message" page .
How to use the feature as a standalone library
You can use logic of the React hook features separately from our UI kit is possible by connecting the library as described below. The library qb-ai-translate contains QBAITranslate object wich provide all nececry features.
install standalone library
QBAITranslate can be installed using Node Package Manager. To include it in your JS based project, follow these command:
npm install qb-ai-translate
add to own project
To use QBAITranslate in your project, follow these steps:
- Import the QBAIAnswerAssistant module:
import { ChatMessage } from 'qb-ai-core';
import { AITranslateSettings, QBAITranslate } from 'qb-ai-translate';
- Create an array of
ChatMessage
objects representing the chat history:
let prompt = "YOUR_TEXT_TO_TRANSLATE";
let messages = [
{role: Role.other, content: "Hello, Bob!"},
{role: Role.me, content: "Hi, Jim!"},
{role: Role.me, content: prompt},
]
or
let prompt = "YOUR_TEXT_TO_TRANSLATE";
let messages = [
{role: "assistant", content: "Hello, Bob!"},
{role: "user", content: "Hi, Jim!"},
{role: "user", content: prompt},
]
- Call the
translate
method to generate translate using an API key:
const settings: AITranslateSettings =
QBAITranslate.createDefaultAITranslateSettings();
settings.apiKey = 'YOUR_OPEN_AI_API_KEY';
settings.organization = 'YOUR_ORGANIZATION';// might be empty
settings.model = AIModel.gpt__3_5__turbo;
settings.language = 'LANGUAGE'; // in BCP47 format
// https://developer.mozilla.org/en-US/docs/Web/API/Navigator/languages
// https://datatracker.ietf.org/doc/html/rfc5646
return QBAITranslate.translate(
promt,
messages,
settings,
);
Alternatively, you can use a QuickBlox user token and a proxy URL for more secure communication:
const settings: AITranslateSettings =
QBAITranslate.createDefaultAITranslateSettings();
settings.organization = 'YOUR_ORGANIZATION';// might be empty
settings.model = AIModel.gpt__3_5__turbo;
settings.token = 'YOUR_QUICKBLOX_USER_SESSION_TOKEN';
settings.language = 'LANGUAGE';
settings.serverPath = `https://your-proxy-server-url`;
return QBAITranslate.translate(
promt,
messages,
settings,
);
AI Rephrase
QuickBlox provides answer prephrase message functionality that helps users allows you to rephrase the response for an outgoing message in the chosen tone..
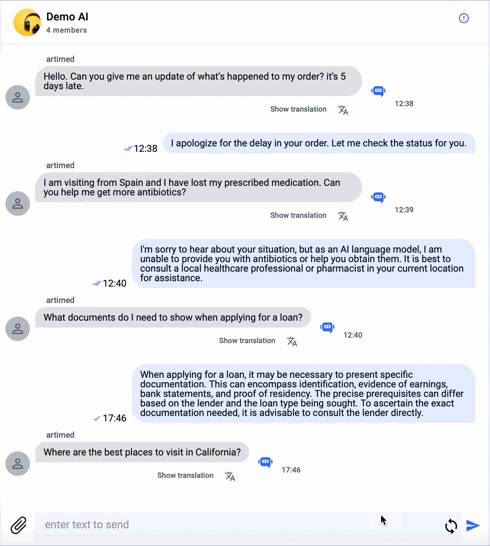
How to use in React UI Kit
Enabling QuickBlox AI Rephrase Message in React UI Kit involves just 2 straightforward actions:
- Set up the
AIRephraseWidgetConfig
in theconfigAIApi
segment of theQBConfig.ts
file to provide your API Key. - Optionally, initialize the
AIRephrase
property within theQuickBloxUIKitDesktopLayout
(orMessageView
) component for customizing the appearance or logic of the AI Rephrase component.
There are two ways to use the QuickBlox AI Rephrase:
- Direct: Access Open AI directly from your client code. The
AIRephrase
component use a raw Open AI token. This is suitable for development or demos, but it's not the recommended approach for production due to security considerations. - Proxy: Access Open AI through a proxy server. The
AIRephrase
component use QuickBlox user session token with a proxy server. This is the recommended method for production environments, ensuring a more secure and controlled setup.
Directly using configuration
To utilize the QuickBlox AI Rephrase Message React hook to communicate directly with the OpenAI API , you should configure the AIRephraseWidgetConfig section in the configuration file, as shown in the code snippet provided below. The token should be stored in the apiKey
property in the configAIApi
section in the QBConfig.ts
file. This contains information about configurations for all AI features . AFter that theAIRephrase
component will communicate directly with the OpenAI API.
export const QBConfig = {
credentials: {
//.. other section data
},
configAIApi: {
AIRephraseWidgetConfig: {
apiKey: 'sk-k2...LfK', // use your Api Key
useDefault: true,
defaultTone: 'Professional',
Tones: [
{
name: 'Professional Tone',
description:
'This would edit messages to sound more formal, using technical vocabulary, clear sentence structures, and maintaining a respectful tone. It would avoid colloquial language and ensure appropriate salutations and sign-offs',
iconEmoji: '👔',
},
{
name: 'Friendly Tone',
description:
'This would adjust messages to reflect a casual, friendly tone. It would incorporate casual language, use emoticons, exclamation points, and other informalities to make the message seem more friendly and approachable.',
iconEmoji: '🤝',
},
{
name: 'Encouraging Tone',
description:
'This tone would be useful for motivation and encouragement. It would include positive words, affirmations, and express support and belief in the recipient.',
iconEmoji: '💪',
},
{
name: 'Empathetic Tone',
description:
'This tone would be utilized to display understanding and empathy. It would involve softer language, acknowledging feelings, and demonstrating compassion and support.',
iconEmoji: '🤲',
},
{
name: 'Neutral Tone',
description:
'For times when you want to maintain an even, unbiased, and objective tone. It would avoid extreme language and emotive words, opting for clear, straightforward communication.',
iconEmoji: '😐',
},
{
name: 'Assertive Tone',
description:
'This tone is beneficial for making clear points, standing ground, or in negotiations. It uses direct language, is confident, and does not mince words.',
iconEmoji: '🔨',
},
{
name: 'Instructive Tone',
description:
'This tone would be useful for tutorials, guides, or other teaching and training materials. It is clear, concise, and walks the reader through steps or processes in a logical manner.',
iconEmoji: '📖',
},
{
name: 'Persuasive Tone',
description:
'This tone can be used when trying to convince someone or argue a point. It uses persuasive language, powerful words, and logical reasoning.',
iconEmoji: '☝️',
},
{
name: 'Sarcastic/Ironic Tone',
description:
'This tone can make the communication more humorous or show an ironic stance. It is harder to implement as it requires the AI to understand nuanced language and may not always be taken as intended by the reader.',
iconEmoji: '😏',
},
{
name: 'Poetic Tone',
description:
'This would add an artistic touch to messages, using figurative language, rhymes, and rhythm to create a more expressive text.',
iconEmoji: '🎭',
},
],
},
},
appConfig: {
//... other section data
},
};
Parameter name | Type | Description |
---|---|---|
apiKey | String | This field should hold your actual API key that you'll receive from the service provider (in this case, OpenAI). This key is used to authenticate your requests to the AI service. |
useDefault | Boolean | This setting seems to indicate whether you want to use default settings for the AI answer assistance widget. If set to true, it implies that the default settings will be applied. |
Tones | array of Tone | Array of objects describing the tones that users can use to rephrase text via the Rephrase component menu. Each tone should be defined as an object implementing the 'Tone' interface: interface Tone { name: string; description: string; iconEmoji: string; } |
Proxy using configuration
To utilize the QuickBlox AI Rephrase Message React hook to communicate through a proxy server (not directly) with OpenAI Api, you should configure the AIRephraseWidgetConfig section in the configuration file, as shown in the code snippet provided below. The fields api
, servername
, port
, and sessionToken
need to be configured within the proxyConfig section for communication with the proxy server.
export const QBConfig = {
credentials: {
//... other section data
},
configAIApi: {
AIRephraseWidgetConfig: {
apiKey: '', // must be empty
useDefault: true,
proxyConfig: {
api: 'v1/chat/completions',
servername: 'https://myproxy.com',
port: '',
sessionToken: '',
},
},
},
appConfig: {
//... other section data
},
};
Parameter name | Type | Description |
---|---|---|
api | String | The specific API endpoint for AI completions.In default, it's set to v1/chat/completions . You should provide your url. |
serverName | String | The base URL for API requests. In default, it's set to 'https://api.openai.com/'. You should provide your url. |
sessionToken | String | Your should provide the QuickBlox user session token by default if you are using our proxy server. |
port | String | Default should be empty. |
We recommend using our proxy server, which you can access through this link. Please ensure that you are using the latest release.
AI Rephrase customization
You have the ability to control AI Rephrase Message from code as follows: set it to enabled/disabled, customize the appearance of the component icon, and modify its operational logic. To enable or disable Answer Assist use the following code:
<QuickBloxUIKitDesktopLayout
theme={new CustomTheme()}
AIRephrase={{ enabled: true, default: true }}
/>
The QuickBloxUIKitDesktopLayout
is a layout component located within the provider section. We explored an example of its usage in our UI Kit documentation, specifically in the Send your First Message section.
To customize the appearance of the component icon, you should create your own React custom hook that must return an object of the AIMessageWidget interface and update the code within the textToWidget method.
For modifying its operational logic, you should create your own React custom hook that must return an object of the AIMessageWidget interface and update the code within the textToWidget method. The interface AIMessageWidget
has next structure:
Parameter name | Type | Description |
---|---|---|
renderWidget | Object function | The renderWidget provides the default appearance of the widget. This is a method that returns a JSX element (React component). It's responsible for rendering the widget, and depending on the presence of errors, it might display an error icon or a support icon. So you should change it to implement your own icon. |
textToWidget | Object function | The texToWidget provides the default logic of the widget. This is a function that takes a string (text) and context (in this case, an array of chat messages) but doesn't return anything (void). This function is used to pass text into the widget. So you should change it to implement your logic. |
textToContent | String | The textToContent provides the output of the default algorithm implementation. This is a string field representing content for the text widget. It's likely to be used to display the response obtained from AI in the text widget. |
Example code of custom hook:
interface MessageWidgetProps {
servername: string;
api: string;
port: string;
sessionToken: string;
}
export default function UseDefaultAIRephraseMessageWidget({
servername,
api,
port,
sessionToken,
}: MessageWidgetProps): AIMessageWidget {
const [errorMessage, setErrorMessage] = useState<string>('');
const [textFromWidgetToContent, setTextFromWidgetToContent] = useState('');
const renderWidget = (): JSX.Element => {
if (errorMessage && errorMessage.length > 0) {
const errorsDescriptions:
| { title: string; action: () => void }[]
| undefined = [];
return (
<ErrorMessageIcon
errorMessageText={errorMessage}
errorsDescriptions={errorsDescriptions}
/>
);
}
// you can customize your own Widget Icon markup
return <AIWidgetIcon applyZoom color="green" />;
};
const textToWidget = async (
textToSend: string,
context: IChatMessage[],
additionalSettings?: { [key: string]: any },
): Promise<string> => {
if (textToSend && textToSend.length > 0) {
let prompt = "Your prompt text with textToSend data";
const { yourAdditionalParam } = additionalSettings || {};
// your logic
// eslint-disable-next-line no-return-await
return await getData(prompt, context).then((data) => {
setTextFromWidgetToContent(data);
return data;
});
}
//
return '';
};
async function getData(
prompt: string,
dialogMessages: IChatMessage[],
): Promise<string> {
let outputMessage = '';
// your logic to use openai
return outputMessage;
}
return {
textToContent: textFromWidgetToContent,
renderWidget,
textToWidget,
};
}
To set up your own realization you should use the property AIRephrase
inQuickBloxUIKitDesktopLayout
or in MessageView
components like this:
Let's modify the QuickBloxUIKitDesktopLayout component from "Send your first message" page :
import React from 'react';
import * as QB from "quickblox/quickblox";
import {
LoginData,
QuickBloxUIKitProvider,
qbDataContext,
RemoteDataSource,
useQBConnection, QuickBloxUIKitDesktopLayout,
UseDefaultAIRephraseMessageWidget,
} from 'quickblox-react-ui-kit';
function App() {
const currentUser: LoginData = {
userName: 'YOUR_REGISTRED_USER_NAME',
password: 'YOUR_REGISTRED_USER_PASSWORD',
};
const apiKey = 'sk-9aXs...ZFqhU'; // use your Api Key
const openAIConfiguration: Configuration = new Configuration({
apiKey,
});
const openAIApi: OpenAIApi = new OpenAIApi(openAIConfiguration);
const defaultAIRephraseMessageWidget =
UseDefaultAIRephraseMessageWidget({
...QBConfig.configAIApi.AITranslateWidgetConfig.proxyConfig,
sessionToken: token,
});
return (
<QuickBloxUIKitProvider
maxFileSize={100 * 1000000}
accountData={{ ...QBConfig.credentials }}
loginData={{
login: currentUser.login,
password: currentUser.password,
}} >
<div className="App">
<QuickBloxUIKitDesktopLayout
theme={new DefaultTheme()}
AIRephrase={{
enabled: true,
default: false,
AIWidget: defaultAIRephraseMessageWidget,
}}
/>
</div>
</QuickBloxUIKitProvider>
);
}
export default App;
in MessageView component se detail information on this page :
<DesktopLayout
theme={theme}
dialogsView={
<DialogsComponent
// ...
/>
}
dialogMessagesView={
<MessagesView
subHeaderContent={<CompanyLogo />}
upHeaderContent={<CompanyLogo />}
dialogsViewModel={dialogsViewModel}
onDialogInformationHandler={informationOpenHandler}
theme={theme}
AIRephrase={defaultAIRephraseMessageWidget}
/>
}
dialogInfoView={
<DialogInformation
// ...
/>
}
/>
You can compare the configuration of the QuickBloxUIKitDesktopLayout component with the AI Widget to the default view of the QuickBloxUIKitDesktopLayout component from the "Send your first message" page .
How to use the feature as a standalone library
You can use logic of the React hook features separately from our UI kit is possible by connecting the library as described below. The library qb-ai-rephrase contains QBAIRephrase object wich provide all nececry features.
install standalone library
QBAIRephrase can be installed using Node Package Manager. To include it in your JS based project, follow these command:
npm install qb-ai-rephrase
add to own project
To use QBAIRephrase in your project, follow these steps:
- Import the QBAIRephrase module:
import { ChatMessage } from 'qb-ai-core';
import { AIRephraseSettings, QBAIRephrase } from 'qb-ai-rephrase';
- Create an array of
ChatMessage
objects representing the chat history:
let prompt = "YOUR_TEXT_TO_REPHRASE";
let messages = [
{role: Role.other, content: "Hello, Bob!"},
{role: Role.me, content: "Hi, Jim!"},
{role: Role.me, content: prompt},
]
or
let prompt = "YOUR_TEXT_TO_REPHRASE";
let messages = [
{role: "assistant", content: "Hello, Bob!"},
{role: "user", content: "Hi, Jim!"},
{role: "user", content: prompt},
]
- Call the
rephrase
method to generate translate using an API key:
const settings: AIRephraseSettings =
QBAIRephrase.createDefaultAIRephraseSettings();
settings.apiKey = 'YOUR_OPEN_AI_API_KEY';
settings.organization = 'YOUR_ORGANIZATION';// might be empty
settings.model = AIModel.gpt__3_5__turbo;
settings.tone = { name : 'YOUR_TONE_NAME',
description: 'YOUR_TONE_DESCRIPTION', // might be empty
iconEmoji: 'YOUR_EMOJI'}; // might be empty
return QBAIRephrase.rephrase(
promt,
messages,
settings,
);
Alternatively, you can use a QuickBlox user token and a proxy URL for more secure communication:
const settings: AIRephraseSettings =
QBAIRephrase.createDefaultAIRephraseSettings();
settings.organization = 'YOUR_ORGANIZATION';// might be empty
settings.model = AIModel.gpt__3_5__turbo;
settings.tone = { name : 'YOUR_TONE_NAME',
description: 'YOUR_TONE_DESCRIPTION', // might be empty
iconEmoji: 'YOUR_EMOJI'}; // might be empty
settings.token = 'YOUR_QUICKBLOX_USER_SESSION_TOKEN';
settings.serverPath = `https://your-proxy-server-url`;
return QBAIRephrase.rephrase(
promt,
messages,
settings,
);
Updated 7 months ago